This is a port of Henning Kralsen's UTFT library for Arduino/chipKIT to mbed, refactored to make full use of C++ inheritance and access control, in order to reduce work when implementing new drivers and at the same time make the code more readable and easier to maintain. As of now supported are SSD1289 (16-bit interface), HX8340-B (serial interface) and ST7735 (serial interface). Drivers for other controllers will be added as time and resources to acquire the displays to test the code permit.
Dependents: test SDCard capstone_display capstone_display_2 ... more
lcd_base.h
00001 /** \file lcd_base.h 00002 * \brief Base class for all LCD controller implementations. 00003 * \copyright GNU Public License, v2. or later 00004 * 00005 * Generic object painting and screen control. 00006 * 00007 * This library is based on the Arduino/chipKIT UTFT library by Henning 00008 * Karlsen, http://henningkarlsen.com/electronics/library.php?id=52 00009 * 00010 * Copyright (C)2010-2012 Henning Karlsen. All right reserved. 00011 * 00012 * Copyright (C)2012 Todor Todorov. 00013 * 00014 * This library is free software; you can redistribute it and/or 00015 * modify it under the terms of the GNU Lesser General Public 00016 * License as published by the Free Software Foundation; either 00017 * version 2.1 of the License, or (at your option) any later version. 00018 * 00019 * This library is distributed in the hope that it will be useful, 00020 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00021 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00022 * Lesser General Public License for more details. 00023 * 00024 * You should have received a copy of the GNU Lesser General Public 00025 * License along with this library; if not, write to: 00026 * 00027 * Free Software Foundation, Inc. 00028 * 51 Franklin St, 5th Floor, Boston, MA 02110-1301, USA 00029 * 00030 *********************************************************************/ 00031 #ifndef TFTLCD_BASE_H 00032 #define TFTLCD_BASE_H 00033 00034 #include "mbed.h" 00035 #include "terminus.h" 00036 00037 #ifdef __cplusplus 00038 extern "C" { 00039 #endif 00040 00041 /** \def RGB(r,g,b) 00042 * \brief Creates a RGB color from distinct bytes for the red, green and blue components. 00043 * 00044 * Displays which use 16 bits to assign colors to a specific pixel, use 00045 * 5 bits for the red component, 6 bits for the green component and 5 00046 * bits for the blue component. Displays which have 18-bit color depth 00047 * use 6 bits for red, 6 bits for green and 6 bits for blue component. 00048 * This macro preserves the full 24-bit color depth, but it is the responsibility 00049 * of the respective driver to convert the color value to the correct format. 00050 */ 00051 #define RGB( r, g, b ) ( ( r ) << 16 ) | ( ( g ) << 8 ) | ( b ) 00052 /** \def COLOR_BLACK 00053 * \brief Shorthand for RGB( 0, 0, 0 ). 00054 */ 00055 #define COLOR_BLACK RGB( 0x00, 0x00, 0x00 ) 00056 /** \def COLOR_WHITE 00057 * \brief Shorthand for RGB( 255, 255, 255 ). 00058 */ 00059 #define COLOR_WHITE RGB( 0xFF, 0xFF, 0xFF ) 00060 /** \def COLOR_RED 00061 * \brief Shorthand for RGB( 255, 0, 0 ). 00062 */ 00063 #define COLOR_RED RGB( 0xFF, 0x00, 0x00 ) 00064 /** \def COLOR_GREEN 00065 * \brief Shorthand for RGB( 0, 255, 0 ). 00066 */ 00067 #define COLOR_GREEN RGB( 0x00, 0xFF, 0x00 ) 00068 /** \def COLOR_BLUE 00069 * \brief Shorthand for RGB( 0, 0, 255 ). 00070 */ 00071 #define COLOR_BLUE RGB( 0x00, 0x00, 0xFF ) 00072 /** \def COLOR_CYAN 00073 * \brief Shorthand for RGB( 0, 255, 255 ) 00074 */ 00075 #define COLOR_CYAN RGB( 0x00, 0xFF, 0xFF ) 00076 /** \def COLOR_MAGENTA 00077 * \brief Shorthand for RGB( 255, 0, 255 ) 00078 */ 00079 #define COLOR_MAGENTA RGB( 0xFF, 0x00, 0xFF ) 00080 /** \def COLOR_YELLOW 00081 * \brief Shorthand for RGB( 255, 255, 0 ) 00082 */ 00083 #define COLOR_YELLOW RGB( 0xFF, 0xFF, 0x00 ) 00084 00085 00086 /** \enum Orientation_enum 00087 * \brief Display orientation. 00088 */ 00089 enum Orientation_enum 00090 { 00091 PORTRAIT = 0, /**< Top row of the screen is at 12 o'clock. */ 00092 LANDSCAPE = 1, /**< Top row of the screen is at 9 o'clock. */ 00093 PORTRAIT_REV = 2, /**< Top row of the screen is at 6 o'clock. */ 00094 LANDSCAPE_REV = 3, /**< Top row of the screen is at 3 o'clock. */ 00095 }; 00096 /** \typedef orientation_t 00097 * \brief Convenience shortcut for display orientation. 00098 */ 00099 typedef enum Orientation_enum orientation_t; 00100 00101 /** \enum ColorDepth_enum 00102 * \brief Color depth 00103 */ 00104 enum ColorDepth_enum 00105 { 00106 RGB16, /**< 16-bit colors, pixels can have 65K+ distinct color values */ 00107 RGB18, /**< 18-bit colors, pixels can have 262K+ distinct color values */ 00108 RGB24, /**< 24-bit colors, full 8 bits per component, 16M+ distinct color values */ 00109 }; 00110 /** \typedef colordepth_t 00111 * \brief Convenience shortcut for display color depth. 00112 */ 00113 typedef enum ColorDepth_enum colordepth_t; 00114 00115 /** \enum Alignment_enum 00116 * \brief Horizontal text alignment on the line. 00117 */ 00118 enum Alignment_enum 00119 { 00120 LEFT = 0, /**< Left-oriented, naturally gravitate closer to the left edge of the screen. */ 00121 CENTER = 9998, /**< Center-oriented, try to fit in the middle of the available space with equal free space to the left and right of the text. */ 00122 RIGHT = 9999, /**< Right-oriented, naturally gravitate closer to the right edge of the screen, leaving any remaining free space to the left of the text. */ 00123 }; 00124 /** \typedef align_t 00125 * \brief Convenience shortcut for text alignment. 00126 */ 00127 typedef enum Alignment_enum align_t; 00128 00129 ///** \struct Font_struct 00130 // * \brief Describes fonts and their properties. 00131 // * \sa Comments in fonts.h 00132 // */ 00133 //struct Font_struct 00134 //{ 00135 // const char* font; /**< A pointer to the first byte in the font. */ 00136 // unsigned char width; /**< The width of each character, in pixels. */ 00137 // unsigned char height; /**< Height of each character, in pixels. */ 00138 // unsigned char offset; /**< Offset of the first character in the font. */ 00139 // unsigned char numchars; /**< Count of the available characters in the font. */ 00140 //}; 00141 ///** \typedef font_metrics_t 00142 // * \brief Convenience shortcut for fonts properties. 00143 // */ 00144 //typedef struct Font_struct font_metrics_t; 00145 00146 /** \struct Bitmap_struct 00147 * \brief Describes an image. 00148 */ 00149 struct Bitmap_struct 00150 { 00151 colordepth_t Format; /**< Color depth of the image. */ 00152 unsigned short Width; /**< Width of the image in pixels. */ 00153 unsigned short Height; /**< Height of the image in pixels. */ 00154 const void* PixelData; /**< Image pixel data. */ 00155 }; 00156 /** \typedef bitmap_t 00157 * \brief Convenience shortcut bitmap type. 00158 */ 00159 typedef struct Bitmap_struct bitmap_t; 00160 00161 /** \struct BacklightPwmCtrl_enum 00162 * \brief Type of backlight control for the LCD. 00163 * 00164 * When the selected type is \c Constant, the pin is simply on or off - there is no gradation in the intensity of the display. 00165 * In this case any free pin can be used to control the backlight. On the other hand, when PWM is used to control brightness, 00166 * take care to use only PWM-able mbed pins (p21, p22, p23, p24, p25, and p26), any other pins won't work. It is assumed that 00167 * you know what you are doing, so no check is done to prevent using a non-PWM pin as assigned control pin, when either \c Direct 00168 * or \c Indirect option is used. 00169 * 00170 * \version 0.1 00171 * \remark When choosing PWM to control the backlight, you have the option to choose the pin to either source (\c Direct) or sink 00172 * (\c Indirect) the current for LCD brightness control. Be aware that the mbed pins can source (and probably sink when 00173 * configured as inputs) only 4 mA @+3V3 VDD. So if you are intending to use a bigger LCD, whith more LEDs in its backlight 00174 * implementation, you probably want to interface it through a small signal transistor or a small MOSFET, in order to be able 00175 * to handle a higher current without damaging your mbed. 00176 * \remark As of version 0.1 (2013-01-25) the Indirect method of PWM has not been implemented yet. 00177 */ 00178 enum BacklightPwmCtrl_enum 00179 { 00180 Constant, /**< When the pin is a simple on/off switch. */ 00181 Direct, /**< Control the brightness with PWM, as the control pin is sourcing the current to drive the backlight LEDs. */ 00182 Indirect, /**< Control the brightness with PWM, as the control pin is sinking the current which drives the backlight LEDs. */ 00183 }; 00184 /** \typedef backlight_t 00185 * \brief Convenience shortcut for the backlight control type. 00186 */ 00187 typedef BacklightPwmCtrl_enum backlight_t; 00188 00189 00190 /** Base class for LCD implementations. 00191 * 00192 * All separate LCD controller implementations have to subclass this one. 00193 * 00194 * \version 0.1 00195 * \author Todor Todorov 00196 */ 00197 class LCD 00198 { 00199 public: 00200 00201 /** Initialize display. 00202 * 00203 * Wakes up the display from sleep, initializes power parameters. 00204 * This function must be called first, befor any painting on the 00205 * display is done, otherwise the positioning of graphical elements 00206 * will not work properly and any paynt operation will not be visible 00207 * or produce garbage. 00208 * 00209 * This function is controller-specific and needs to be implemented 00210 * separately for each available display. 00211 * \param oritentation The display orientation, landscape is default. 00212 * \param colors The correct color depth to use for the pixel data. 00213 */ 00214 virtual void Initialize( orientation_t orientation, colordepth_t colors ) = 0; 00215 00216 /** Puts the display to sleep. 00217 * 00218 * When the display is in sleep mode, its power consumption is 00219 * minimized. Before new pixel data can be written to the display 00220 * memory, the controller needs to be brought out of sleep mode. 00221 * \sa #WakeUp( void ); 00222 * \remarks The result of this operation might not be exactly as 00223 * expected. Putting the display to sleep will cause the 00224 * controller to switch to the standard color of the LCD, 00225 * so depending on whether the display is normally white, 00226 * or normally dark, the screen might or might not go 00227 * dark. Additional power saving can be achieved, if 00228 * the backlight of the used display is not hardwired on 00229 * the PCB and can be controlled via the BL pin. 00230 * \remarks This function is controller-specific and needs to be 00231 * implemented separately for each available display. 00232 */ 00233 virtual void Sleep( void ); 00234 00235 /** Wakes up the display from sleep mode. 00236 * 00237 * This function needs to be called before any other, when the 00238 * display has been put into sleep mode by a previois call to 00239 * #Sleep( void ). 00240 * \remarks This function is controller-specific and needs to be 00241 * implemented separately for each available display. 00242 */ 00243 virtual void WakeUp( void ); 00244 00245 /** Set the foreground color for painting. 00246 * 00247 * This is the default foreground color to be used in painting operations. 00248 * If a specific output function allows for a different color to be specified 00249 * in place, the new setting will be used for that single operation only and 00250 * will not change this value. 00251 * 00252 * \param color The color to be used (24-bit color depth). 00253 * \sa #RGB(r,g,b) 00254 */ 00255 virtual void SetForeground( unsigned int color = COLOR_WHITE ); 00256 00257 /** Set the background color for painting. 00258 * 00259 * This is the default color to be used for "empty" pixels while painting. 00260 * If a particular function allows for a different value to be specified 00261 * when the function is called, the new value will be used only for this 00262 * single call and will not change this setting. 00263 * 00264 * \param color The background color (24-bit color depth). 00265 * \sa #RGB(r,g,b) 00266 */ 00267 virtual void SetBackground( unsigned int color = COLOR_BLACK ); 00268 00269 /** Sets the font to be used for painting of text on the screen. 00270 * \param font A pointer to the font data. 00271 * \sa Comments in file fonts.h 00272 */ 00273 virtual void SetFont( const font_t* font ); 00274 00275 /** Gets the display width. 00276 * \return Display width in pixels. 00277 */ 00278 unsigned short GetWidth( void ); 00279 00280 /** Gets the display height. 00281 * \return Display height in pixels. 00282 */ 00283 unsigned short GetHeight( void ); 00284 00285 /** Gets the font width. 00286 * \return The current font width. 00287 */ 00288 uint8_t GetFontWidth( void ); 00289 00290 /** Gets the font height. 00291 * \return The current font height. 00292 */ 00293 uint8_t GetFontHeight( void ); 00294 00295 /** Fills the whole screen with a single color. 00296 * \param color The color to be used. The value must be in RGB-565 format. 00297 * \remarks The special values -1 and -2 signify the preset background and foreground colors, respectively. 00298 * The backround color is the default. 00299 */ 00300 virtual void FillScreen( int color = -1 ); 00301 00302 /** Sets the backlight intensity in percent as a float value in the range [0.0,1.0]. 00303 * \param level The backligh intensity in percent, where 0.0 is off and 1.0 is full brightness. 00304 */ 00305 virtual void SetBacklightLevel( float level ); 00306 00307 /** Clears the screen. 00308 * 00309 * This is the same as calling #FillScreen() or #FillScreen( -1 ) to use the background color. 00310 */ 00311 virtual void ClearScreen( void ); 00312 00313 /** Draws a pixel at the specified location. 00314 * 00315 * By default the function will use the preset foreground color, but the background 00316 * or a custom color could be used as well. 00317 * 00318 * \param x The horizontal offset of the pixel from the upper left corner of the screen. 00319 * \param y The vertical offset of the pixel from the upper left corner of the screen. 00320 * \param color The color to be used. Use a custom color, or -1 for background and -2 for foreground color. 00321 */ 00322 virtual void DrawPixel( unsigned short x, unsigned short y, int color = -2 ); 00323 00324 /** Draws a line. 00325 * 00326 * \param x1 Horizontal offset of the beginning point of the line. 00327 * \param y1 Vertical offset of the beginning point of the line. 00328 * \param x2 Horizontal offset of the end point of the line. 00329 * \param y2 Verical offset of the end point of the line. 00330 * \param color The color to use for painting, or -1 for background, or -2 for foreground. 00331 */ 00332 virtual void DrawLine( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color = -2 ); 00333 00334 /** Paints a rectangle. 00335 * 00336 * \param x1 The horizontal offset of the beginning point of one of the rectangle's diagonals. 00337 * \param y1 The vertical offset of the beginning point of one of the rectangle's diagonals. 00338 * \param x2 The horizontal offset of the end point of the same of the rectangle's diagonals. 00339 * \param y2 The vertical offset of the end point of the same of the rectangle's diagonals. 00340 * \param color The color to use for painting. -1 indicated background, -2 foreground, or custom color. 00341 */ 00342 virtual void DrawRect( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color = -2 ); 00343 00344 /** Paints a rectangle and fills it with the paint color. 00345 * 00346 * \param x1 The horizontal offset of the beginning point of one of the rectangle's diagonals. 00347 * \param y1 The vertical offset of the beginning point of one of the rectangle's diagonals. 00348 * \param x2 The horizontal offset of the end point of the same of the rectangle's diagonals. 00349 * \param y2 The vertical offset of the end point of the same of the rectangle's diagonals. 00350 * \param color The color to use for painting. -1 indicated background, -2 foreground, or custom color. 00351 */ 00352 virtual void DrawRoundRect( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color = -2 ); 00353 00354 /** Paints a rectangle with rounded corners. 00355 * 00356 * \param x1 The horizontal offset of the beginning point of one of the rectangle's diagonals. 00357 * \param y1 The vertical offset of the beginning point of one of the rectangle's diagonals. 00358 * \param x2 The horizontal offset of the end point of the same of the rectangle's diagonals. 00359 * \param y2 The vertical offset of the end point of the same of the rectangle's diagonals. 00360 * \param color The color to use for painting. -1 indicated background, -2 foreground, or custom color. 00361 */ 00362 virtual void FillRect( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color = -2 ); 00363 00364 /** Paints a rectangle with rounded corners and fills it with the paint color. 00365 * 00366 * \param x1 The horizontal offset of the beginning point of one of the rectangle's diagonals. 00367 * \param y1 The vertical offset of the beginning point of one of the rectangle's diagonals. 00368 * \param x2 The horizontal offset of the end point of the same of the rectangle's diagonals. 00369 * \param y2 The vertical offset of the end point of the same of the rectangle's diagonals. 00370 * \param color The color to use for painting. -1 indicated background, -2 foreground, or custom color. 00371 */ 00372 virtual void FillRoundRect( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color = -2 ); 00373 00374 /** Paints a circle. 00375 * 00376 * \param x The offset of the circle's center from the left edge of the screen. 00377 * \param y The offset of the circle's center from the top edge of the screen. 00378 * \param radius The circle's radius. 00379 * \param color The color to use for painting. -1 indicated background, -2 foreground, or custom color. 00380 */ 00381 virtual void DrawCircle( unsigned short x, unsigned short y, unsigned short radius, int color = -2 ); 00382 00383 /** Paints a circle and fills it with the paint color. 00384 * 00385 * \param x The offset of the circle's center from the left edge of the screen. 00386 * \param y The offset of the circle's center from the top edge of the screen. 00387 * \param radius The circle's radius. 00388 * \param color The color to use for painting. -1 indicated background, -2 foreground, or custom color. 00389 */ 00390 virtual void FillCircle( unsigned short x, unsigned short y, unsigned short radius, int color = -2 ); 00391 00392 /** Print a text on the screen. 00393 * 00394 * \param str The text. 00395 * \param x The horizontal offset form the left edge of the screen. The special values LEFT, CENTER, 00396 * or RIGHT can be used instead of pixel offset to indicate the text's horizontal alignment. 00397 * \param y The vertical offset of the text from the top of the screen. 00398 * \param fgColor The foreground to use for painting the text; -1 indicates background color, -2 the foreground setting, or custom color. 00399 * \param bgColor The color to use for painting the empty pixels; -1 indicates the background color, -2 the foreground setting, or custom color. 00400 * \param deg If different than 0, the text will be rotated at an angle this many degrees around its starting point. Default is not to ratate. 00401 */ 00402 virtual void Print( const char *str, unsigned short x, unsigned short y, int fgColor = -2, int bgColor = -1, unsigned short deg = 0 ); 00403 00404 /** Draw an image on the screen. 00405 * 00406 * The pixels of the picture must be in the RGB-565 format. The data can be provided 00407 * as an array in a source or a header file. To convert an image file to the appropriate 00408 * format, a special utility must be utilized. One such tool is provided by Henning Karlsen, 00409 * the author of the UTFT display liberary and can be downloaded for free from his web site: 00410 * http://henningkarlsen.com/electronics/library.php?id=52 00411 * 00412 * \param x Horizontal offset of the first pixel of the image. 00413 * \param y Vertical offset of the first pixel of the image. 00414 * \param img Image data pointer. 00415 * \param scale A value of 1 will produce an image with its original size, while a different value will scale the image. 00416 */ 00417 virtual void DrawBitmap( unsigned short x, unsigned short y, const bitmap_t* img, unsigned char scale = 1 ); 00418 00419 /** Draw an image on the screen. 00420 * 00421 * The pixels of the picture must be in the RGB-565 format. The data can be provided 00422 * as an array in a source or a header file. To convert an image file to the appropriate 00423 * format, a special utility must be utilized. One such tool is provided by Henning Karlsen, 00424 * the author of the UTFT display liberary and can be downloaded for free from his web site: 00425 * http://henningkarlsen.com/electronics/library.php?id=52 00426 * 00427 * \param x Horizontal offset of the first pixel of the image. 00428 * \param y Vertical offset of the first pixel of the image. 00429 * \param img Image data pointer. 00430 * \param deg Angle to rotate the image before painting on screen, in degrees. 00431 * \param rox 00432 * \param roy 00433 */ 00434 virtual void DrawBitmap( unsigned short x, unsigned short y, const bitmap_t* img, unsigned short deg, unsigned short rox, unsigned short roy ); 00435 00436 protected: 00437 /** Creates an instance of the class. 00438 * 00439 * \param width Width of the display in pixels. 00440 * \param height Height of the display in pixels. 00441 * \param CS Pin connected to the CS input of the display. 00442 * \param RS Pin connected to the RS input of the display. 00443 * \param RESET Pin connected to the RESET input of the display. 00444 * \param BL Pin connected to the circuit controlling the LCD's backlight. 00445 * \param blType The type of backlight to be used. 00446 * \param defaultBacklight The standard backlight intensity (if using PWM control), expressed in percent as float value from 0.0 to 1.0 00447 */ 00448 LCD( unsigned short width, unsigned short height ,PinName CS, PinName RS, PinName RESET, PinName BL, backlight_t blType, float defaultBacklight ); 00449 00450 /** Activates the display for command/data transfer. 00451 * 00452 * Usually achieved by pulling the CS pin of the display low. 00453 */ 00454 virtual void Activate( void ); 00455 00456 /** Deactivates the display after data has been transmitted. 00457 * 00458 * Usually achieved by pulling the CS pin of the display high. 00459 */ 00460 virtual void Deactivate( void ); 00461 00462 /** Sends a command to the display. 00463 * 00464 * \param cmd The display command. 00465 * \remarks Commands are controller-specific and this function needs to 00466 * be implemented separately for each available controller. 00467 */ 00468 virtual void WriteCmd( unsigned short cmd ) = 0; 00469 00470 /** Sends pixel data to the display. 00471 * 00472 * \param data The display data. 00473 * \remarks Sendin data is controller-specific and this function needs to 00474 * be implemented separately for each available controller. 00475 */ 00476 virtual void WriteData( unsigned short data ) = 0; 00477 00478 /** Sends both command and data to the display controller. 00479 * 00480 * This is a helper utility function which combines the 2 functions above 00481 * into one single convenience step. 00482 * 00483 * \param cmd The display command. 00484 * \param data The display pixel data. 00485 */ 00486 virtual void WriteCmdData( unsigned short cmd, unsigned short data ); 00487 00488 /** Assigns a chunk of the display memory to receive data. 00489 * 00490 * When data is sent to the display after this function completes, the opertion will 00491 * start from the begining of the assigned address (pixel position) and the pointer 00492 * will be automatically incremented so that the next data write operation will continue 00493 * with the next pixel from the memory block. If more data is written than available 00494 * pixels, at the end of the block the pointer will jump back to its beginning and 00495 * commence again, until the next address change command is sent to the display. 00496 * 00497 * \param x1 The X coordinate of the pixel at the beginning of the block. 00498 * \param y1 The Y coordinate of the pixel at the beginning of the block. 00499 * \param x2 The X coordinate of the pixel at the end of the block. 00500 * \param y2 The Y coordinate of the pixel at the end of the block. 00501 * \remarks Addressing commands are controller-specific and this function needs to be 00502 * implemented separately for each available controller. 00503 */ 00504 virtual void SetXY( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2 ) = 0; 00505 00506 /** Resets the memory address for the next display write operation to the screen origins (0,0). 00507 */ 00508 virtual void ClearXY( void ); 00509 00510 /** Sets the color of the pixel at the address pointer of the controller. 00511 * 00512 * This function is to be provided by each implementation separately in 00513 * order to account for different color depths used by the controller. 00514 * \param color The color of the pixel. 00515 * \param mode The depth (palette) of the color. 00516 */ 00517 virtual void SetPixelColor( unsigned int color, colordepth_t mode = RGB24 ) = 0; 00518 00519 /** Draws a horizontal line. 00520 * 00521 * This is a utility function to draw horizontal-only lines 00522 * for reduced code complexity and faster execution. 00523 * 00524 * \param x X coordinate of the starting point of the line. 00525 * \param y Y coordinate of the starting point of the line. 00526 * \param len Length of the line. 00527 * \param color The color to use to draw the line. By default the global foreground color is used ( -2 ), 00528 * -1 switches to the default background color, or any custom color can be used. 00529 */ 00530 virtual void DrawHLine( unsigned short x, unsigned short y, unsigned short len, int color = -2 ); 00531 00532 /** Draws a vertical line. 00533 * 00534 * This is a utility function to draw vertical-only lines 00535 * for reduced code complexity and faster execution. 00536 * 00537 * \param x X coordinate of the starting point of the line. 00538 * \param y Y coordinate of the starting point of the line. 00539 * \param len Height of the line. 00540 * \param color The color to use to draw the line. By default the global foreground color is used ( -2 ), 00541 * -1 switches to the default background color, or any custom color can be used. 00542 */ 00543 virtual void DrawVLine( unsigned short x, unsigned short y, unsigned short len, int color = -2 ); 00544 00545 /** Prints a character at the given position and using the given color. 00546 * 00547 * \param c The character. 00548 * \param x X coordinate of the character position. 00549 * \param y Y coordinate of the character position. 00550 * \param fgColor Foreground color for drawing. By default the global foreground color is used ( -2 ), 00551 * -1 switches to the default background color, or any custom color can be used. 00552 * \param bgColor Background color for drawing. By default the global background color is used ( -1 ), 00553 * -2 switches to the default foreground color, or any custom color can be used. 00554 */ 00555 virtual void PrintChar( char c, unsigned short x, unsigned short y, int fgColor = -2, int bgColor = -1 ); 00556 00557 /** Prints a character at the given position and using the given color and with the given rotation. 00558 * 00559 * \param c The character. 00560 * \param x X coordinate of the character position. 00561 * \param y Y coordinate of the character position. 00562 * \param pos Position of the character in the string from which it originates (used to rotate a whole string). 00563 * \param fgColor Foreground color for drawing. By default the global foreground color is used ( -2 ), 00564 * -1 switches to the default background color, or any custom color can be used. 00565 * \param bgColor Background color for drawing. By default the global background color is used ( -1 ), 00566 * -2 switches to the default foreground color, or any custom color can be used. 00567 * \param deg The angle at which to rotate. 00568 */ 00569 virtual void RotateChar( char c, unsigned short x, unsigned short y, int pos, int fgColor = -2, int bgColor = -1, unsigned short deg = 0 ); 00570 00571 protected: 00572 unsigned short _disp_width, _disp_height; 00573 DigitalOut _lcd_pin_cs, _lcd_pin_rs, _lcd_pin_reset; 00574 orientation_t _orientation; 00575 colordepth_t _colorDepth; 00576 unsigned int _foreground, _background; 00577 const font_t* _font; 00578 DigitalOut* _lcd_pin_bl; 00579 PwmOut* _bl_pwm; 00580 backlight_t _bl_type; 00581 float _bl_pwm_default, _bl_pwm_current; 00582 }; 00583 00584 #ifdef __cplusplus 00585 } 00586 #endif 00587 00588 #endif /* TFTLCD_BASE_H */
Generated on Thu Jul 14 2022 02:02:54 by
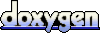