
Test of Embedded Artists LPCXpresso baseboard ethernet, SD card, audio and OLED display facilities. The program displays the day, date and time on the baseboard OLED and sounds the Big Ben chimes on the hour and quarter hour. On initial startup the program checks that the mbed clock is set and that the chime wav files can be accessed on the SD card. If not it asks to be connected to the internet to obtain the current time and to download the wav files to the SD card.
Dependencies: EthernetNetIf NTPClient_NetServices mbed EAOLED
SDHCFileSystem.h
00001 /* mbed SDFileSystem Library, for providing file access to SD cards 00002 * Copyright (c) 2008-2010, sford 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef MBED_SDHCFILESYSTEM_H 00024 #define MBED_SDHCFILESYSTEM_H 00025 00026 #include "mbed.h" 00027 #include "FATFileSystem.h" 00028 00029 /* Double Words */ 00030 typedef unsigned long long uint64_t; 00031 typedef long long sint64_t; 00032 00033 /** Access the filesystem on an SD Card using SPI 00034 * 00035 * @code 00036 * #include "mbed.h" 00037 * #include "SDFileSystem.h" 00038 * 00039 * SDFileSystem sd(p5, p6, p7, p12, "sd"); // mosi, miso, sclk, cs 00040 * 00041 * int main() { 00042 * FILE *fp = fopen("/sd/myfile.txt", "w"); 00043 * fprintf(fp, "Hello World!\n"); 00044 * fclose(fp); 00045 * } 00046 */ 00047 class SDFileSystem : public FATFileSystem { 00048 public: 00049 00050 /** Create the File System for accessing an SD Card using SPI 00051 * 00052 * @param mosi SPI mosi pin connected to SD Card 00053 * @param miso SPI miso pin conencted to SD Card 00054 * @param sclk SPI sclk pin connected to SD Card 00055 * @param cs DigitalOut pin used as SD Card chip select 00056 * @param name The name used to access the virtual filesystem 00057 */ 00058 SDFileSystem(PinName mosi, PinName miso, PinName sclk, PinName cs, const char* name); 00059 virtual int disk_initialize(); 00060 virtual int disk_write(const char *buffer, int block_number); 00061 virtual int disk_read(char *buffer, int block_number); 00062 virtual int disk_status(); 00063 virtual int disk_sync(); 00064 virtual int disk_sectors(); 00065 00066 protected: 00067 00068 int _cmd(int cmd, int arg); 00069 int _cmdx(int cmd, int arg); 00070 int _cmd8(); 00071 int _cmd58(); 00072 int initialise_card(); 00073 int initialise_card_v1(); 00074 int initialise_card_v2(); 00075 00076 int _read(char *buffer, int length); 00077 int _write(const char *buffer, int length); 00078 int _sd_sectors(); 00079 int _sectors; 00080 00081 SPI _spi; 00082 DigitalOut _cs; 00083 int cdv; 00084 }; 00085 00086 #endif
Generated on Mon Jul 25 2022 09:57:29 by
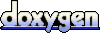