
can't push chnages :(
Fork of FBRDash by
Embed:
(wiki syntax)
Show/hide line numbers
Menu.h
00001 #ifndef FBRDASH_MENU_H 00002 #define FBRDASH_MENU_H 00003 00004 #include "TextLCD.h" 00005 #include "PinDetect.h" 00006 #include <string> 00007 00008 class MenuEntryBase 00009 { 00010 public: 00011 bool editable; 00012 00013 string label; 00014 string format; 00015 00016 virtual void increment() = 0; 00017 virtual void decrement() = 0; 00018 virtual string getFormatted() = 0; 00019 }; 00020 00021 template <typename T> 00022 class MenuEntry : public MenuEntryBase 00023 { 00024 public: 00025 T* value; 00026 T step; 00027 00028 virtual void increment() 00029 { 00030 *value += step; 00031 } 00032 00033 virtual void decrement() 00034 { 00035 *value -= step; 00036 } 00037 00038 virtual string getFormatted() 00039 { 00040 char buffer[16]; 00041 sprintf(buffer, format.c_str(), *value); 00042 return buffer; 00043 } 00044 }; 00045 00046 class Menu 00047 { 00048 public: 00049 bool display; 00050 bool edit; 00051 00052 Menu(TextLCD* _screen, PinName ok, PinName left, PinName right); 00053 00054 template <typename T> 00055 void addItem(string label, string format, T* value); 00056 00057 template <typename T> 00058 void addEditableItem(string label, string format, T* value, T step); 00059 00060 void refresh(); 00061 00062 void enter(); 00063 void done(); 00064 void left(); 00065 void right(); 00066 00067 private: 00068 MenuEntryBase* entries[20]; 00069 int entryCount; 00070 00071 PinDetect* btnOK; 00072 PinDetect* btnLeft; 00073 PinDetect* btnRight; 00074 00075 TextLCD* screen; 00076 00077 int position; 00078 bool ignoreNextEnter; 00079 00080 Timeout leftHeldTimeout; 00081 Timeout rightHeldTimeout; 00082 00083 void leftHeld(); 00084 void cancelLeftHeld(); 00085 void rightHeld(); 00086 void cancelRightHeld(); 00087 00088 static const float holdRepeatTime = 0.15; //should be multiple of screen refresh rate or wierdness will ensue 00089 static const char leftArrow = 0x7F; 00090 static const char rightArrow = 0x7E; 00091 }; 00092 00093 template <typename T> 00094 void Menu::addItem(string label, string format, T* value) 00095 { 00096 MenuEntry<T>* newEntry = new MenuEntry<T>(); 00097 00098 newEntry->value = value; 00099 newEntry->editable = false; 00100 00101 newEntry->label = label; 00102 newEntry->format = format; 00103 00104 entries[entryCount] = newEntry; 00105 entryCount++; 00106 } 00107 00108 template <typename T> 00109 void Menu::addEditableItem(string label, string format, T* value, T step) 00110 { 00111 MenuEntry<T> newEntry; 00112 00113 newEntry.value = value; 00114 newEntry.editable = true; 00115 newEntry.step = step; 00116 00117 newEntry.label = label; 00118 newEntry.format = format; 00119 00120 entries[entryCount] = newEntry; 00121 entryCount++; 00122 } 00123 00124 #endif
Generated on Wed Jul 13 2022 14:23:01 by
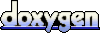