
can't push chnages :(
Fork of FBRDash by
Embed:
(wiki syntax)
Show/hide line numbers
Menu.cpp
00001 #include "Menu.h" 00002 #include "mbed.h" 00003 #include <string> 00004 00005 //Drive the menu system 00006 00007 //Initialise and attach button handlers 00008 Menu::Menu(TextLCD* _screen, PinName ok, PinName left, PinName right) 00009 { 00010 screen = _screen; 00011 00012 entryCount = 0; 00013 00014 position = 0; 00015 00016 btnOK = new PinDetect(ok, PullUp); 00017 btnLeft = new PinDetect(left, PullUp); 00018 btnRight = new PinDetect(right, PullUp); 00019 00020 btnOK->attach_deasserted(this, &Menu::enter); 00021 btnOK->attach_asserted_held(this, &Menu::done); 00022 00023 btnLeft->attach_asserted(this, &Menu::left); 00024 btnLeft->attach_asserted_held(this, &Menu::leftHeld); 00025 btnLeft->attach_deasserted(this, &Menu::cancelLeftHeld); 00026 00027 btnRight->attach_asserted(this, &Menu::right); 00028 btnRight->attach_asserted_held(this, &Menu::rightHeld); 00029 btnRight->attach_deasserted(this, &Menu::cancelRightHeld); 00030 00031 btnOK->setAssertValue(0); 00032 btnLeft->setAssertValue(0); 00033 btnRight->setAssertValue(0); 00034 00035 btnOK->setSampleFrequency(); 00036 btnLeft->setSampleFrequency(); 00037 btnRight->setSampleFrequency(); 00038 00039 ignoreNextEnter = false; 00040 } 00041 00042 void Menu::refresh() 00043 { 00044 //Create chars (if needed) for left and right arrows and editable indicator 00045 char labelLeft = (!edit & position > 0)?leftArrow:' '; 00046 char labelRight = (!edit & position < entryCount)?rightArrow:' '; 00047 char editIndic = (entries[position]->editable)?'*':' '; 00048 00049 screen->locate(0, 0); 00050 00051 //If position is on an entry, display it 00052 if(position <= entryCount - 1) 00053 { 00054 screen->printf("%c%-14s%c", labelLeft, (entries[position])->label.c_str(), labelRight); 00055 00056 char editLeft = (edit)?'-':editIndic; 00057 char editRight = (edit)?'+':' '; 00058 00059 screen->putc(editLeft); 00060 screen->printf(entries[position]->getFormatted().c_str()); 00061 screen->locate(15, 1); 00062 screen->putc(editRight); 00063 } 00064 //Otherwise show the return command 00065 else 00066 { 00067 screen->printf("%cReturn ", leftArrow); 00068 } 00069 } 00070 00071 //Handler for enter button 00072 void Menu::enter() 00073 { 00074 //Menu not on screen, display it 00075 if(!display && !ignoreNextEnter) 00076 { 00077 display = true; 00078 edit = false; 00079 } 00080 //Menu on screen 00081 else 00082 { 00083 //On an entry 00084 if(position <= entryCount - 1) 00085 { 00086 //If its editable, toggle edit status 00087 if(entries[position]->editable) 00088 edit = !edit; 00089 } 00090 //On return 00091 else 00092 { 00093 //Return, resetting position first 00094 position = 0; 00095 done(); 00096 } 00097 } 00098 00099 ignoreNextEnter = false; 00100 } 00101 00102 //Handler for enter held, clear the menu retaining position 00103 void Menu::done() 00104 { 00105 //Hide the menu 00106 display = false; 00107 //TODO: Can't remember why I needed this 00108 ignoreNextEnter = true; 00109 } 00110 00111 //Handler for left button 00112 void Menu::left() 00113 { 00114 //If not editing, scroll 00115 if(!edit && display && position > 0) 00116 position--; 00117 //If editing, edit 00118 else if(edit) 00119 entries[position]->decrement(); 00120 } 00121 00122 //Handler for left button held, start scrolling through menu. 00123 void Menu::leftHeld() 00124 { 00125 left(); 00126 leftHeldTimeout.attach(this, &Menu::leftHeld, holdRepeatTime); 00127 } 00128 00129 //Handler for left button release, stop scrolling 00130 void Menu::cancelLeftHeld() 00131 { 00132 leftHeldTimeout.detach(); 00133 } 00134 00135 //Same as left 00136 void Menu::right() 00137 { 00138 if(!edit && display && position < entryCount) 00139 position++; 00140 else if(edit) 00141 entries[position]->increment(); 00142 } 00143 00144 //Same as left 00145 void Menu::rightHeld() 00146 { 00147 right(); 00148 rightHeldTimeout.attach(this, &Menu::rightHeld, holdRepeatTime); 00149 } 00150 00151 //Same as left 00152 void Menu::cancelRightHeld() 00153 { 00154 rightHeldTimeout.detach(); 00155 }
Generated on Wed Jul 13 2022 14:23:01 by
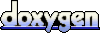