BlinkLed automatically. This library requires RTOS.
Dependents: mpod_nhk_english mpod_picasa_photoframe mpod_nhk_english_spxml
BlinkLed.cpp
00001 #include "BlinkLed.h" 00002 00003 BlinkLed::BlinkLed(PinName pin, float dutyChangeStep) : 00004 led(pin), 00005 dutyChangeStep(dutyChangeStep), 00006 pause(true), 00007 thread(0) 00008 { 00009 } 00010 00011 BlinkLed::~BlinkLed() 00012 { 00013 } 00014 00015 void BlinkLed::startBlink() 00016 { 00017 pause = false; 00018 if(!thread) 00019 { 00020 thread = new Thread(blink, this, osPriorityNormal, 128, NULL); 00021 } 00022 thread->signal_set(1); 00023 } 00024 00025 void BlinkLed::finishBlink() 00026 { 00027 pause = true; 00028 } 00029 00030 bool BlinkLed::isBlinking() 00031 { 00032 return !pause; 00033 } 00034 00035 void BlinkLed::blink(void const *argument) 00036 { 00037 BlinkLed* self = (BlinkLed*)argument; 00038 00039 bool sign = false; 00040 while(1) 00041 { 00042 if(self->pause) 00043 { 00044 self->led = 0.0F; 00045 Thread::signal_wait(1); 00046 } 00047 float brightness = self->led; 00048 sign = (brightness <= 0.0F) ? true : (1.0F <= brightness) ? false : sign; 00049 self->led = sign ? brightness + self->dutyChangeStep : brightness - self->dutyChangeStep; 00050 Thread::wait(20); 00051 } 00052 }
Generated on Wed Jul 20 2022 21:41:27 by
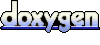