hello world
Fork of lmic_MOTE_L152RC by
Embed:
(wiki syntax)
Show/hide line numbers
debug.h
00001 /******************************************************************************* 00002 * Copyright (c) 2014-2015 IBM Corporation. 00003 * All rights reserved. This program and the accompanying materials 00004 * are made available under the terms of the Eclipse Public License v1.0 00005 * which accompanies this distribution, and is available at 00006 * http://www.eclipse.org/legal/epl-v10.html 00007 * 00008 * Contributors: 00009 * IBM Zurich Research Lab - initial API, implementation and documentation 00010 *******************************************************************************/ 00011 00012 #include <stdarg.h> 00013 #include <stdio.h> 00014 00015 /** Output a debug message 00016 * 00017 * @param format printf-style format string, followed by variables 00018 */ 00019 static inline void debug(const char *format, ...) { 00020 va_list args; 00021 va_start(args, format); 00022 vfprintf(stderr, format, args); 00023 va_end(args); 00024 } 00025 00026 #define NDEBUG 00027 #ifndef NDEBUG 00028 00029 /** Output a debug message 00030 * 00031 * @param format printf-style format string, followed by variables 00032 */ 00033 static inline void debugSW(const char *format, ...) { 00034 va_list args; 00035 va_start(args, format); 00036 vfprintf(stderr, format, args); 00037 va_end(args); 00038 } 00039 00040 #else 00041 static inline void debugSW(const char *format, ...) {} 00042 #endif 00043 00044 // intialize debug library 00045 void debug_init (void); 00046 00047 // set LED state 00048 void debug_led (u1_t val); 00049 00050 // write character to USART 00051 void debug_char (u1_t c); 00052 00053 // write byte as two hex digits to USART 00054 void debug_hex (u1_t b); 00055 00056 // write buffer as hex dump to USART 00057 void debug_buf (const u1_t* buf, u2_t len); 00058 00059 // write 32-bit integer as eight hex digits to USART 00060 void debug_uint (u4_t v); 00061 00062 // write nul-terminated string to USART 00063 void debug_str (const char* str); 00064 00065 // write LMiC event name to USART 00066 void debug_event (int ev); 00067 00068 // write label and 32-bit value as hex to USART 00069 void debug_val (const char* label, u4_t val); 00070 00071 void debug_done(void);
Generated on Mon Jul 18 2022 04:23:42 by
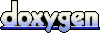