
kk
Dependencies: GPS TextLCD mbed
Fork of GPS_classroom by
main.cpp
00001 #include "mbed.h" 00002 #include "GPS.h" 00003 #include "TextLCD.h" 00004 #define BUFFER_SIZE 100 00005 00006 GPS gps(PA_11,PA_12); 00007 I2C i2c_lcd(D14,D15); 00008 TextLCD_I2C lcd(&i2c_lcd, 0x4E, TextLCD::LCD16x2); 00009 Serial esp(D8,D2); 00010 DigitalIn bt(D5,PullUp); 00011 Serial pc(USBTX, USBRX); 00012 char message[400]; 00013 00014 uint8_t buffer[0x100]; 00015 uint16_t buffer_head; 00016 uint16_t buffer_tail; 00017 char s1[100]; 00018 00019 00020 void rxcallback(void) { 00021 uint8_t chr; 00022 while (esp.readable()) { 00023 chr = esp.getc(); 00024 pc.putc(chr); 00025 buffer[buffer_head++] = chr; 00026 if (buffer_head == BUFFER_SIZE) 00027 { 00028 buffer_head = 0; 00029 } 00030 } 00031 } 00032 void flush_fifo(void) 00033 { 00034 while (esp.readable()) 00035 { 00036 (void)esp.getc(); 00037 } 00038 buffer_head = 0; 00039 buffer_tail = 0; 00040 } 00041 int find_response(const char *resp) { 00042 /* Give some delay for buffer to fill up */ 00043 wait_ms(10); 00044 int timeout = 0xFFFFFF; 00045 int len = strlen(resp); 00046 do 00047 { 00048 if (buffer_head > (buffer_tail + len)) 00049 { 00050 if (!memcmp(&buffer[buffer_tail], resp, len)) 00051 { 00052 flush_fifo(); 00053 return 0; 00054 } 00055 buffer_tail++; 00056 } 00057 }while(timeout--); 00058 flush_fifo(); 00059 return 1; 00060 } 00061 00062 int main() 00063 { 00064 lcd.setMode(TextLCD::DispOn); 00065 lcd.setBacklight(TextLCD::LightOff); 00066 lcd.setCursor(TextLCD::CurOff_BlkOff); 00067 esp.attach(&rxcallback, Serial::RxIrq); 00068 lcd.setAddress(0,0); 00069 lcd.printf("Initial GPS...\n"); 00070 esp.printf("AT+RST\r\n"); 00071 //lcd.printf("1\n"); 00072 MBED_ASSERT(find_response("OK") == 0); 00073 //lcd.printf("2\n"); 00074 esp.printf("AT+CWMODE=1\r\n"); 00075 //MBED_ASSERT(find_response("OK") == 0); 00076 //lcd.printf("3\n"); 00077 esp.printf("AT+CWJAP=\"%s\",\"%s\"\r\n","Fookies-One(m8)","12312344"); 00078 wait(5); 00079 //MBED_ASSERT(find_response("OK") == 0); 00080 //lcd.printf("4\n"); 00081 esp.printf("AT+CIPMUX=0\r\n"); 00082 //MBED_ASSERT(find_response("OK") == 0); 00083 //lcd.printf("5\n"); 00084 while(1){ 00085 if(gps.sample()) 00086 { 00087 lcd.cls(); 00088 lcd.printf("LAT:%6.3f\nLON:%7.3f",gps.latitude,gps.longitude); 00089 } 00090 wait(0.5); 00091 if(bt==0) 00092 { 00093 lcd.cls(); 00094 lcd.printf("Sending to twitter"); 00095 00096 sprintf(s1,"api_key=GRMKGK1CTUU7L5RD&status=LAT:%.3f,LON:%.3f",gps.latitude,gps.longitude); 00097 sprintf(message,"POST /apps/thingtweet/1/statuses/update HTTP/1.1\nHost: api.thingspeak.com\nConnection: close\nContent-Type: application/x-www-form-urlencoded\nContent-Length: %d\n\n%s",strlen(s1),s1); 00098 esp.printf("AT+CIPSTART=\"TCP\",\"%s\",%s\r\n","184.106.153.149","80"); 00099 //lcd.cls(); 00100 //lcd.printf("AT+CIPSTART=\"TCP\",\"%s\",%s\r\n","184.106.153.149","80"); 00101 wait(2); 00102 esp.printf("AT+CIPSEND=%d\r\n",strlen(message)); 00103 //lcd.cls(); 00104 //lcd.printf("AT+CIPSEND=%d\r\n",strlen(message)); 00105 wait(2); 00106 if(find_response(">") == 0) 00107 { 00108 //lcd.cls(); 00109 //lcd.printf(message); 00110 esp.printf(message); 00111 } 00112 wait(2); 00113 flush_fifo(); 00114 esp.printf("AT+CIPCLOSE\r\n"); 00115 wait(0.5); 00116 flush_fifo(); 00117 lcd.cls(); 00118 lcd.printf("Send Completed\n"); 00119 } 00120 } 00121 } 00122
Generated on Wed Jul 13 2022 02:58:47 by
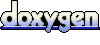