
Modbus RTU/ASCII/TCP with lwip TCP working partial, but with errors (retransmitions)
Dependencies: EthernetNetIf mbed
portother.cpp
00001 /* 00002 * FreeModbus Libary: lwIP Port 00003 * Copyright (C) 2006 Christian Walter <wolti@sil.at> 00004 * 00005 * This library is free software; you can redistribute it and/or 00006 * modify it under the terms of the GNU Lesser General Public 00007 * License as published by the Free Software Foundation; either 00008 * version 2.1 of the License, or (at your option) any later version. 00009 * 00010 * This library is distributed in the hope that it will be useful, 00011 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00012 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00013 * Lesser General Public License for more details. 00014 * 00015 * You should have received a copy of the GNU Lesser General Public 00016 * License along with this library; if not, write to the Free Software 00017 * Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00018 * 00019 * File: $Id: portother.c,v 1.1 2006/08/30 23:18:07 wolti Exp $ 00020 */ 00021 00022 /* ----------------------- System includes ----------------------------------*/ 00023 //#include <sys/types.h> 00024 //#include <sys/stat.h> 00025 //#include <unistd.h> 00026 #include <stdio.h> 00027 #include <stdarg.h> 00028 #include <string.h> 00029 00030 #include "port.h" 00031 00032 /* ----------------------- Defines ------------------------------------------*/ 00033 #define MB_FRAME_LOG_BUFSIZE 512 00034 00035 /* ----------------------- Start implementation -----------------------------*/ 00036 00037 #ifdef MB_TCP_DEBUG 00038 void 00039 prvvMBTCPLogFrame( const CHAR * pucMsg, UCHAR * pucFrame, USHORT usFrameLen ) 00040 { 00041 int i; 00042 int res; 00043 int iBufPos = 0; 00044 size_t iBufLeft = MB_FRAME_LOG_BUFSIZE; 00045 static CHAR arcBuffer[MB_FRAME_LOG_BUFSIZE]; 00046 00047 assert( pucFrame != NULL ); 00048 00049 for( i = 0; i < usFrameLen; i++ ) 00050 { 00051 /* Print some additional frame information. */ 00052 switch ( i ) 00053 { 00054 case 0: 00055 /* TID = Transaction Identifier. */ 00056 res = snprintf( &arcBuffer[iBufPos], iBufLeft, "| TID = " ); 00057 break; 00058 case 2: 00059 /* PID = Protocol Identifier. */ 00060 res = snprintf( &arcBuffer[iBufPos], iBufLeft, " | PID = " ); 00061 break; 00062 case 4: 00063 /* Length */ 00064 res = snprintf( &arcBuffer[iBufPos], iBufLeft, " | LEN = " ); 00065 break; 00066 case 6: 00067 /* UID = Unit Identifier. */ 00068 res = snprintf( &arcBuffer[iBufPos], iBufLeft, " | UID = " ); 00069 break; 00070 case 7: 00071 /* MB Function Code. */ 00072 res = snprintf( &arcBuffer[iBufPos], iBufLeft, "|| FUNC = " ); 00073 break; 00074 case 8: 00075 /* MB PDU rest. */ 00076 res = snprintf( &arcBuffer[iBufPos], iBufLeft, " | DATA = " ); 00077 break; 00078 default: 00079 res = 0; 00080 break; 00081 } 00082 if( res == -1 ) 00083 { 00084 break; 00085 } 00086 else 00087 { 00088 iBufPos += res; 00089 iBufLeft -= res; 00090 } 00091 00092 /* Print the data. */ 00093 res = snprintf( &arcBuffer[iBufPos], iBufLeft, "%02X", pucFrame[i] ); 00094 if( res == -1 ) 00095 { 00096 break; 00097 } 00098 else 00099 { 00100 iBufPos += res; 00101 iBufLeft -= res; 00102 } 00103 } 00104 00105 if( res != -1 ) 00106 { 00107 /* Append an end of frame string. */ 00108 res = snprintf( &arcBuffer[iBufPos], iBufLeft, " |\r\n" ); 00109 if( res != -1 ) 00110 { 00111 vMBPortLog( MB_LOG_DEBUG, pucMsg, "%s", arcBuffer ); 00112 } 00113 } 00114 } 00115 #endif 00116 00117 #ifdef MB_TCP_DEBUG 00118 void 00119 vMBPortLog( eMBPortLogLevel eLevel, const CHAR * szModule, const CHAR * szFmt, ... ) 00120 { 00121 va_list args; 00122 static const char *arszLevel2Str[] = { "ERROR", "WARN", "INFO", "DEBUG"}; 00123 00124 ( void )printf( "%s: %s: ", arszLevel2Str[eLevel], szModule ); 00125 va_start( args, szFmt ); 00126 vprintf( szFmt, args ); 00127 va_end( args ); 00128 } 00129 #endif
Generated on Tue Jul 12 2022 21:29:48 by
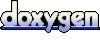