
clone
Dependencies: UniGraphic mbed-STM32F103C8T6
Fork of STM32F103C8T6_ILI9341 by
main.cpp
00001 #include "stm32f103c8t6.h" 00002 #include "mbed.h" 00003 #include "Arial12x12.h" 00004 #include "Arial24x23.h" 00005 #include "Arial43x48_numb.h" 00006 #include "pict.h" 00007 #include "pavement_48x34.h" 00008 #include "ILI9341.h" 00009 00010 const unsigned short FOREGROUND_COLORS[] = {White, Cyan, Red, Magenta, Yellow, Orange, GreenYellow}; 00011 const unsigned short BACKGROUND_COLORS[] = {Black, Navy, DarkGreen, DarkCyan, Maroon}; 00012 00013 Serial pc(PA_2, PA_3); // serial interface with PC 00014 ILI9341* tft; // ILI9341 driver 00015 Timer t; 00016 unsigned short backgroundColor; 00017 unsigned short foregroundColor; 00018 unsigned short colorIndex = 0; 00019 char orient = 3; 00020 00021 00022 00023 int main() 00024 { 00025 confSysClock(); //Configure system clock (72MHz HSE clock, 48MHz USB clock) 00026 00027 tft = new ILI9341(SPI_8, 20000000, PB_5, PB_4, PB_3, PA_15, PA_12, PA_11, "tft"); // SPI type, SPI speed, mosi, miso, sclk, cs, reset, dc 00028 tft->idle_on(); 00029 tft->set_orientation(orient); 00030 int time, time2; 00031 pc.baud (115200); 00032 pc.printf("\n\nSystem Core Clock = %.3f MHZ\r\n",(float)SystemCoreClock/1000000); 00033 t.start(); 00034 00035 while(1) { 00036 foregroundColor = FOREGROUND_COLORS[colorIndex % 7]; 00037 tft->foreground(foregroundColor); // set chars to white 00038 backgroundColor = BACKGROUND_COLORS[colorIndex % 5]; 00039 colorIndex++; 00040 tft->background(backgroundColor); // set background to black 00041 tft->set_orientation((orient++)%4); 00042 tft->cls(); // clear the screen 00043 tft->locate(0,30); 00044 tft->printf("Display ID: %.8X\r\n", tft->tftID); 00045 pc.printf("Display ID: %.8X\r\n", tft->tftID); 00046 // mem write/read test 00047 unsigned short readback; 00048 unsigned short colorstep = (0x10000/tft->width()); 00049 for(unsigned short i=0; i<tft->width(); i++) { 00050 tft->pixel(i,0,i*colorstep); // write line 00051 } 00052 bool readerror=false; 00053 for(unsigned short i=0; i<tft->width(); i++) { // verify line 00054 readback = tft->pixelread(i,0); 00055 if(readback!=i*colorstep) { 00056 readerror=true; 00057 pc.printf("pix %.4X readback %.4X\r\n", i*colorstep, readback); 00058 } 00059 } 00060 tft->locate(0,10); 00061 tft->printf("pixelread test %s\r\n", readerror ? "FAIL":"PASS"); 00062 wait(2); 00063 00064 tft->cls(); 00065 tft->set_font((unsigned char*) Terminal6x8,32,127,false); //variable width disabled 00066 tft->locate(0,0); 00067 tft->printf("Display Test\r\nSome text just to see if auto carriage return works correctly"); 00068 tft->set_font((unsigned char*) Terminal6x8); 00069 tft->printf("\r\nDisplay Test\r\nSome text just to see if auto carriage return works correctly"); 00070 pc.printf(" Display Test \r\n"); 00071 wait(3); 00072 t.reset(); 00073 tft->cls(); 00074 time=t.read_us(); 00075 tft->locate(2,55); 00076 tft->printf("cls: %.3fms", (float)time/1000); 00077 pc.printf("cls: %.3fms\r\n", (float)time/1000); 00078 wait(3); 00079 00080 tft->cls(); 00081 t.reset(); 00082 // draw some graphics 00083 tft->set_font((unsigned char*) Arial24x23); 00084 tft->locate(10,10); 00085 tft->printf("Test"); 00086 00087 tft->line(0,0,tft->width()-1,0,foregroundColor); 00088 tft->line(0,0,0,tft->height()-1,foregroundColor); 00089 tft->line(0,0,tft->width()-1,tft->height()-1,foregroundColor); 00090 00091 tft->rect(10,30,50,40,foregroundColor); 00092 tft->fillrect(60,30,100,40,foregroundColor); 00093 00094 tft->circle(150,32,30,foregroundColor); 00095 tft->fillcircle(140,20,10,foregroundColor); 00096 00097 double s; 00098 00099 for (unsigned short i=0; i<tft->width(); i++) { 00100 s =10 * sin((long double) i / 10 ); 00101 tft->pixel(i,40 + (int)s ,foregroundColor); 00102 } 00103 00104 00105 time=t.read_us(); 00106 tft->locate(2,55); 00107 tft->set_font((unsigned char*) Terminal6x8); 00108 tft->printf("plot: %.3fms", (float)time/1000); 00109 pc.printf("plot: %.3fms\r\n", (float)time/1000); 00110 wait(3); 00111 tft->cls(); 00112 t.reset(); 00113 Bitmap_s pic = { 00114 64, // XSize 00115 64, // YSize 00116 8, // Bytes in Line 00117 burp, // Pointer to picture data 00118 }; 00119 tft->Bitmap_BW(pic,tft->width()-64,0); 00120 time=t.read_us(); 00121 tft->locate(2,55); 00122 tft->printf("bmp: %.3fms", (float)time/1000); 00123 pc.printf("bmp: %.3fms\r\n", (float)time/1000); 00124 wait(3); 00125 tft->cls(); 00126 tft->set_font((unsigned char*) Arial43x48_numb, 46, 58, false); //only numbers, variable-width disabled 00127 t.reset(); 00128 tft->locate(0,0); 00129 tft->printf("%d", 12345); 00130 time=t.read_us(); 00131 tft->locate(2,55); 00132 tft->set_font((unsigned char*) Terminal6x8); 00133 tft->printf("Big Font: %.3fms", (float)time/1000); 00134 pc.printf("Big Font: %.3fms\r\n", (float)time/1000); 00135 wait(3); 00136 // sparse pixels test 00137 tft->cls(); 00138 tft->FastWindow(true); 00139 t.reset(); 00140 for(unsigned int i=0; i<20000; i++) { 00141 tft->pixel((i+(i*89)%tft->width()), (i+(i*61)%tft->height()), White); 00142 } 00143 tft->copy_to_lcd(); 00144 time=t.read_us(); 00145 tft->cls(); 00146 tft->FastWindow(false); 00147 t.reset(); 00148 for(unsigned int i=0; i<20000; i++) { 00149 tft->pixel((i+(i*89)%tft->width()), (i+(i*61)%tft->height()), White); 00150 } 00151 tft->copy_to_lcd(); 00152 time2=t.read_us(); 00153 tft->locate(2,55); 00154 tft->printf("std:%.3fms fastw:%.3fms", (float)time2/1000, (float)time/1000); 00155 pc.printf("std: %.3fms fastw: %.3fms\r\n", (float)time2/1000, (float)time/1000); 00156 wait(3); 00157 // scroll test, only for TFT 00158 tft->cls(); 00159 tft->set_font((unsigned char*) Arial24x23); 00160 tft->locate(2,10); 00161 tft->printf("Scrolling"); 00162 tft->rect(0,0,tft->width()-1,tft->height()-1,White); 00163 tft->rect(1,1,tft->width()-2,tft->height()-2,Blue); 00164 tft->setscrollarea(0,tft->sizeY()); 00165 wait(1); 00166 tft->scroll(1); //up 1 00167 wait(1); 00168 tft->scroll(0); //center 00169 wait(1); 00170 tft->scroll(tft->sizeY()-1); //down 1 00171 wait(1); 00172 tft->scroll(tft->sizeY()); // same as 0, center 00173 wait(1); 00174 tft->scroll(tft->sizeY()>>1); // half screen 00175 wait(1); 00176 tft->scrollreset(); // center 00177 wait(1); 00178 for(unsigned short i=1; i<=tft->sizeY(); i++) { 00179 tft->scroll(i); 00180 wait_ms(2); 00181 } 00182 wait(2); 00183 // color inversion 00184 for(unsigned short i=0; i<=8; i++) { 00185 tft->invert(i&1); 00186 wait_ms(200); 00187 } 00188 wait(2); 00189 // bmp 16bit test 00190 tft->cls(); 00191 t.reset(); 00192 for(int y=0; y<tft->height(); y+=34) { 00193 for(int x=0; x<tft->width(); x+=48) tft->Bitmap(x,y,48,34,(unsigned char *)pavement_48x34); 00194 } 00195 time=t.read_us(); 00196 tft->locate(2,55); 00197 tft->set_font((unsigned char*) Terminal6x8); 00198 tft->printf("Bmp speed: %.3fms", (float)time/1000); 00199 pc.printf("Bmp speed: %.3fms\r\n", (float)time/1000); 00200 wait(2); 00201 } 00202 } 00203 00204
Generated on Sat Jul 16 2022 16:46:05 by
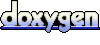