
Memory to Memory DMA demo from CMSIS example. This demo execute 1000 times of 32 word memory to memory DMA (copy), and also measures number of dummy loop execution during DMA cylcles. Line 56 of "DMA_M2M.c" can change DMA source. where; 1)static : source is SRAM 2)const : source is Flash ROM
lpc_types.h
00001 /***********************************************************************//** 00002 * @file lpc_types.h 00003 * @brief Contains the NXP ABL typedefs for C standard types. 00004 * It is intended to be used in ISO C conforming development 00005 * environments and checks for this insofar as it is possible 00006 * to do so. 00007 * @version 1.0 00008 * @date 27 Jul. 2008 00009 * @author wellsk 00010 ************************************************************************** 00011 * Software that is described herein is for illustrative purposes only 00012 * which provides customers with programming information regarding the 00013 * products. This software is supplied "AS IS" without any warranties. 00014 * NXP Semiconductors assumes no responsibility or liability for the 00015 * use of the software, conveys no license or title under any patent, 00016 * copyright, or mask work right to the product. NXP Semiconductors 00017 * reserves the right to make changes in the software without 00018 * notification. NXP Semiconductors also make no representation or 00019 * warranty that such application will be suitable for the specified 00020 * use without further testing or modification. 00021 **************************************************************************/ 00022 00023 /* Type group ----------------------------------------------------------- */ 00024 /** @defgroup LPC_Types LPC_Types 00025 * @ingroup LPC1700CMSIS_FwLib_Drivers 00026 * @{ 00027 */ 00028 00029 #ifndef LPC_TYPES_H 00030 #define LPC_TYPES_H 00031 00032 /* Includes ------------------------------------------------------------------- */ 00033 #include <stdint.h> 00034 00035 00036 /* Public Types --------------------------------------------------------------- */ 00037 /** @defgroup LPC_Types_Public_Types LPC_Types Public Types 00038 * @{ 00039 */ 00040 00041 /** 00042 * @brief Boolean Type definition 00043 */ 00044 typedef enum {FALSE = 0, TRUE = !FALSE} Bool; 00045 00046 /** 00047 * @brief Flag Status and Interrupt Flag Status type definition 00048 */ 00049 typedef enum {RESET = 0, SET = !RESET} FlagStatus, IntStatus, SetState; 00050 #define PARAM_SETSTATE(State) ((State==RESET) || (State==SET)) 00051 00052 /** 00053 * @brief Functional State Definition 00054 */ 00055 typedef enum {DISABLE = 0, ENABLE = !DISABLE} FunctionalState; 00056 #define PARAM_FUNCTIONALSTATE(State) ((State==DISABLE) || (State==ENABLE)) 00057 00058 /** 00059 * @ Status type definition 00060 */ 00061 typedef enum {ERROR = 0, SUCCESS = !ERROR} Status; 00062 00063 00064 /** 00065 * Read/Write transfer type mode (Block or non-block) 00066 */ 00067 typedef enum 00068 { 00069 NONE_BLOCKING = 0, /**< None Blocking type */ 00070 BLOCKING, /**< Blocking type */ 00071 } TRANSFER_BLOCK_Type; 00072 00073 00074 /** Pointer to Function returning Void (any number of parameters) */ 00075 typedef void (*PFV)(); 00076 00077 /** Pointer to Function returning int32_t (any number of parameters) */ 00078 typedef int32_t(*PFI)(); 00079 00080 /** 00081 * @} 00082 */ 00083 00084 00085 /* Public Macros -------------------------------------------------------------- */ 00086 /** @defgroup LPC_Types_Public_Macros LPC_Types Public Macros 00087 * @{ 00088 */ 00089 00090 /* _BIT(n) sets the bit at position "n" 00091 * _BIT(n) is intended to be used in "OR" and "AND" expressions: 00092 * e.g., "(_BIT(3) | _BIT(7))". 00093 */ 00094 #undef _BIT 00095 /* Set bit macro */ 00096 #define _BIT(n) (1<<n) 00097 00098 /* _SBF(f,v) sets the bit field starting at position "f" to value "v". 00099 * _SBF(f,v) is intended to be used in "OR" and "AND" expressions: 00100 * e.g., "((_SBF(5,7) | _SBF(12,0xF)) & 0xFFFF)" 00101 */ 00102 #undef _SBF 00103 /* Set bit field macro */ 00104 #define _SBF(f,v) (v<<f) 00105 00106 /* _BITMASK constructs a symbol with 'field_width' least significant 00107 * bits set. 00108 * e.g., _BITMASK(5) constructs '0x1F', _BITMASK(16) == 0xFFFF 00109 * The symbol is intended to be used to limit the bit field width 00110 * thusly: 00111 * <a_register> = (any_expression) & _BITMASK(x), where 0 < x <= 32. 00112 * If "any_expression" results in a value that is larger than can be 00113 * contained in 'x' bits, the bits above 'x - 1' are masked off. When 00114 * used with the _SBF example above, the example would be written: 00115 * a_reg = ((_SBF(5,7) | _SBF(12,0xF)) & _BITMASK(16)) 00116 * This ensures that the value written to a_reg is no wider than 00117 * 16 bits, and makes the code easier to read and understand. 00118 */ 00119 #undef _BITMASK 00120 /* Bitmask creation macro */ 00121 #define _BITMASK(field_width) ( _BIT(field_width) - 1) 00122 00123 /* NULL pointer */ 00124 #ifndef NULL 00125 #define NULL ((void*) 0) 00126 #endif 00127 00128 /* Number of elements in an array */ 00129 #define NELEMENTS(array) (sizeof (array) / sizeof (array[0])) 00130 00131 /* Static data/function define */ 00132 #define STATIC static 00133 /* External data/function define */ 00134 #define EXTERN extern 00135 00136 #define MAX(a, b) (((a) > (b)) ? (a) : (b)) 00137 #define MIN(a, b) (((a) < (b)) ? (a) : (b)) 00138 00139 /** 00140 * @} 00141 */ 00142 00143 00144 /* Old Type Definition compatibility ------------------------------------------ */ 00145 /** @addtogroup LPC_Types_Public_Types LPC_Types Public Types 00146 * @{ 00147 */ 00148 00149 /** SMA type for character type */ 00150 typedef char CHAR; 00151 00152 /** SMA type for 8 bit unsigned value */ 00153 typedef uint8_t UNS_8; 00154 00155 /** SMA type for 8 bit signed value */ 00156 typedef int8_t INT_8; 00157 00158 /** SMA type for 16 bit unsigned value */ 00159 typedef uint16_t UNS_16; 00160 00161 /** SMA type for 16 bit signed value */ 00162 typedef int16_t INT_16; 00163 00164 /** SMA type for 32 bit unsigned value */ 00165 typedef uint32_t UNS_32; 00166 00167 /** SMA type for 32 bit signed value */ 00168 typedef int32_t INT_32; 00169 00170 /** SMA type for 64 bit signed value */ 00171 typedef int64_t INT_64; 00172 00173 /** SMA type for 64 bit unsigned value */ 00174 typedef uint64_t UNS_64; 00175 00176 /** 32 bit boolean type */ 00177 typedef Bool BOOL_32; 00178 00179 /** 16 bit boolean type */ 00180 typedef Bool BOOL_16; 00181 00182 /** 8 bit boolean type */ 00183 typedef Bool BOOL_8; 00184 00185 /** 00186 * @} 00187 */ 00188 00189 00190 #endif /* LPC_TYPES_H */ 00191 00192 /** 00193 * @} 00194 */ 00195 00196 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 21:38:20 by
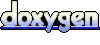