Matrix driver for HDSP-200x four character 5x7 alphanumeric displays. These are 12 pin DIL package ICs full of LEDs arranged in 4 characters of 5 columns & 7 rows each. The ones I have are the yellow HDSP-2001, but they are also available in red (HDSP-2000), high efficiency red (HDSP-2002) or green (HDSP 2003). I don't know if they are easily available anymore, but I thought, since I've got 30 or so, I'd have a go at programming them. See my notepad (http://mbed.org/users/tonymudd/notebook/led-matrix-display/) for videos of this working.
Dependents: alpha_message TP1_matriz
matrixDisplay.cpp
00001 #include "mbed.h" 00002 #include "matrixDisplay.h" 00003 #include "matrix.h" 00004 #include <string> 00005 00006 matrixDisplay::matrixDisplay( float scrollRate, PinName clockPinIn, 00007 PinName dataPinIn, PinName col1, 00008 PinName col2, PinName col3, 00009 PinName col4, PinName col5) 00010 { 00011 clockPin = new DigitalOut(clockPinIn); 00012 dataPin = new DigitalOut(dataPinIn); 00013 columnsBus = new BusOut(col1, col2, col3, col4, col5); 00014 column = 0; 00015 whereInMessage = 0; 00016 message = NULL; 00017 // clear the text array. 00018 for (int i =0 ; i < numOfChars; i++) 00019 text[i] = ' '; 00020 loadDisplay(); 00021 colTick.attach_us(this, &matrixDisplay::colChange, 5000); 00022 scroll.attach(this, &matrixDisplay::scrollMessage, scrollRate); 00023 } 00024 00025 matrixDisplay::~matrixDisplay() 00026 { 00027 stop(); 00028 } 00029 00030 // Stop displaying stuff. 00031 void matrixDisplay::stop() 00032 { 00033 colTick.detach(); 00034 scroll.detach(); 00035 columnsBus->write( 0); 00036 } 00037 00038 // load a new message 00039 // keeps a pointer to it, so bad things could happen. 00040 void matrixDisplay::setMessage(char * newMessage) 00041 { 00042 for (int i =0 ; i < numOfChars; i++) 00043 text[i] = ' '; 00044 message = newMessage; 00045 whereInMessage = 0; 00046 } 00047 00048 void matrixDisplay::outputCol(int colNum) // colNum is 0-4 00049 { 00050 for (int i = 0; i< numOfLeds; i++) // once for each bool in the "display" which has already been set up 00051 { 00052 clockPin->write(1); 00053 if (display[colNum][i]) 00054 dataPin->write(1); 00055 else 00056 dataPin->write(0); 00057 clockPin->write(0); 00058 } 00059 } 00060 00061 void matrixDisplay::colChange() 00062 { 00063 columnsBus->write( 0); 00064 column ++; 00065 if (column > 4) 00066 column = 0; 00067 outputCol(column); 00068 columnsBus->write (1 << column); // columns is a bit pattern 00069 } 00070 00071 // turns the contents of "text" into the bit pattern in "display" 00072 void matrixDisplay::loadDisplay() 00073 { 00074 for (int i =0;i < numOfChars; i ++) // for each char in text 00075 { 00076 char c = text[i]; 00077 c -= 32; // index an array starting at 0 for char ' ' (space) 00078 for (int led = 0; led < numOfRows; led++) // for each led in the column, done this way round because that's the way it's stored in matrix.h 00079 { 00080 int bits = char_data[c][led]; 00081 for (int col = 0; col < numOfColumns; col++) // for each column 00082 { 00083 display[col][led+i*numOfRows] = (bits & 1<< col); 00084 } 00085 } 00086 } 00087 } 00088 00089 void matrixDisplay::scrollMessage() 00090 { 00091 if (message == NULL) // It may not have been set yet. 00092 return; 00093 if (whereInMessage >= strlen(message)) 00094 whereInMessage =0; 00095 for (int i=1;i<numOfChars;i++) 00096 { 00097 text[i-1] = text[i]; 00098 } 00099 text[numOfChars-1] = message[whereInMessage]; 00100 loadDisplay(); 00101 whereInMessage ++; 00102 }
Generated on Wed Jul 13 2022 16:35:11 by
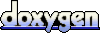