
Test of Embedded Artists LPCXpresso baseboard ethernet, SD card, audio and OLED display facilities. The program displays the day, date and time on the baseboard OLED and sounds the Big Ben chimes on the hour and quarter hour. On initial startup the program checks that the mbed clock is set and that the chime wav files can be accessed on the SD card. If not it asks to be connected to the internet to obtain the current time and to download the wav files to the SD card.
Dependencies: EthernetNetIf NTPClient_NetServices mbed EAOLED
main.cpp
00001 /* EA_BigBen 00002 00003 Just for fun this turns the Embedded Artists LPCXpresso baseboard into a Big Ben substitute. 00004 00005 Well OK it is a little smaller and the sound is a little tinnier than the real thing but it works. 00006 00007 This pulls together the following test programs for the Embedded Artists LPCXpresso baseboard: 00008 00009 EA_InternetClock (now superseded by this program) 00010 EA_WavPlayer 00011 EA_DownloadToSD 00012 00013 The program displays the day, date and time on the baseboard OLED and sounds the Big Ben chimes 00014 on the hour and quarter hour. 00015 00016 On initial startup the program checks that the mbed clock is set and that the wav files can be accessed 00017 on the SD card. If not it asks to be connected to the internet to obtain the current time and to download 00018 the wav files to the SD card. 00019 00020 The wav file player works with uncompressed wav files with 8 or 16 bit sample sizes. 00021 00022 Setup of the baseboard: 00023 00024 Please set all jumpers on board to the default case except for 00025 the following: 00026 00027 Audio setup: 00028 00029 1. Insert a jumper in J31 to connect signal PIO1_2 to the low 00030 pass filer as described in section 4.7.2. of base board users 00031 guide. 00032 00033 2. Insert three jumpers in J33 to connect PIO3_0, PIO3_1 and 00034 PIO3_2 to control the amplifier as described in section 4.8 00035 of base board users guide. 00036 00037 3. Insert a jumper in J32 and remove any from J34 to use the 00038 internal speaker as described in section 4.8 00039 of base board users guide. 00040 00041 SD Card setup: 00042 00043 4. Insert all five jumpers in J39 as described in section 4.3.3 00044 of base board users guide. 00045 00046 5. Remove jumper marked "A" in J55 In order to connect PIO1_11 00047 to CS signal of J40 (the SPI-SSEL signal) as described in section 4.3.3 00048 of base board users guide. 00049 00050 OLED setup: 00051 00052 1. The LCPXpresso board does not provide the data/command signal to the mbed. 00053 To provide this signal please connect a jumper wire from PIO2_7 of baseboard mbed socket 00054 (empty 2nd hole below mbed pin 21) to to PIO2_5 (on J6 right side ninth hole from bottom). 00055 00056 */ 00057 00058 #include "mbed.h" 00059 #include "wavplayer.h" 00060 #include "SDHCFileSystem.h" 00061 #include "EthernetNetIf.h" 00062 #include "NTPClient.h" 00063 #include "EAOLED.h" 00064 #include "HTTPClient.h" 00065 00066 int debug = 0; 00067 00068 EthernetNetIf eth; 00069 NTPClient ntp; 00070 HTTPClient http; 00071 00072 int ethernetUp = 0;//flag ethernet status 1 = up 0 = down 00073 00074 WavPlayer myWavPlayer; 00075 00076 EAOLED oled(p5, p21, p7, p8, p25); // mosi, dnc, sclk, cs, power 00077 00078 SDFileSystem sd(p5, p6, p7, p24, "sd");//mosi, miso, sclk, cs, name 00079 00080 DigitalOut led1(LED1); 00081 //DigitalOut led2(LED2); 00082 //DigitalOut led3(LED3); 00083 DigitalOut led4(LED4); 00084 00085 Ticker secTicker; 00086 Ticker blink; 00087 00088 int hour, minute, second, bongCount; 00089 00090 char day[12]; 00091 char dmy[12]; 00092 char hms[12]; 00093 00094 00095 void startUpMsgToOLED() { 00096 oled.cls(); 00097 oled.locate(0,1); 00098 oled.printf(" mbed clock\n"); 00099 oled.locate(0,3); 00100 oled.printf(" connect\n"); 00101 oled.locate(0,5); 00102 oled.printf(" ethernet\n"); 00103 oled.locate(0,7); 00104 oled.printf(" to start..\n"); 00105 } 00106 00107 //sets up ethernet connection only once 00108 void ethernetSetup() { 00109 if (!ethernetUp) { 00110 printf("Connecting to network ...\r\n"); 00111 EthernetErr ethErr = eth.setup(); 00112 if (ethErr) { 00113 printf("Error %d in setup.\r\n", ethErr); 00114 } 00115 printf("Network interface is up\r\n"); 00116 ethernetUp = 1; 00117 } 00118 } 00119 00120 //blinks led4 during download 00121 void blinkLED4() { 00122 led4 = !led4; 00123 } 00124 00125 void downloadFileToSD(char *url, char *path) { 00126 oled.cls(); 00127 oled.locate(0,1); 00128 oled.printf("Downloading\n"); 00129 oled.locate(0,3); 00130 oled.printf(" Wav files\n"); 00131 oled.locate(0,5); 00132 oled.printf("Please wait\n"); 00133 00134 HTTPFile httpfile(path); 00135 00136 printf("Downloading to "); 00137 printf("%s", path); 00138 printf(" please wait ... \r\n"); 00139 00140 blink.attach(& blinkLED4, 0.5); 00141 00142 HTTPResult result = http.get(url, &httpfile); 00143 00144 if (result == HTTP_OK) { 00145 printf("File downloaded OK\r\n"); 00146 } else { 00147 printf("Error during download %d\r\n", result); 00148 } 00149 00150 blink.detach(); 00151 00152 led4 = 0; 00153 } 00154 00155 void downloadFiles() { 00156 00157 ethernetSetup(); 00158 00159 printf("\r\n----------- Starting file download ------------\r\n"); 00160 00161 downloadFileToSD("http://homepage.ntlworld.com/green_bean/mbed/bong.wav", "/sd/bong.wav" ); 00162 downloadFileToSD("http://homepage.ntlworld.com/green_bean/mbed/quarter.wav", "/sd/quarter.wav" ); 00163 downloadFileToSD("http://homepage.ntlworld.com/green_bean/mbed/hour.wav", "/sd/hour.wav" ); 00164 00165 printf("-------------- Files downloaded ------------\r\n"); 00166 00167 } 00168 00169 void checkChimeWavFilesExist() { 00170 int filesExist = 1;//default exist 00171 FILE *fp1 = fopen("/sd/bong.wav", "rb"); 00172 if (fp1 == NULL) { 00173 filesExist = 0; 00174 } else fclose(fp1); 00175 FILE *fp2 = fopen("/sd/quarter.wav", "rb"); 00176 if (fp2 == NULL) { 00177 filesExist = 0; 00178 } else fclose(fp2); 00179 FILE *fp3 = fopen("/sd/hour.wav", "rb"); 00180 if (fp3 == NULL) { 00181 filesExist = 0; 00182 } else fclose(fp3); 00183 00184 if (!filesExist) downloadFiles(); 00185 } 00186 00187 void setmbedClock() { 00188 00189 startUpMsgToOLED(); 00190 printf("Setting mbed clock\r\n"); 00191 00192 ethernetSetup(); 00193 00194 printf("Connecting to time server...\r\n"); 00195 00196 Host server(IpAddr(), 123, "0.uk.pool.ntp.org"); 00197 ntp.setTime(server); 00198 00199 printf("Time updated\r\n"); 00200 oled.cls(); 00201 } 00202 00203 void updateTimeDisplay() { 00204 00205 time_t seconds; 00206 seconds = time(NULL); 00207 00208 struct tm *timeStruct = localtime(&seconds); 00209 00210 if (timeStruct->tm_isdst) {//daylight savings is on add one hour 00211 seconds+=3600; 00212 tm *timeStruct = localtime(&seconds); 00213 } 00214 00215 strftime(day,sizeof(day)," %A ", timeStruct);//localtime(&seconds)); 00216 strftime(dmy,sizeof(dmy),"%d/%m/%Y", timeStruct);//localtime(&seconds)); 00217 strftime(hms,sizeof(hms),"%H:%M:%S", timeStruct);//localtime(&seconds)); 00218 00219 //global hour, minute & second used in main() to set chimes 00220 hour = timeStruct->tm_hour; 00221 minute = timeStruct->tm_min; 00222 second = timeStruct->tm_sec; 00223 00224 if (debug == 1) printf("%s %s %s\r\n", hms,day,dmy); 00225 00226 oled.locate((12-strlen(day))/2,1); 00227 oled.printf("%s", day); 00228 oled.locate((12-strlen(hms))/2,4); 00229 oled.printf("%s", hms); 00230 oled.locate((12-strlen(dmy))/2,7); 00231 oled.printf("%s", dmy); 00232 00233 } 00234 00235 void checkIfChimesRequired() { 00236 00237 //check if time to sound hour 00238 if (minute == 59 && second == 34) { 00239 second ++; // ensure does not reenter this before updateTime 00240 secTicker.detach(); 00241 myWavPlayer.play_wave("/sd/hour.wav"); 00242 secTicker.attach(& updateTimeDisplay, 1); 00243 bongCount = (hour % 12) + 1; // No. bongs to count the hours 00244 //check if time to sound quarter hour 00245 } else if (minute % 15 == 0 && second == 0 ) { // will not strike on hour as caught above 00246 second ++; // ensure does not reenter this before updateTime 00247 secTicker.detach(); 00248 myWavPlayer.play_wave("/sd/quarter.wav"); 00249 secTicker.attach(& updateTimeDisplay, 1); 00250 //check if time for next hour bong 00251 } else if (bongCount > 0 && second % 5 == 0) { 00252 second ++; // ensure does not reenter this before updateTime 00253 secTicker.detach(); 00254 myWavPlayer.play_wave("/sd/bong.wav"); 00255 bongCount --; 00256 secTicker.attach(& updateTimeDisplay, 1); 00257 } 00258 } 00259 00260 int main() { 00261 00262 printf("\r\n------ Starting Big Ben -------\r\n"); 00263 00264 startUpMsgToOLED(); 00265 00266 //Check if chime wav files are on SD card if not download them 00267 checkChimeWavFilesExist(); 00268 00269 //Check if clock already set if < year 1990 assume needs setting 00270 time_t seconds = time(NULL); 00271 if (seconds < 630720000) { 00272 setmbedClock(); 00273 } 00274 oled.cls(); 00275 00276 00277 // starting ticker to update time display once per second 00278 secTicker.attach(& updateTimeDisplay, 1); 00279 00280 // On startup the quarter hour chime will play to ensure the SD card can be read 00281 // if you do not want this to happen remove the comment from the following line 00282 // second = 1; // so does not sound the quarter hour on first start 00283 00284 while (1) { 00285 00286 checkIfChimesRequired(); 00287 00288 wait(0.1); 00289 } 00290 00291 }
Generated on Mon Jul 25 2022 09:57:29 by
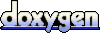