
This is a port of the mruby/c tutorial Chapter 03 to the mbed environment.
Embed:
(wiki syntax)
Show/hide line numbers
global.c
00001 00002 #include "value.h " 00003 #include "static.h" 00004 #include "vm_config.h" 00005 00006 /* 00007 00008 GLobal objects are stored in 'mrbc_global' array. 00009 'mrbc_global' array is decending order by sym_id. 00010 In case of searching a global object, binary search is used. 00011 In case of adding a global object, insertion sort is used. 00012 00013 */ 00014 00015 /* search */ 00016 static int search_global_object(mrb_sym sym_id) 00017 { 00018 int left = 0, right = MAX_GLOBAL_OBJECT_SIZE-1; 00019 while( left <= right ){ 00020 int mid = (left+right)/2; 00021 if( mrbc_global[mid].sym_id == sym_id ) return mid; 00022 if( mrbc_global[mid].sym_id < sym_id ){ 00023 right = mid - 1; 00024 } else { 00025 left = mid + 1; 00026 } 00027 } 00028 return -1; 00029 } 00030 00031 static int search_const(mrb_sym sym_id) { 00032 int left = 0, right = MAX_CONST_COUNT-1; 00033 while (left <= right) { 00034 int mid = (left + right) / 2; 00035 if ( mrbc_const[mid].sym_id == sym_id ) return mid; 00036 if ( mrbc_const[mid].sym_id < sym_id ) { 00037 right = mid - 1; 00038 } else { 00039 left = mid + 1; 00040 } 00041 } 00042 return -1; 00043 } 00044 00045 /* add */ 00046 void global_object_add(mrb_sym sym_id, mrb_object *obj) 00047 { 00048 int index = search_global_object(sym_id); 00049 if( index == -1 ){ 00050 index = MAX_GLOBAL_OBJECT_SIZE-1; 00051 while( index > 0 && mrbc_global[index].sym_id < sym_id ){ 00052 mrbc_global[index] = mrbc_global[index-1]; 00053 index--; 00054 } 00055 } 00056 mrbc_global[index].sym_id = sym_id; 00057 mrbc_global[index].obj = *obj; 00058 } 00059 00060 void const_add(mrb_sym sym_id, mrb_object *obj) 00061 { 00062 int index = search_const(sym_id); 00063 if( index == -1 ){ 00064 index = MAX_CONST_COUNT-1; 00065 while(index > 0 && mrbc_const[index].sym_id < sym_id ){ 00066 mrbc_const[index] = mrbc_const[index-1]; 00067 index--; 00068 } 00069 } 00070 mrbc_const[index].sym_id = sym_id; 00071 mrbc_const[index].obj = *obj; 00072 } 00073 00074 /* get */ 00075 mrb_object global_object_get(mrb_sym sym_id) 00076 { 00077 int index = search_global_object(sym_id); 00078 if( index >= 0 ){ 00079 return mrbc_global[index].obj; 00080 } else { 00081 /* nil */ 00082 mrb_object obj; 00083 obj.tt = MRB_TT_FALSE; 00084 return obj; 00085 } 00086 } 00087 00088 mrb_object const_get(mrb_sym sym_id) { 00089 int index = search_const(sym_id); 00090 if (index >= 0){ 00091 return mrbc_const[index].obj; 00092 } else { 00093 mrb_object obj; 00094 obj.tt = MRB_TT_FALSE; 00095 return obj; 00096 } 00097 } 00098
Generated on Tue Jul 12 2022 23:36:30 by
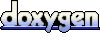