
Granulo Eldar Fahrudin Brbutović
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "sMotor.h" 00003 00004 #include <string> 00005 00006 00007 #define PIN1 dp13 00008 #define PIN2 dp11 00009 #define PIN3 dp10 00010 #define PIN4 dp9 00011 00012 Serial pc(USBTX, USBRX); //tx, rx 00013 sMotor stepper_motor(PIN1, PIN2, PIN3, PIN4); 00014 00015 int step_speed = 1200 ; // set default motor speed 00016 int numsteps = 512; 00017 int oldsteps = 0; 00018 00019 Ticker rotator; 00020 bool cw = true, working = true; 00021 00022 int speed =1200; 00023 00024 string buffer = ""; 00025 00026 bool is_digit(char c) { 00027 return c >= '0' && c <= '9'; 00028 } 00029 00030 void wrong_comm() { 00031 pc.printf("Wrong command\n"); 00032 buffer = ""; 00033 } 00034 00035 void rotate() { 00036 if(working) { 00037 cw ? stepper_motor.step(numsteps, 0, speed) : 00038 stepper_motor.step(numsteps, 1, speed); 00039 } 00040 } 00041 00042 int main() { 00043 00044 rotator.attach(&rotate, .001); 00045 00046 /* 00047 PROTOCOL: 00048 00049 Axxx - set angle to xxx (out of 360) 00050 Sxxx - set motor speed to xxx 00051 W - toggle turn motor on/off 00052 C - set cw/ccw rotation direction 00053 */ 00054 00055 while(true) { 00056 buffer.push_back(pc.getc()); 00057 if(buffer.length() == 4) { 00058 if(!is_digit(buffer[1]) || !is_digit(buffer[2]) || !is_digit(buffer[3])) 00059 wrong_comm(); 00060 int value = buffer[3] + buffer[2] * 10 + buffer[1] * 100; 00061 switch(buffer[0]) { 00062 case 'A': 00063 oldsteps = numsteps; 00064 numsteps = value; 00065 if(numsteps > oldsteps) 00066 stepper_motor.step(numsteps, 1, speed); 00067 else if(numsteps < oldsteps) stepper_motor.step(numsteps, 0, speed); 00068 buffer = ""; 00069 break; 00070 00071 case 'S': 00072 speed = value; 00073 buffer = ""; 00074 00075 break; 00076 00077 default: 00078 wrong_comm(); 00079 } 00080 } else if(buffer.length() == 1 && buffer[0] == 'W') 00081 working = !working; 00082 else if(buffer.length() == 1 && buffer[0] == 'C') 00083 cw = !cw; 00084 // stepper_motor.pins = 3; 00085 } 00086 00087 00088 }
Generated on Sun Jul 24 2022 10:38:05 by
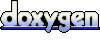