
Modbus RTU/ASCII/TCP with lwip TCP working partial, but with errors (retransmitions)
Dependencies: EthernetNetIf mbed
main.cpp
00001 /* 00002 * FreeModbus Libary: BARE Demo Application 00003 * Copyright (C) 2006 Christian Walter <wolti@sil.at> 00004 * 00005 * This program is free software; you can redistribute it and/or modify 00006 * it under the terms of the GNU General Public License as published by 00007 * the Free Software Foundation; either version 2 of the License, or 00008 * (at your option) any later version. 00009 * 00010 * This program is distributed in the hope that it will be useful, 00011 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00012 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00013 * GNU General Public License for more details. 00014 * 00015 * You should have received a copy of the GNU General Public License 00016 * along with this program; if not, write to the Free Software 00017 * Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00018 * 00019 * File: $Id: demo.c,v 1.1 2006/08/22 21:35:13 wolti Exp $ 00020 00021 * modified from: Thanassis Mavrogeorgiadis 5-12-2011 00022 */ 00023 00024 #include "mbed.h" 00025 00026 /* ----------------------- Modbus includes ----------------------------------*/ 00027 #include "mb.h" 00028 #include "mbport.h" 00029 #include "mbconfig.h" 00030 00031 00032 #if MB_TCP_ENABLED == 1 00033 #include "EthernetNetIf.h" 00034 EthernetNetIf eth; 00035 #endif 00036 00037 extern Serial pc; 00038 00039 DigitalOut led1(LED1); 00040 00041 DigitalIn ENET_LINK(P1_25); 00042 DigitalOut LedLINK(LED4); 00043 Ticker EtherPinMonitor; 00044 00045 #if MB_TCP_ENABLED == 1 00046 void EtherPinMonitorFunc(void) 00047 { 00048 LedLINK = !ENET_LINK; 00049 } 00050 #endif 00051 00052 /* ----------------------- Defines ------------------------------------------*/ 00053 #define REG_INPUT_START 1000 00054 #define REG_INPUT_NREGS 4 00055 #define REG_HOLDING_START 2000 00056 #define REG_HOLDING_NREGS 130 00057 00058 #define SLAVE_ID 0x0A 00059 00060 /* ----------------------- Static variables ---------------------------------*/ 00061 static USHORT usRegInputStart = REG_INPUT_START; 00062 static USHORT usRegInputBuf[REG_INPUT_NREGS]; 00063 static USHORT usRegHoldingStart = REG_HOLDING_START; 00064 static USHORT usRegHoldingBuf[REG_HOLDING_NREGS]; 00065 00066 /* ----------------------- Start implementation -----------------------------*/ 00067 00068 00069 int main() { 00070 eMBErrorCode eStatus; 00071 00072 #if MB_TCP_ENABLED == 1 00073 pc.baud(115200); 00074 EtherPinMonitor.attach(&EtherPinMonitorFunc, 0.01); 00075 printf("Setting up...\n"); 00076 EthernetErr ethErr = eth.setup(); 00077 if(ethErr) 00078 { 00079 printf("Error %d in setup.\n", ethErr); 00080 return -1; 00081 } 00082 printf("Setup OK\n"); 00083 #endif 00084 00085 Timer tm; 00086 tm.start(); 00087 00088 #if MB_RTU_ENABLED == 1 00089 eStatus = eMBInit( MB_RTU , SLAVE_ID, 0, 9600, MB_PAR_NONE ); 00090 #endif 00091 #if MB_ASCII_ENABLED == 1 00092 eStatus = eMBInit( MB_ASCII , SLAVE_ID, 0, 9600, MB_PAR_NONE ); 00093 #endif 00094 #if MB_TCP_ENABLED == 1 00095 eStatus = eMBTCPInit( MB_TCP_PORT_USE_DEFAULT ); 00096 #endif 00097 if (eStatus != MB_ENOERR ) 00098 printf( "can't initialize modbus stack!\r\n" ); 00099 00100 /* Enable the Modbus Protocol Stack. */ 00101 eStatus = eMBEnable( ); 00102 if (eStatus != MB_ENOERR ) 00103 fprintf( stderr, "can't enable modbus stack!\r\n" ); 00104 00105 // Initialise some registers 00106 usRegInputBuf[1] = 0x1234; 00107 usRegInputBuf[2] = 0x5678; 00108 usRegInputBuf[3] = 0x9abc; 00109 00110 while(true) 00111 { 00112 #if MB_TCP_ENABLED == 1 00113 Net::poll(); 00114 #endif 00115 if(tm.read()>.5) 00116 { 00117 led1=!led1; //Show that we are alive 00118 tm.start(); 00119 } 00120 00121 eStatus = eMBPoll( ); 00122 00123 /* Here we simply count the number of poll cycles. */ 00124 usRegInputBuf[0]++; 00125 } 00126 return 0; 00127 } 00128 00129 eMBErrorCode 00130 eMBRegInputCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNRegs ) 00131 { 00132 eMBErrorCode eStatus = MB_ENOERR ; 00133 int iRegIndex; 00134 00135 if( ( usAddress >= REG_INPUT_START ) 00136 && ( usAddress + usNRegs <= REG_INPUT_START + REG_INPUT_NREGS ) ) 00137 { 00138 iRegIndex = ( int )( usAddress - usRegInputStart ); 00139 while( usNRegs > 0 ) 00140 { 00141 *pucRegBuffer++ = ( unsigned char )( usRegInputBuf[iRegIndex] >> 8 ); 00142 *pucRegBuffer++ = ( unsigned char )( usRegInputBuf[iRegIndex] & 0xFF ); 00143 iRegIndex++; 00144 usNRegs--; 00145 } 00146 } 00147 else 00148 { 00149 eStatus = MB_ENOREG ; 00150 } 00151 return eStatus; 00152 } 00153 00154 eMBErrorCode 00155 eMBRegHoldingCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNRegs, eMBRegisterMode eMode ) 00156 { 00157 eMBErrorCode eStatus = MB_ENOERR ; 00158 int iRegIndex; 00159 00160 if( ( usAddress >= REG_HOLDING_START ) && 00161 ( usAddress + usNRegs <= REG_HOLDING_START + REG_HOLDING_NREGS ) ) 00162 { 00163 iRegIndex = ( int )( usAddress - usRegHoldingStart ); 00164 switch ( eMode ) 00165 { 00166 /* Pass current register values to the protocol stack. */ 00167 case MB_REG_READ : 00168 while( usNRegs > 0 ) 00169 { 00170 *pucRegBuffer++ = ( UCHAR ) ( usRegHoldingBuf[iRegIndex] >> 8 ); 00171 *pucRegBuffer++ = ( UCHAR ) ( usRegHoldingBuf[iRegIndex] & 0xFF ); 00172 iRegIndex++; 00173 usNRegs--; 00174 } 00175 break; 00176 00177 /* Update current register values with new values from the 00178 * protocol stack. */ 00179 case MB_REG_WRITE : 00180 while( usNRegs > 0 ) 00181 { 00182 usRegHoldingBuf[iRegIndex] = *pucRegBuffer++ << 8; 00183 usRegHoldingBuf[iRegIndex] |= *pucRegBuffer++; 00184 iRegIndex++; 00185 usNRegs--; 00186 } 00187 } 00188 } 00189 else 00190 { 00191 eStatus = MB_ENOREG ; 00192 } 00193 return eStatus; 00194 } 00195 00196 eMBErrorCode 00197 eMBRegCoilsCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNCoils, eMBRegisterMode eMode ) 00198 { 00199 return MB_ENOREG ; 00200 } 00201 00202 eMBErrorCode 00203 eMBRegDiscreteCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNDiscrete ) 00204 { 00205 return MB_ENOREG ; 00206 } 00207
Generated on Tue Jul 12 2022 21:29:48 by
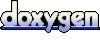