pin pong
Fork of SX1276Lib by
Embed:
(wiki syntax)
Show/hide line numbers
sx1272-hal.cpp
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C) 2014 Semtech 00008 00009 Description: - 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainers: Miguel Luis, Gregory Cristian and Nicolas Huguenin 00014 */ 00015 #include "sx1272-hal.h" 00016 #include "debug.h " 00017 00018 const RadioRegisters_t SX1272MB1xAS::RadioRegsInit[] = RADIO_INIT_REGISTERS_VALUE; 00019 00020 SX1272MB1xAS::SX1272MB1xAS( RadioEvents_t *events, 00021 PinName mosi, PinName miso, PinName sclk, PinName nss, PinName reset, 00022 PinName dio0, PinName dio1, PinName dio2, PinName dio3, PinName dio4, PinName dio5, 00023 PinName antSwitch ) 00024 : SX1272 ( events, mosi, miso, sclk, nss, reset, dio0, dio1, dio2, dio3, dio4, dio5 ), 00025 antSwitch( antSwitch ), 00026 #if( defined ( TARGET_NUCLEO_L152RE ) ) 00027 fake( D8 ) 00028 #else 00029 fake( A3 ) 00030 #endif 00031 { 00032 debug("SX1272MB1xAS constructor\r\n"); 00033 this->RadioEvents = events; 00034 00035 Reset( ); 00036 00037 RxChainCalibration( ); 00038 00039 IoInit( ); 00040 00041 SetOpMode( RF_OPMODE_SLEEP ); 00042 00043 IoIrqInit( dioIrq ); 00044 00045 RadioRegistersInit( ); 00046 00047 SetModem( MODEM_FSK ); 00048 00049 00050 this->settings.State = RF_IDLE ; 00051 } 00052 00053 SX1272MB1xAS::SX1272MB1xAS( RadioEvents_t *events ) 00054 #if defined ( TARGET_NUCLEO_L152RE ) 00055 : SX1272 ( events, D11, D12, D13, D10, A0, D2, D3, D4, D5, A3, D9 ), // For NUCLEO L152RE dio4 is on port A3 00056 antSwitch( A4 ), 00057 fake( D8 ) 00058 #elif defined( TARGET_LPC11U6X ) 00059 : SX1272 ( events, D11, D12, D13, D10, A0, D2, D3, D4, D5, D8, D9 ), 00060 antSwitch( P0_23 ), 00061 fake( A3 ) 00062 #else 00063 : SX1272 ( events, D11, D12, D13, D10, A0, D2, D3, D4, D5, D8, D9 ), 00064 antSwitch( PTC6 ), //changed from A4 to PTC6 by jlc 00065 fake( A3 ) 00066 #endif 00067 { 00068 debug("SX1272MB1xAS constructor1\r\n"); 00069 this->RadioEvents = events; 00070 00071 Reset( ); 00072 00073 boardConnected = UNKNOWN; 00074 00075 DetectBoardType( ); 00076 00077 RxChainCalibration( ); 00078 00079 IoInit( ); 00080 00081 SetOpMode( RF_OPMODE_SLEEP ); 00082 IoIrqInit( dioIrq ); 00083 00084 RadioRegistersInit( ); 00085 00086 00087 // SetModem( MODEM_LORA ); 00088 00089 this->settings.State = RF_IDLE ; 00090 } 00091 00092 //------------------------------------------------------------------------- 00093 // Board relative functions 00094 //------------------------------------------------------------------------- 00095 uint8_t SX1272MB1xAS::DetectBoardType( void ) 00096 { 00097 debug("DetectBoardType\r\n"); 00098 if( boardConnected == UNKNOWN ) 00099 { 00100 antSwitch .input( ); 00101 wait_ms( 1 ); 00102 if( antSwitch == 1 ) 00103 { 00104 boardConnected = SX1272RF1; 00105 } 00106 else 00107 { 00108 boardConnected = SX1272RF1; 00109 } 00110 antSwitch .output( ); 00111 wait_ms( 1 ); 00112 } 00113 return ( boardConnected ); 00114 } 00115 00116 void SX1272MB1xAS::IoInit( void ) 00117 { 00118 debug("IoInit\r\n"); 00119 AntSwInit( ); 00120 SpiInit( ); 00121 } 00122 00123 void SX1272MB1xAS::RadioRegistersInit( ) 00124 { 00125 uint8_t i = 0; 00126 for( i = 0; i < sizeof( RadioRegsInit ) / sizeof( RadioRegisters_t ); i++ ) 00127 { 00128 debug("RadioRegistersInit %d %02X %02X\r\n",RadioRegsInit[i].Modem ,RadioRegsInit[i].Addr, RadioRegsInit[i].Value ); 00129 SetModem( RadioRegsInit[i].Modem ); 00130 Write( RadioRegsInit[i].Addr, RadioRegsInit[i].Value ); 00131 } 00132 } 00133 00134 void SX1272MB1xAS::SpiInit( void ) 00135 { 00136 debug("SpiInit\r\n"); 00137 nss = 1; 00138 spi .format( 8,0 ); 00139 uint32_t frequencyToSet = 1000000; 00140 // uint32_t frequencyToSet = 8000000; 00141 // #if( defined ( TARGET_NUCLEO_L152RE ) || defined ( TARGET_LPC11U6X ) ) 00142 spi .frequency( frequencyToSet ); 00143 // #elif( defined ( TARGET_KL25Z ) ) //busclock frequency is halved -> double the spi frequency to compensate 00144 // spi.frequency( frequencyToSet * 2 ); 00145 // #else 00146 // #warning "Check the board's SPI frequency" 00147 // #endif 00148 wait(0.1); 00149 } 00150 00151 void SX1272MB1xAS::IoIrqInit( DioIrqHandler *irqHandlers ) 00152 { 00153 debug("IoIrqInit\r\n"); 00154 #if( defined ( TARGET_NUCLEO_L152RE ) || defined ( TARGET_LPC11U6X ) ) 00155 dio0 .mode(PullDown); 00156 dio1.mode(PullDown); 00157 dio2.mode(PullDown); 00158 dio3.mode(PullDown); 00159 dio4.mode(PullDown); 00160 #endif 00161 dio0 .rise( this, static_cast< TriggerMB1xAS > ( irqHandlers[0] ) ); 00162 dio1.rise( this, static_cast< TriggerMB1xAS > ( irqHandlers[1] ) ); 00163 dio2.rise( this, static_cast< TriggerMB1xAS > ( irqHandlers[2] ) ); 00164 dio3.rise( this, static_cast< TriggerMB1xAS > ( irqHandlers[3] ) ); 00165 dio4.rise( this, static_cast< TriggerMB1xAS > ( irqHandlers[4] ) ); 00166 } 00167 00168 void SX1272MB1xAS::IoDeInit( void ) 00169 { 00170 debug("IoDeInit\r\n"); 00171 //nothing 00172 } 00173 00174 uint8_t SX1272MB1xAS::GetPaSelect( uint32_t channel ) 00175 { 00176 debug("GetPaSelect\r\n"); 00177 if( channel > RF_MID_BAND_THRESH ) 00178 { 00179 if( boardConnected == SX1276MB1LAS ) 00180 { 00181 return RF_PACONFIG_PASELECT_PABOOST; 00182 } 00183 else 00184 { 00185 return RF_PACONFIG_PASELECT_RFO; 00186 } 00187 } 00188 else 00189 { 00190 return RF_PACONFIG_PASELECT_RFO; 00191 } 00192 } 00193 00194 void SX1272MB1xAS::SetAntSwLowPower( bool status ) 00195 { 00196 debug("SetAntSwLowPower %d\r\n",status); 00197 if( isRadioActive != status ) 00198 { 00199 isRadioActive = status; 00200 00201 if( status == false ) 00202 { 00203 AntSwInit( ); 00204 } 00205 else 00206 { 00207 AntSwDeInit( ); 00208 } 00209 } 00210 } 00211 00212 void SX1272MB1xAS::AntSwInit( void ) 00213 { 00214 debug("AntSwInit\r\n"); 00215 antSwitch = 0; 00216 } 00217 00218 void SX1272MB1xAS::AntSwDeInit( void ) 00219 { 00220 debug("AntSwDeInit\r\n"); 00221 antSwitch = 0; 00222 } 00223 00224 void SX1272MB1xAS::SetAntSw( uint8_t rxTx ) 00225 { 00226 debug("SetAntSw %d\r\n",rxTx); 00227 if( this->rxTx == rxTx ) 00228 { 00229 //no need to go further 00230 return; 00231 } 00232 00233 this->rxTx = rxTx ; 00234 00235 if( rxTx != 0 ) 00236 { 00237 antSwitch = 1; 00238 } 00239 else 00240 { 00241 antSwitch = 0; 00242 } 00243 } 00244 00245 bool SX1272MB1xAS::CheckRfFrequency( uint32_t frequency ) 00246 { 00247 debug("CheckRfFrequency\r\n"); 00248 //TODO: Implement check, currently all frequencies are supported 00249 return true; 00250 } 00251 00252 00253 void SX1272MB1xAS::Reset( void ) 00254 { 00255 debug("Reset\r\n"); 00256 reset .output(); 00257 reset = 0; 00258 wait_ms( 1 ); 00259 reset .input(); 00260 wait_ms( 6 ); 00261 } 00262 00263 void SX1272MB1xAS::Write( uint8_t addr, uint8_t data ) 00264 { 00265 debug("Write %02X %02X\r\n",addr, data); 00266 Write( addr, &data, 1 ); 00267 } 00268 00269 uint8_t SX1272MB1xAS::Read( uint8_t addr ) 00270 { 00271 uint8_t data; 00272 Read( addr, &data, 1 ); 00273 debug("Read %02X %02X\r\n",addr ,data); 00274 return data; 00275 } 00276 00277 void SX1272MB1xAS::Write( uint8_t addr, uint8_t *buffer, uint8_t size ) 00278 { 00279 uint8_t i; 00280 00281 nss = 0; 00282 spi .write( addr | 0x80 ); 00283 for( i = 0; i < size; i++ ) 00284 { 00285 spi .write( buffer[i] ); 00286 } 00287 nss = 1; 00288 } 00289 00290 void SX1272MB1xAS::Read( uint8_t addr, uint8_t *buffer, uint8_t size ) 00291 { 00292 uint8_t i; 00293 00294 nss = 0; 00295 spi .write( addr & 0x7F ); 00296 for( i = 0; i < size; i++ ) 00297 { 00298 buffer[i] = spi .write( 0 ); 00299 } 00300 nss = 1; 00301 } 00302 00303 void SX1272MB1xAS::WriteFifo( uint8_t *buffer, uint8_t size ) 00304 { 00305 debug("WriteFifo\r\n"); 00306 Write( 0, buffer, size ); 00307 } 00308 00309 void SX1272MB1xAS::ReadFifo( uint8_t *buffer, uint8_t size ) 00310 { 00311 debug("ReadFifo\r\n"); 00312 Read( 0, buffer, size ); 00313 }
Generated on Thu Jul 14 2022 02:13:10 by
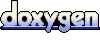