
tempcommit 13/05
Embed:
(wiki syntax)
Show/hide line numbers
Datetime.cpp
00001 #include "mbed.h" 00002 #include "Datetime.h" 00003 #include <string> 00004 #include <sstream> 00005 #include <iostream> 00006 using namespace std; 00007 00008 Datetime::Datetime(string datetime) { 00009 /* Voorbeeld datetime input 00010 "2019-03-22 15:45:00" 00011 */ 00012 this->year = atoi(datetime.substr(0,4).c_str()); 00013 this->month = atoi(datetime.substr(5,2).c_str()); 00014 this->day = atoi(datetime.substr(8,2).c_str()); 00015 this->hour = atoi(datetime.substr(11,2).c_str()); 00016 this->minute = atoi(datetime.substr(14,2).c_str()); 00017 this->second = atoi(datetime.substr(17,2).c_str()); 00018 } 00019 00020 Datetime::~Datetime() { 00021 //int's are freed automatically 00022 } 00023 00024 int Datetime::getDay () { 00025 return day; 00026 } 00027 00028 int Datetime::getMonth () { 00029 return month; 00030 } 00031 00032 int Datetime::getYear () { 00033 return year; 00034 } 00035 00036 int Datetime::getHour () { 00037 return hour; 00038 } 00039 00040 int Datetime::getMinute () { 00041 return minute; 00042 } 00043 00044 int Datetime::getSecond () { 00045 return second; 00046 } 00047 00048 string Datetime::getDate () { 00049 string date = ""; 00050 string buf; // string which will contain the result 00051 ostringstream convert0; // stream used for the conversion 00052 ostringstream convert1; 00053 ostringstream convert2; 00054 convert0 << day; // insert the textual representation of 'Number' in the characters in the stream 00055 buf = convert0.str(); // set 'Result' to the contents of the stream 00056 if(day < 10) {date += "0";} 00057 date += buf + "/"; 00058 if(month < 10) {date += "0";} 00059 convert1 << month; 00060 buf = convert1.str(); 00061 date += buf + "/"; 00062 convert2 << year; 00063 buf = convert2.str(); 00064 date += buf; 00065 return date; 00066 } 00067 00068 string Datetime::getTime () { 00069 string time = ""; 00070 string buf; // string which will contain the result 00071 ostringstream convert0; // stream used for the conversion 00072 ostringstream convert1; 00073 ostringstream convert2; 00074 convert0 << hour; // insert the textual representation of 'Number' in the characters in the stream 00075 buf = convert0.str(); // set 'Result' to the contents of the stream 00076 if(hour < 10) {time += "0";} 00077 time += buf + ":"; 00078 if(minute < 10) {time += "0";} 00079 convert1 << minute; 00080 buf = convert1.str(); 00081 time += buf + ":"; 00082 if(second < 10) {time += "0";} 00083 convert2 << second; 00084 buf = convert2.str(); 00085 time += buf; 00086 return time; 00087 } 00088 00089 string Datetime::getDatetime () { 00090 return getDate() + " " + getTime(); 00091 }
Generated on Thu Jul 14 2022 00:56:38 by
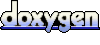