
2532
Dependencies: QEI WS2812 PixelArray DFPlayerMini MODSERIAL PCA9685_ pca9685
DebounceIn.h
00001 /* 00002 Copyright (c) 2010 Andy Kirkham 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef DEBOUNCEIN_H 00024 #define DEBOUNCEIN_H 00025 00026 #include "mbed.h" 00027 00028 /** DebounceIn adds mechanical switch debouncing to DigitialIn. 00029 * 00030 * Example: 00031 * @code 00032 * #include "mbed.h" 00033 * #include "DebounceIn.h" 00034 * 00035 * DebounceIn d(p5); 00036 * DigitialOut led1(LED1); 00037 * DigitialOut led2(LED2); 00038 * 00039 * int main() { 00040 * while(1) { 00041 * led1 = d; 00042 * led2 = d.read(); 00043 * } 00044 * } 00045 * @endcode 00046 * 00047 * @see set_debounce_us() To change the sampling frequency. 00048 * @see set_samples() To alter the number of samples. 00049 * 00050 * Users of this library may also be interested in PinDetect library:- 00051 * @see http://mbed.org/users/AjK/libraries/PinDetect/latest 00052 * 00053 * This example shows one input displayed by two outputs. The input 00054 * is debounced by the default 10ms. 00055 */ 00056 00057 class DebounceIn : public DigitalIn { 00058 public: 00059 00060 /** set_debounce_us 00061 * 00062 * Sets the debounce sample period time in microseconds, default is 1000 (1ms) 00063 * 00064 * @param int i The debounce sample period time to set. 00065 */ 00066 void set_debounce_us(int i) 00067 { 00068 _ticker.attach_us(this, &DebounceIn::_callback, i); 00069 //ticker.attach(callback(this, &Sonar::background_read), 0.01f) 00070 } 00071 00072 /** set_samples 00073 * 00074 * Defines the number of samples before switching the shadow 00075 * definition of the pin. 00076 * 00077 * @param int i The number of samples. 00078 */ 00079 void set_samples(int i) { _samples = i; } 00080 00081 /** read 00082 * 00083 * Read the value of the debounced pin. 00084 */ 00085 int read(void) { return _shadow; } 00086 int trig(void) { _trig2 = _trig; _trig = 0; return _trig2; } 00087 int ntrig(void) { _ntrig2 = _ntrig; _ntrig = 0; return _ntrig2; } 00088 00089 #ifdef MBED_OPERATORS 00090 /** operator int() 00091 * 00092 * Read the value of the debounced pin. 00093 */ 00094 operator int() { return read(); } 00095 #endif 00096 00097 /** Constructor 00098 * 00099 * @param PinName pin The pin to assign as an input. 00100 */ 00101 DebounceIn(PinName pin) : DigitalIn(pin,PullUp) { _counter = 0; _samples = 5; set_debounce_us(8100); }; 00102 00103 protected: 00104 void _callback(void) { 00105 if (DigitalIn::read()) { 00106 if (_counter < _samples) _counter++; 00107 if (_counter == _samples) 00108 { 00109 _ntrig = 0; // comment if you don`t want to miss signal 00110 _nlock = 0; 00111 _shadow = 1; 00112 if (!_lock) {_trig = 1; _lock = 1;} 00113 } 00114 } 00115 else { 00116 if (_counter > 0) _counter--; 00117 if (_counter == 0) 00118 { 00119 _shadow = 0; 00120 _trig = 0; // comment if you don`t want to miss signal 00121 _lock = 0; 00122 if (!_nlock) {_ntrig = 1; _nlock = 1;} 00123 } 00124 } 00125 } 00126 00127 Ticker _ticker; 00128 int _lock; 00129 int _nlock; 00130 00131 int _ntrig; 00132 int _ntrig2; 00133 00134 int _trig; 00135 int _trig2; 00136 00137 int _shadow; 00138 int _counter; 00139 int _samples; 00140 }; 00141 00142 #endif 00143
Generated on Tue Jul 19 2022 11:38:13 by
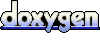