
tempcommit 13/05
Embed:
(wiki syntax)
Show/hide line numbers
epd4in2b.h
00001 /** 00002 * @filename : epd4in2.h 00003 * @brief : Header file for Dual-color e-paper library epd4in2.cpp 00004 * @author : Yehui from Waveshare 00005 * 00006 * Copyright (C) Waveshare August 10 2017 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documnetation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS OR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 */ 00026 00027 #ifndef EPD4IN2_H 00028 #define EPD4IN2_H 00029 00030 #include "epdif.h" 00031 00032 // Display resolution 00033 #define EPD_WIDTH 400 00034 #define EPD_HEIGHT 300 00035 00036 // EPD4IN2 commands 00037 #define PANEL_SETTING 0x00 00038 #define POWER_SETTING 0x01 00039 #define POWER_OFF 0x02 00040 #define POWER_OFF_SEQUENCE_SETTING 0x03 00041 #define POWER_ON 0x04 00042 #define POWER_ON_MEASURE 0x05 00043 #define BOOSTER_SOFT_START 0x06 00044 #define DEEP_SLEEP 0x07 00045 #define DATA_START_TRANSMISSION_1 0x10 00046 #define DATA_STOP 0x11 00047 #define DISPLAY_REFRESH 0x12 00048 #define DATA_START_TRANSMISSION_2 0x13 00049 #define LUT_FOR_VCOM 0x20 00050 #define LUT_WHITE_TO_WHITE 0x21 00051 #define LUT_BLACK_TO_WHITE 0x22 00052 #define LUT_WHITE_TO_BLACK 0x23 00053 #define LUT_BLACK_TO_BLACK 0x24 00054 #define PLL_CONTROL 0x30 00055 #define TEMPERATURE_SENSOR_COMMAND 0x40 00056 #define TEMPERATURE_SENSOR_SELECTION 0x41 00057 #define TEMPERATURE_SENSOR_WRITE 0x42 00058 #define TEMPERATURE_SENSOR_READ 0x43 00059 #define VCOM_AND_DATA_INTERVAL_SETTING 0x50 00060 #define LOW_POWER_DETECTION 0x51 00061 #define TCON_SETTING 0x60 00062 #define RESOLUTION_SETTING 0x61 00063 #define GSST_SETTING 0x65 00064 #define GET_STATUS 0x71 00065 #define AUTO_MEASUREMENT_VCOM 0x80 00066 #define READ_VCOM_VALUE 0x81 00067 #define VCM_DC_SETTING 0x82 00068 #define PARTIAL_WINDOW 0x90 00069 #define PARTIAL_IN 0x91 00070 #define PARTIAL_OUT 0x92 00071 #define PROGRAM_MODE 0xA0 00072 #define ACTIVE_PROGRAMMING 0xA1 00073 #define READ_OTP 0xA2 00074 #define POWER_SAVING 0xE3 00075 00076 00077 #define LOW 0; 00078 #define HIGH 1; 00079 class Epd : EpdIf { 00080 public: 00081 unsigned int width; 00082 unsigned int height; 00083 00084 Epd(); 00085 ~Epd(); 00086 int Init(void); 00087 void SendCommand(unsigned char command); 00088 void SendData(unsigned char data); 00089 void WaitUntilIdle(void); 00090 void Reset(void); 00091 void SetPartialWindow(const unsigned char* buffer_black, const unsigned char* buffer_red, int x, int y, int w, int l); 00092 void SetPartialWindowBlack(const unsigned char* buffer_black, int x, int y, int w, int l); 00093 void SetPartialWindowRed(const unsigned char* buffer_red, int x, int y, int w, int l); 00094 void DisplayFrame(const unsigned char* frame_black, const unsigned char* frame_red); 00095 void DisplayFrame(void); 00096 void ClearFrame(void); 00097 void Sleep(void); 00098 00099 private: 00100 00101 /* 00102 unsigned int reset_pin; 00103 unsigned int dc_pin; 00104 unsigned int cs_pin; 00105 unsigned int busy_pin; 00106 */ 00107 char rx_buffer[256]; 00108 char tx_buffer[256]; 00109 00110 DigitalOut dc_pin; 00111 DigitalOut busy_pin; 00112 DigitalOut reset_pin; 00113 //DigitalIn cs_pin; 00114 00115 00116 }; 00117 00118 #endif /* EPD4IN2_H */ 00119 00120 /* END OF FILE */
Generated on Thu Jul 14 2022 00:56:38 by
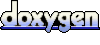