
It's nucleo f4 DMA trandfer for ov7670&ILI9341.
Dependencies: mbed ILI9341_SPI OV7670_SCCB
main.cpp
00001 #include "mbed.h" 00002 #include "TIM_DMAInit.h" 00003 #include "SPI_DMAInit.h" 00004 #include "ILI9341.h" 00005 #include "SCCB.h" 00006 #include "GPIOInit.h" 00007 /* 00008 00009 ********ov7670******** 00010 +3V3 - 3V3 GND-GND 00011 PC_12 - SIOC SIDO - PC10 00012 PD_2 - VSYNC HREF - A3 00013 B4,A4 - PCLK XCLK - PC_9 00014 PB_7 - D7 D6 - PB_6 00015 PB_5 - D5 D4 - PB_4 00016 PB_3 - D3 D2 - PB_2 00017 PB_1 - D1 D0 - PB_0 00018 +3V3-330Ω-RESET 00019 00020 ********74HC00********* 00021 A1 VCC - +3V3 00022 B1 B4 -- PCLK 00023 Y1 A4 -- PCLK 00024 A2 Y4 - B3 00025 B2 B3 - Y4 00026 Y2 A3 - HREF 00027 GND Y3 - PA8 00028 00029 ********ILI9341********* 00030 SDO - PA6 00031 LED - 10kΩ - +3V3 00032 SCK - PA5 00033 SDI - PA7 00034 DC - PA_4 00035 RS - PA_12 00036 CS - PA_11 00037 GND - GND 00038 VCC - +5V 00039 00040 00041 00042 */ 00043 00044 uint8_t dma_buf[145][300]; 00045 uint8_t flag_dma; 00046 00047 InterruptIn VSYNC(PD_2); 00048 SPI spi(PA_7,PA_6,PA_5); 00049 ili9341_spi lcd(spi,PA_11,PA_4,PA_12); 00050 ov7670_sccb sccb(PC_10,PC_12); 00051 PortIn cdata(PortB, 0x00ff); 00052 00053 void camdma_start(); 00054 00055 int main() { 00056 00057 00058 gpio_Init(); 00059 dma_init(); 00060 00061 spi.format(8,3); 00062 spi.frequency(20000000); 00063 lcd.tft_reset(); 00064 00065 __disable_irq(); 00066 00067 VSYNC.rise(&camdma_start); 00068 sccb.cam_init(); 00069 00070 lcd.fillrect(0,0,149,144,0xf800);//red 00071 00072 wait(1); 00073 lcd.wr_cmd(0x2C); 00074 spi.write(0x66); 00075 spi_Init(); 00076 00077 __enable_irq(); 00078 00079 00080 while(1) { 00081 } 00082 } 00083 00084 00085 void camdma_start() 00086 { 00087 if(flag_dma==0){ 00088 HAL_DMA_Start_IT(&DMA_HandleType/*DMA初期設定構造体*/, (uint32_t)&GPIOB->IDR/*&0x00ff転送元アドレス*/, (uint32_t)dma_buf/*転送先アドレス*/,43500/*データ転送回数*/); 00089 00090 HAL_SPI_Transmit_DMA(&spi1, (uint8_t*)dma_buf, sizeof(dma_buf)); 00091 } 00092 flag_dma=1; 00093 }
Generated on Fri Jul 15 2022 11:31:21 by
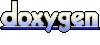