
This is a simple clock (hh:mm) make with the library Multi7Seg. The library and the demo software is make by 5OFT.
Dependencies: mbed Led7Seg Multi7Seg
main.cpp
00001 #include "mbed.h" 00002 #include "Multi7Seg.h" 00003 //##### A simple Clock ##### 00004 // Created by Michele Trombetta 00005 // Copyright 2010 5OFT. All rights reserved. 00006 00007 Ticker ticker_sec; 00008 Multi7Seg d_seconds(p21, p22, p23, p24, p25, p26, p27, p20, p19, led_ANODE); 00009 Multi7Seg d_minutes(p21, p22, p23, p24, p25, p26, p27, p18, p17, led_ANODE); 00010 DigitalOut seconds(p16); 00011 00012 unsigned int cnt_h = 0, cnt_m = 0, cnt_s = 0; 00013 00014 void inc_num() { 00015 cnt_s++; 00016 seconds = !seconds; 00017 if (cnt_s == 60) { 00018 cnt_s = 0; 00019 cnt_m++; 00020 } 00021 if (cnt_m == 60) { 00022 cnt_m = 0; 00023 cnt_h++; 00024 } 00025 if (cnt_h == 24) cnt_h = 0; 00026 } 00027 00028 int main() { 00029 00030 ticker_sec.attach(&inc_num, 1); 00031 seconds = 0; 00032 d_minutes.setformat(format_DEC); 00033 d_seconds.setformat(format_DEC); 00034 //d_seconds.setenabled(1); // Simple test to disable a single display 00035 while (1) { 00036 d_minutes.write(cnt_m); 00037 d_seconds.write(cnt_s); 00038 } 00039 }
Generated on Sun Jul 17 2022 07:50:05 by
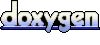