CyaSSL is an SSL library for devices like mbed.
Dependents: cyassl-client Sync
types.h
00001 /* types.h 00002 * 00003 * Copyright (C) 2006-2009 Sawtooth Consulting Ltd. 00004 * 00005 * This file is part of CyaSSL. 00006 * 00007 * CyaSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * CyaSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA 00020 */ 00021 00022 00023 #ifndef CTAO_CRYPT_TYPES_H 00024 #define CTAO_CRYPT_TYPES_H 00025 00026 #include "os_settings.h" 00027 00028 #ifdef HAVE_CONFIG_H 00029 #include "config.h" 00030 #endif 00031 00032 #ifdef __cplusplus 00033 extern "C" { 00034 #endif 00035 00036 00037 #if defined(WORDS_BIGENDIAN) || (defined(__MWERKS__) && !defined(__INTEL__)) 00038 #define BIG_ENDIAN_ORDER 00039 #endif 00040 00041 #ifndef BIG_ENDIAN_ORDER 00042 #define LITTLE_ENDIAN_ORDER 00043 #endif 00044 00045 #ifndef CYASSL_TYPES 00046 typedef unsigned char byte; 00047 typedef unsigned short word16; 00048 typedef unsigned int word32; 00049 #endif 00050 00051 #if defined(_MSC_VER) || defined(__BCPLUSPLUS__) 00052 #define WORD64_AVAILABLE 00053 #define W64LIT(x) x##ui64 00054 typedef unsigned __int64 word64; 00055 #elif SIZEOF_LONG == 8 00056 #define WORD64_AVAILABLE 00057 #define W64LIT(x) x##LL 00058 typedef unsigned long word64; 00059 #elif SIZEOF_LONG_LONG == 8 00060 #define WORD64_AVAILABLE 00061 #define W64LIT(x) x##LL 00062 typedef unsigned long long word64; 00063 #else 00064 #define MP_16BIT /* for mp_int, mp_word needs to be twice as big as 00065 mp_digit, no 64 bit type so make mp_digit 16 bit */ 00066 #endif 00067 00068 00069 /* These platforms have 64-bit CPU registers. */ 00070 #if (defined(__alpha__) || defined(__ia64__) || defined(_ARCH_PPC64) || \ 00071 defined(__mips64) || defined(__x86_64__)) 00072 typedef word64 word; 00073 #else 00074 typedef word32 word; 00075 #ifdef WORD64_AVAILABLE 00076 #define CTAOCRYPT_SLOW_WORD64 00077 #endif 00078 #endif 00079 00080 00081 enum { 00082 WORD_SIZE = sizeof(word), 00083 BIT_SIZE = 8, 00084 WORD_BITS = WORD_SIZE * BIT_SIZE 00085 }; 00086 00087 00088 /* use inlining if compiler allows */ 00089 #ifndef INLINE 00090 #ifndef NO_INLINE 00091 #ifdef _MSC_VER 00092 #define INLINE __inline 00093 #elif defined(__GNUC__) 00094 #define INLINE inline 00095 #elif defined(THREADX) 00096 #define INLINE _Inline 00097 #else 00098 #define INLINE 00099 #endif 00100 #else 00101 #define INLINE 00102 #endif 00103 #endif 00104 00105 00106 /* set up rotate style */ 00107 #if defined(_MSC_VER) || defined(__BCPLUSPLUS__) 00108 #define INTEL_INTRINSICS 00109 #define FAST_ROTATE 00110 #elif defined(__MWERKS__) && TARGET_CPU_PPC 00111 #define PPC_INTRINSICS 00112 #define FAST_ROTATE 00113 #elif defined(__GNUC__) && defined(__i386__) 00114 /* GCC does peephole optimizations which should result in using rotate 00115 instructions */ 00116 #define FAST_ROTATE 00117 #endif 00118 00119 00120 /* Micrium will use Visual Studio for compilation but not the Win32 API */ 00121 #if defined(_WIN32) && !defined(MICRIUM) 00122 #define USE_WINDOWS_API 00123 #endif 00124 00125 00126 /* idea to add global alloc override by Moisés Guimarães */ 00127 /* default to libc stuff */ 00128 /* XREALLOC is used once in mormal math lib, not in fast math lib */ 00129 /* XFREE on some embeded systems doesn't like free(0) so test */ 00130 #ifdef XMALLOC_USER 00131 /* prototypes for user heap override functions */ 00132 #include <stddef.h> /* for size_t */ 00133 extern void *XMALLOC(size_t n, void* heap, int type); 00134 extern void *XREALLOC(void *p, size_t n, void* heap, int type); 00135 extern void XFREE(void *p, void* heap, int type); 00136 #elif !defined(MICRIUM_MALLOC) 00137 /* defaults to C runtime if user doesn't override and not Micrium */ 00138 #include <stdlib.h> 00139 #define XMALLOC(s, h, t) malloc((s)) 00140 #define XFREE(p, h, t) {void* xp = (p); if((xp)) free((xp));} 00141 #define XREALLOC(p, n, h, t) realloc((p), (n)) 00142 #endif 00143 00144 #ifndef STRING_USER 00145 #include <string.h> 00146 #define XMEMCPY(d,s,l) memcpy((d),(s),(l)) 00147 #define XMEMSET(b,c,l) memset((b),(c),(l)) 00148 #define XMEMCMP(s1,s2,n) memcmp((s1),(s2),(n)) 00149 #define XMEMMOVE(d,s,l) memmove((d),(s),(l)) 00150 00151 #define XSTRLEN(s1) strlen((s1)) 00152 #define XSTRNCPY(s1,s2,n) strncpy((s1),(s2),(n)) 00153 /* strstr and strncmp only used by CyaSSL proper, not required for 00154 CTaoCrypt only */ 00155 #define XSTRSTR(s1,s2) strstr((s1),(s2)) 00156 #define XSTRNCMP(s1,s2,n) strncmp((s1),(s2),(n)) 00157 #endif 00158 00159 00160 /* memory allocation types for user hints */ 00161 enum { 00162 DYNAMIC_TYPE_CA = 1, 00163 DYNAMIC_TYPE_CERT = 2, 00164 DYNAMIC_TYPE_KEY = 3, 00165 DYNAMIC_TYPE_FILE = 4, 00166 DYNAMIC_TYPE_ISSUER_CN = 5, 00167 DYNAMIC_TYPE_PUBLIC_KEY = 6, 00168 DYNAMIC_TYPE_SIGNER = 7, 00169 DYNAMIC_TYPE_NONE = 8, 00170 DYNAMIC_TYPE_BIGINT = 9, 00171 DYNAMIC_TYPE_RSA = 10, 00172 DYNAMIC_TYPE_METHOD = 11, 00173 DYNAMIC_TYPE_OUT_BUFFER = 12, 00174 DYNAMIC_TYPE_IN_BUFFER = 13, 00175 DYNAMIC_TYPE_INFO = 14, 00176 DYNAMIC_TYPE_DH = 15, 00177 DYNAMIC_TYPE_DOMAIN = 16, 00178 DYNAMIC_TYPE_SSL = 17, 00179 DYNAMIC_TYPE_CTX = 18, 00180 DYNAMIC_TYPE_WRITEV = 19, 00181 DYNAMIC_TYPE_OPENSSL = 20 00182 }; 00183 00184 00185 #ifdef __cplusplus 00186 } /* extern "C" */ 00187 #endif 00188 00189 00190 #endif /* CTAO_CRYPT_TYPES_H */ 00191
Generated on Tue Jul 12 2022 18:43:19 by
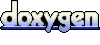