
Memory to Memory DMA demo from CMSIS example. This demo execute 1000 times of 32 word memory to memory DMA (copy), and also measures number of dummy loop execution during DMA cylcles. Line 56 of "DMA_M2M.c" can change DMA source. where; 1)static : source is SRAM 2)const : source is Flash ROM
DMA_M2M.c
00001 /***********************************************************************//** 00002 * @file flash_2_ram.c 00003 * @purpose This example used to test GPDMA function by transferring 00004 * data from flash to ram memory 00005 * @version 2.0 00006 * @date 21. May. 2010 00007 * @author NXP MCU SW Application Team 00008 * 00009 * Adupted for mebed 00010 * and modified for insturction execution test during DMA 00011 * by Todotani 14 Nov. 2010 00012 *--------------------------------------------------------------------- 00013 * Software that is described herein is for illustrative purposes only 00014 * which provides customers with programming information regarding the 00015 * products. This software is supplied "AS IS" without any warranties. 00016 * NXP Semiconductors assumes no responsibility or liability for the 00017 * use of the software, conveys no license or title under any patent, 00018 * copyright, or mask work right to the product. NXP Semiconductors 00019 * reserves the right to make changes in the software without 00020 * notification. NXP Semiconductors also make no representation or 00021 * warranty that such application will be suitable for the specified 00022 * use without further testing or modification. 00023 **********************************************************************/ 00024 00025 #include "lpc17xx_gpdma.h" 00026 #include "lpc17xx_libcfg.h" 00027 #include "mbed.h" 00028 #include "GetTickCount.h" 00029 00030 /* Example group ----------------------------------------------------------- */ 00031 /** @defgroup GPDMA_Flash_2_Ram_Test Flash_2_Ram_Test 00032 * @ingroup GPDMA_Examples 00033 * @{ 00034 */ 00035 00036 /************************** PRIVATE DEFINTIONS*************************/ 00037 #define DMA_SIZE 32 /** DMA transfer size */ 00038 #define DMA_CYCLES 1000 00039 00040 /************************** PRIVATE VARIABLES *************************/ 00041 uint8_t menu[]= 00042 "********************************************************************************\n\r" 00043 "Hello NXP Semiconductors \n\r" 00044 "GPDMA demo \n\r" 00045 "\t - MCU: LPC1768(mbed) \n\r" 00046 "\t - Core: ARM CORTEX-M3 \n\r" 00047 "\t - Communicate via: UART0 - 9600 bps \n\r" 00048 "This example used to test GPDMA function by transfer data from Flash \n\r" 00049 "to RAM memory\n\r" 00050 "********************************************************************************\n\r"; 00051 uint8_t menu2[] = "Demo terminated! \n\r"; 00052 uint8_t err_menu[] = "Buffer Check fail!\n\r"; 00053 uint8_t compl_menu[] = "Buffer Check success!\n\r\n\r"; 00054 00055 //DMAScr_Buffer will be burn into flash when compile 00056 static uint32_t DMASrc_Buffer[DMA_SIZE]= 00057 { 00058 0x01020304,0x05060708,0x090A0B0C,0x0D0E0F10, 00059 0x11121314,0x15161718,0x191A1B1C,0x1D1E1F20, 00060 0x21222324,0x25262728,0x292A2B2C,0x2D2E2F30, 00061 0x31323334,0x35363738,0x393A3B3C,0x3D3E3F40, 00062 0x01020304,0x05060708,0x090A0B0C,0x0D0E0F10, 00063 0x11121314,0x15161718,0x191A1B1C,0x1D1E1F20, 00064 0x21222324,0x25262728,0x292A2B2C,0x2D2E2F30, 00065 0x31323334,0x35363738,0x393A3B3C,0x3D3E3F40 00066 }; 00067 00068 uint32_t DMADest_Buffer[DMA_SIZE]; 00069 //uint32_t *DMADest_Buffer = (uint32_t *)0x2007C000; //LPC_AHBRAM0_BASE; 00070 00071 volatile uint32_t TC_count = 0; 00072 uint32_t loop = 0; 00073 GPDMA_Channel_CFG_Type GPDMACfg; 00074 00075 Serial pc(USBTX, USBRX); 00076 00077 00078 /*-------------------------MAIN FUNCTION------------------------------*/ 00079 extern "C" void DMA_IRQHandler (void); // extern "C" required for mbed 00080 00081 void Buffer_Init(void); 00082 void Buffer_Verify(void); 00083 void SetupDMA(void); 00084 00085 00086 /*----------------- INTERRUPT SERVICE ROUTINES --------------------------*/ 00087 /*********************************************************************//** 00088 * @brief GPDMA interrupt handler sub-routine 00089 * @param[in] None 00090 * @return None 00091 **********************************************************************/ 00092 void DMA_IRQHandler (void) 00093 { 00094 // check GPDMA interrupt on channel 0 00095 if (GPDMA_IntGetStatus(GPDMA_STAT_INT, 0)) { //check interrupt status on channel 0 00096 // Check counter terminal status 00097 if(GPDMA_IntGetStatus(GPDMA_STAT_INTTC, 0)) { 00098 // Clear terminate counter Interrupt pending 00099 GPDMA_ClearIntPending (GPDMA_STATCLR_INTTC, 0); 00100 00101 if (++TC_count < DMA_CYCLES) { 00102 // Setup channel with given parameter 00103 GPDMA_Setup(&GPDMACfg); 00104 // Run DMA again 00105 GPDMA_ChannelCmd(0, ENABLE); 00106 return; 00107 } else { 00108 /* DMA run predetermined cycles */ 00109 int elapsedTime = GetTickCount(); 00110 Buffer_Verify(); 00111 pc.printf("%s", compl_menu); 00112 pc.printf("DMA %d cycles, %d loop executions\n\r", TC_count, loop); 00113 pc.printf("Elapsed time %d ms\n\r\n\r", elapsedTime); 00114 00115 while(1); // Halt program 00116 } 00117 } 00118 00119 if (GPDMA_IntGetStatus(GPDMA_STAT_INTERR, 0)){ 00120 // Clear error counter Interrupt pending 00121 GPDMA_ClearIntPending (GPDMA_STATCLR_INTERR, 0); 00122 pc.printf("DMA Error detected.\n\r"); 00123 while(1); // Halt program 00124 } 00125 } 00126 } 00127 00128 00129 /*********************************************************************//** 00130 * @brief Verify buffer 00131 * @param[in] None 00132 * @return None 00133 **********************************************************************/ 00134 void Buffer_Verify(void) 00135 { 00136 uint8_t i; 00137 uint32_t *src_addr = (uint32_t *)DMASrc_Buffer; 00138 uint32_t *dest_addr = (uint32_t *)DMADest_Buffer; 00139 00140 for ( i = 0; i < DMA_SIZE; i++ ) 00141 { 00142 if ( *src_addr++ != *dest_addr++ ) 00143 { 00144 pc.printf("Verrify Error!.\n\r"); 00145 } 00146 } 00147 } 00148 00149 00150 /*-------------------------MAIN FUNCTION--------------------------------*/ 00151 /*********************************************************************//** 00152 * @brief c_entry: Main program body 00153 * @param[in] None 00154 * @return int 00155 **********************************************************************/ 00156 int c_entry(void) 00157 { 00158 pc.baud(9600); 00159 00160 // print welcome screen 00161 pc.printf("%s", menu); 00162 00163 /* Disable GPDMA interrupt */ 00164 NVIC_DisableIRQ(DMA_IRQn); 00165 /* preemption = 1, sub-priority = 1 */ 00166 NVIC_SetPriority(DMA_IRQn, ((0x01<<3)|0x01)); 00167 00168 /* Initialize GPDMA controller */ 00169 GPDMA_Init(); 00170 00171 // Setup GPDMA channel -------------------------------- 00172 // channel 0 00173 GPDMACfg.ChannelNum = 0; 00174 // Source memory 00175 GPDMACfg.SrcMemAddr = (uint32_t)DMASrc_Buffer; 00176 // Destination memory 00177 GPDMACfg.DstMemAddr = (uint32_t)DMADest_Buffer; 00178 // Transfer size 00179 GPDMACfg.TransferSize = DMA_SIZE; 00180 // Transfer width 00181 GPDMACfg.TransferWidth = GPDMA_WIDTH_WORD; 00182 // Transfer type 00183 GPDMACfg.TransferType = GPDMA_TRANSFERTYPE_M2M; 00184 // Source connection - unused 00185 GPDMACfg.SrcConn = 0; 00186 // Destination connection - unused 00187 GPDMACfg.DstConn = 0; 00188 // Linker List Item - unused 00189 GPDMACfg.DMALLI = 0; 00190 // Setup channel with given parameter 00191 GPDMA_Setup(&GPDMACfg); 00192 00193 /* Enable GPDMA interrupt */ 00194 NVIC_EnableIRQ(DMA_IRQn); 00195 00196 pc.printf("Start transfer...\n\r"); 00197 GetTickCount_Start(); 00198 00199 // Enable GPDMA channel 0 00200 GPDMA_ChannelCmd(0, ENABLE); 00201 00202 /* Wait for GPDMA processing complete */ 00203 while (1) { 00204 loop++; 00205 } 00206 00207 return 1; 00208 } 00209 00210 /* With ARM and GHS toolsets, the entry point is main() - this will 00211 allow the linker to generate wrapper code to setup stacks, allocate 00212 heap area, and initialize and copy code and data segments. For GNU 00213 toolsets, the entry point is through __start() in the crt0_gnu.asm 00214 file, and that startup code will setup stacks and data */ 00215 int main(void) { 00216 return c_entry(); 00217 } 00218 00219 #ifdef DEBUG 00220 /******************************************************************************* 00221 * @brief Reports the name of the source file and the source line number 00222 * where the CHECK_PARAM error has occurred. 00223 * @param[in] file Pointer to the source file name 00224 * @param[in] line assert_param error line source number 00225 * @return None 00226 *******************************************************************************/ 00227 void check_failed(uint8_t *file, uint32_t line) { 00228 /* User can add his own implementation to report the file name and line number, 00229 ex: printf("Wrong parameters value: file %s on line %d\r\n", file, line) */ 00230 00231 /* Infinite loop */ 00232 while (1) 00233 ; 00234 } 00235 #endif 00236 00237 /* 00238 * @} 00239 */
Generated on Tue Jul 12 2022 21:38:20 by
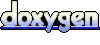