
tempcommit 13/05
Embed:
(wiki syntax)
Show/hide line numbers
Esp8266.cpp
00001 #include "Esp8266.h" 00002 #include <vector> 00003 using namespace std; 00004 00005 Esp8266::Esp8266(PinName TX, PinName RX, int BaudRate, PinName ResetPin, PinName ChipSelect) { 00006 rst = new DigitalOut(ResetPin); 00007 cs = new DigitalOut(ChipSelect); 00008 setupModule(TX, RX, BaudRate); 00009 } 00010 00011 void Esp8266::setupModule(PinName TX, PinName RX, int BaudRate) { 00012 cs->write(1); 00013 serial = new UARTSerial(TX, RX, BaudRate); 00014 parser = new ATCmdParser(serial); 00015 parser->debug_on(0); 00016 parser->set_delimiter("\r\n"); 00017 cs->write(0); 00018 } 00019 00020 void Esp8266::toggleResetPin(void) { 00021 rst->write(0); 00022 wait(0.01); 00023 rst->write(1); 00024 } 00025 00026 void Esp8266::setModuleMode(int Mode) { 00027 cs->write(1); 00028 toggleResetPin(); 00029 bool processed = false; 00030 do { 00031 processed = parser->send("AT+CWMODE=%d", Mode) && parser->recv("OK"); 00032 } 00033 while(processed == false); 00034 } 00035 00036 void Esp8266::connectToAP(const char* SSID, const char* Password) { 00037 bool processed = false; 00038 do { 00039 processed = parser->send("AT+CWJAP_CUR=\"%s\",\"%s\"", SSID, Password) && parser->recv("OK"); 00040 } 00041 while(processed == false); 00042 } 00043 00044 string Esp8266::getRequest(const char* TCPorUDP, const char* Server, int Port, const char* ConnectionString, bool stayAlive) { 00045 bool processed = false; 00046 do { 00047 processed = parser->send("AT+CIPSTART=\"%s\",\"%s\",%d", TCPorUDP, Server, Port) && parser->recv("OK"); 00048 } 00049 while(processed == false); 00050 00051 string con = "GET "; 00052 con.append(ConnectionString); 00053 con.append(" HTTP/1.1\r\nHost: "); 00054 con.append(Server); 00055 con.append("\r\n\r\n"); 00056 00057 do { 00058 processed = parser->send("AT+CIPSEND=%d", con.length()) && parser->recv("OK"); 00059 } 00060 while(processed == false); 00061 00062 parser->send("%s", con.c_str()); 00063 if(!stayAlive) { 00064 closeConnection(); 00065 } 00066 return getJsonString(); 00067 } 00068 00069 void Esp8266::closeConnection(void) { 00070 bool processed = false; 00071 do { 00072 processed = parser->send("AT+CIPCLOSE"); 00073 } 00074 while(processed == false); 00075 } 00076 00077 string Esp8266::getJsonString(void) { 00078 char previousChar = ' '; 00079 char presentChar = ' '; 00080 bool startOfJSON = false; 00081 00082 string resp = ""; 00083 00084 while(1) { 00085 presentChar = (char)parser->getc(); 00086 00087 if(presentChar == '{' && previousChar == '[') { 00088 startOfJSON = true; 00089 } 00090 00091 if(startOfJSON) { 00092 resp += presentChar; 00093 } 00094 00095 if(presentChar == ']' && previousChar == '}') { 00096 break; 00097 } 00098 00099 previousChar = presentChar; 00100 } 00101 00102 cs->write(0); 00103 return resp; 00104 } 00105 00106 vector<string> Esp8266::processJsonString(string response) { 00107 vector<string> jsonStrings; 00108 response = response.substr(0, response.length()-1); 00109 00110 int delimiterPlace = 0; 00111 do { 00112 delimiterPlace = response.find("},{")-2; 00113 string sub; 00114 if(delimiterPlace < 0) { 00115 if(response[0] != '{') 00116 sub = response.substr(2, response.length()-3); 00117 else 00118 sub = response.substr(1, response.length()-2); 00119 00120 jsonStrings.push_back(sub); 00121 break; 00122 } 00123 else { 00124 sub = response.substr(1, delimiterPlace+1); 00125 jsonStrings.push_back(sub); 00126 response.erase(0, delimiterPlace+4); 00127 } 00128 } 00129 while(delimiterPlace > 0); 00130 00131 return jsonStrings; 00132 }
Generated on Thu Jul 14 2022 00:56:38 by
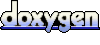