
library to drive dotstar strip
Fork of DotStar by
Embed:
(wiki syntax)
Show/hide line numbers
DotStar.h
00001 #include "rtos.h" 00002 00003 class DotStar { 00004 SPI* const spi_; 00005 const int ledNum_; 00006 int brightness_; 00007 int colors_[3]; 00008 public: 00009 enum { red = 0, green = 1, blue = 2 }; 00010 enum { redIndex = 2, greenIndex = 3, blueIndex = 4 }; 00011 enum {off = 0, dim = 8, half = 16, brightest = 31}; 00012 enum {pause = 100, sleep = 2000}; 00013 enum cmdType : char { 00014 setColor = '!', 00015 onOff = 'o', 00016 switchStrip = 's' 00017 }; 00018 void set_color(const int color, const int val); 00019 void set_leds(); 00020 DotStar(SPI* const spi, const int nLeds); 00021 void set_brightness(const int brightness); 00022 }; 00023 00024 struct RedGreenBlue { 00025 int red, green, blue; 00026 }; 00027 00028 class DotStarPair { 00029 DotStar* left; 00030 DotStar* right; 00031 public: 00032 DotStarPair(DotStar* l, DotStar* r); 00033 void set_brightness(const int brightness); 00034 void set_rgb(const RedGreenBlue& rgb); 00035 }; 00036 00037 DotStarPair::DotStarPair(DotStar* l, DotStar* r) : left(l), right(r) {} 00038 00039 void DotStarPair::set_brightness(const int brightness) { 00040 left->set_brightness(brightness); 00041 left->set_leds(); 00042 right->set_brightness(brightness); 00043 right->set_leds(); 00044 }; 00045 00046 void DotStarPair::set_rgb(const RedGreenBlue& rgb) { 00047 left->set_color(DotStar::blue, rgb.blue); 00048 left->set_color(DotStar::red, rgb.red); 00049 left->set_color(DotStar::green, rgb.green); 00050 left->set_leds(); 00051 right->set_color(DotStar::blue, rgb.blue); 00052 right->set_color(DotStar::red, rgb.red); 00053 right->set_color(DotStar::green, rgb.green); 00054 right->set_leds(); 00055 }; 00056 00057 DotStar::DotStar(SPI* const spi, const int nLeds) : spi_(spi), ledNum_(nLeds), 00058 brightness_(Init::brightness) { 00059 spi_->frequency(Init::frequency); 00060 colors_[DotStar::red] = Init::red; 00061 colors_[DotStar::blue] = Init::blue; 00062 colors_[DotStar::green] = Init::green; 00063 set_leds(); 00064 } 00065 00066 void DotStar::set_leds() { 00067 const int brightnessFrame = (7<<5)|brightness_; 00068 const int blueFrame = (colors_[DotStar::blue] ) & 0xff; 00069 const int greenFrame = (colors_[DotStar::green]) & 0xff; 00070 const int redFrame = colors_[DotStar::red] & 0xff; 00071 int i; 00072 Thread::wait(DotStar::pause); 00073 for (i = 4; i --> 0; spi_->write(0)) { } // start frame 00074 for (i = 0; i < ledNum_ ; ++i) { 00075 spi_->write(brightnessFrame); // led frame 00076 spi_->write(blueFrame); // B 00077 spi_->write(greenFrame); // G 00078 spi_->write(redFrame); // R 00079 } 00080 for (i = 4; i --> 0; spi_->write(1)) { } // end frame 00081 } 00082 00083 void DotStar::set_brightness(const int brightness) { 00084 brightness_ = brightness; 00085 } 00086 00087 void DotStar::set_color(const int c, const int val) { 00088 colors_[c] = val; 00089 };
Generated on Thu Jul 21 2022 10:42:39 by
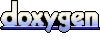