
Sample program to get ambient temperature from MCP9700 sensor
Embed:
(wiki syntax)
Show/hide line numbers
LinearTempSensor.h
00001 /* mbed Linear Temperature Sensor library 00002 * Supports Microchip MCP9700/9701, National Semiconductor LM35 00003 * 00004 * Written by Todotani, Nov 22 2010 00005 */ 00006 00007 #ifndef MBED_LINEARTEMPSENSOR_H 00008 #define MBED_LINEARTEMPSENSOR_H 00009 00010 #include "mbed.h" 00011 00012 /** Linear Temperature Sensor class. 00013 * Sample and store sensor acuired value in N (default=10) times and 00014 * calculate avarage temperature from sampled data 00015 * Supports Microchip MCP9700/9701, National Semiconductor LM35 00016 * @author Todotani 00017 */ 00018 class LinearTempSensor { 00019 public: 00020 /** Sensor Type Definitions */ 00021 enum SensorType { 00022 MCP9700, /**< Microchip MCP9700 (Default) */ 00023 MCP9701, /**< Microchip MCP9701 */ 00024 LM35 /**< National Semiconductor LM35 */ 00025 }; 00026 00027 /** Create a Temperature Sensor instanse 00028 * 00029 * @param ain PinName of analog input 00030 * @param N Number of samples to calculate average temperature (default = 10) 00031 * @param type Sensor type (default = MCP9700) 00032 */ 00033 LinearTempSensor(PinName ain, int N = 10, SensorType type = MCP9700); 00034 00035 /** Sample (read) sensor data and store to buffer 00036 * 00037 * @param None 00038 * @return Sensor-acuired value (mV) 00039 */ 00040 float Sense(); 00041 00042 /** Calculate average temperature from sample buffer 00043 * 00044 * @param None 00045 * @return Average temperature from N times of sumple (Centigrade) 00046 */ 00047 float GetAverageTemp(); 00048 00049 /** Calculate temperature from the latest sample 00050 * 00051 * @param None 00052 * @return Temperature from the latest sampled data (Centigrade) 00053 */ 00054 float GetLatestTemp(); 00055 00056 ~LinearTempSensor(); 00057 00058 private: 00059 AnalogIn _ain; 00060 int _samples; 00061 SensorType _type; 00062 00063 float *sampleBuffer; // Buffer to store sensor acuired data 00064 bool bufferNotFilled; // Flag shows that buffer have not filled 00065 uint32_t sampleCount; 00066 uint32_t index; 00067 00068 float V0; // Sensor read value in case 0 degree 00069 float Tc; // Tmperature coefficient (temprature inclease) in each degree 00070 float Vref; // Reference volgate for ADC 00071 }; 00072 00073 #endif
Generated on Fri Jul 15 2022 01:52:53 by
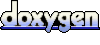