
tempcommit 13/05
Embed:
(wiki syntax)
Show/hide line numbers
Shed.h
00001 #ifndef SHED_H 00002 #define SHED_H 00003 #include <string> 00004 #include <sstream> 00005 #include <iostream> 00006 #include <vector> 00007 #include "Datetime.h" 00008 00009 using namespace std; 00010 /** 00011 * This class Shed can be used to act as one sheduled class (of many) 00012 * 00013 * @author Jordy Ampe 00014 * @version 1.0 00015 * @since 2019-05-08 00016 */ 00017 class Shed{ 00018 private: 00019 string lokaal; 00020 Datetime* start; 00021 Datetime* einde; 00022 string prof; 00023 string klasgroep; 00024 string vak; 00025 string comments; 00026 public: 00027 /** 00028 * Constructor to make a Shed from json as a string 00029 * @param json is a string with the received json for one shedules class from the API 00030 */ 00031 Shed(string json); 00032 00033 /** 00034 * Destructor for a Shed 00035 */ 00036 ~Shed(); 00037 00038 /** 00039 * @return lokaal as a string 00040 */ 00041 string getLokaal(); 00042 00043 /** 00044 * @return start as a Datetime* 00045 */ 00046 Datetime* getStart(); 00047 00048 /** 00049 * @return einde as a Datetime* 00050 */ 00051 Datetime* getEinde(); 00052 00053 /** 00054 * @return prof as a string 00055 */ 00056 string getProf(); 00057 00058 /** 00059 * @return Klasgroep as a string 00060 */ 00061 string getKlasgroep(); 00062 00063 /** 00064 * @return vak as a string 00065 */ 00066 string getVak(); 00067 00068 /** 00069 * @return comments as a string 00070 */ 00071 string getComments(); 00072 00073 /** 00074 * @param jsons is a vector of json formated shedules 00075 * @return * to the vector of Shed's 00076 */ 00077 static vector<Shed>* getShedVector(vector<string> jsons); 00078 }; 00079 #endif
Generated on Thu Jul 14 2022 00:56:38 by
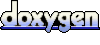