
Sample program to get ambient temperature from MCP9700 sensor
Embed:
(wiki syntax)
Show/hide line numbers
LinearTempSensor.cpp
00001 /* mbed Linear Temperature Sensor library 00002 * Supports Microchip MCP9700/9701, National Semiconductor LM35 00003 * 00004 * Written by Todotani, Nov 22 2010 00005 */ 00006 00007 #include "LinearTempSensor.h" 00008 00009 LinearTempSensor::LinearTempSensor(PinName ain, int N, SensorType type): 00010 // Initialize private variables using Initialization Lists 00011 // _ain(ain) is equivalent to _ain = new AnalogIn(ain) 00012 // _sample(N) is equivalent to _sample = N 00013 _ain(ain), _samples(N), _type(type) { 00014 sampleBuffer = (float *)malloc(sizeof(float) * _samples); 00015 sampleCount = 0; 00016 index = 0; 00017 Vref = 3300; 00018 bufferNotFilled = true; 00019 00020 // Set constants to calculate temperature from sensor value 00021 switch (_type) { 00022 case LM35: 00023 V0 = 0.0f; 00024 Tc = 10.0f; 00025 break; 00026 case MCP9701: 00027 V0 = 400.0f; 00028 Tc = 19.5f; 00029 break; 00030 case MCP9700: 00031 default: 00032 V0 = 500.0f; 00033 Tc = 10.0f; 00034 } 00035 00036 for (int i = 0; i < _samples; i++) 00037 sampleBuffer[i] = 0.0f; 00038 } 00039 00040 00041 LinearTempSensor::~LinearTempSensor() { 00042 free(sampleBuffer); 00043 } 00044 00045 00046 float LinearTempSensor::Sense() { 00047 float val; 00048 00049 val = _ain * Vref; 00050 sampleBuffer[index] = val; 00051 //printf("Index:%d ", index); // Debug print 00052 if ( ++index >= _samples ) 00053 index = 0; 00054 00055 if ( bufferNotFilled && (sampleCount == _samples) ) { 00056 bufferNotFilled = false; 00057 } 00058 //printf("flag:%d ", bufferNotFilled); // Debug print 00059 sampleCount++; 00060 00061 return val; 00062 } 00063 00064 00065 float LinearTempSensor::GetAverageTemp() { 00066 float sum = 0; 00067 int i, numberOfsumples; 00068 00069 if (sampleCount == 0) 00070 return 0; 00071 00072 if (bufferNotFilled) { 00073 // In case number of samples less than buffer lenght 00074 for (i = 0; i < sampleCount; i++) { 00075 sum += sampleBuffer[i]; 00076 } 00077 numberOfsumples = sampleCount; 00078 } else { 00079 // In case buffer is filled 00080 for (i = 0; i < _samples; i++) { 00081 sum += sampleBuffer[i]; 00082 } 00083 numberOfsumples = _samples; 00084 } 00085 00086 return ((sum / numberOfsumples) - V0) / Tc; // Temp = (Vout - V0) / Tc 00087 } 00088 00089 00090 float LinearTempSensor::GetLatestTemp() { 00091 return (sampleBuffer[(sampleCount-1)%_samples] - V0) / Tc; 00092 }
Generated on Fri Jul 15 2022 01:52:53 by
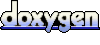