A library for adafruit's neo pixel ring and Addressable LED.
Embed:
(wiki syntax)
Show/hide line numbers
TI_NEOPIXEL.cpp
00001 #include "TI_NEOPIXEL.h" 00002 #include "mbed.h" 00003 00004 TI_NEOPIXEL::TI_NEOPIXEL(PinName input) : _ledStrip(input) { 00005 } 00006 00007 void TI_NEOPIXEL::switchLightOff(int count) { 00008 00009 rgb_color colors[count]; 00010 00011 for(int i = 0; i < count; i++) { 00012 colors[i] = (rgb_color) {0, 0, 0}; 00013 } 00014 00015 _ledStrip.write(colors, count); 00016 } 00017 00018 void TI_NEOPIXEL::switchLightOn(int count) { 00019 00020 rgb_color colors[count]; 00021 00022 for(int i = 0; i < count; i++) { 00023 colors[i] = (rgb_color) {50, 10, 170}; 00024 } 00025 00026 _ledStrip.write(colors, count); 00027 } 00028 00029 void TI_NEOPIXEL::changeColor(int count, rgb_color rgbColor) { 00030 00031 rgb_color colors[count]; 00032 00033 for(int i = 0; i < count; i++) { 00034 colors[i] = rgbColor; 00035 } 00036 00037 _ledStrip.write(colors, count); 00038 } 00039 00040 void TI_NEOPIXEL::changePointColor(int count, rgb_color topColor, rgb_color bottomColor) { 00041 00042 rgb_color colors[count]; 00043 00044 for(int i = 0; i < count; i++) { 00045 if (i == 0) { 00046 colors[i] = topColor; 00047 } else if (i == count/2) { 00048 colors[i] = bottomColor; 00049 } else { 00050 colors[i] = (rgb_color) {0, 0, 0}; 00051 } 00052 } 00053 00054 _ledStrip.write(colors, count); 00055 } 00056 00057 void TI_NEOPIXEL::circle(int count, rgb_color rgbColor) { 00058 00059 for(int j = 0; j < count; j++) { 00060 rgb_color colors[count]; 00061 00062 for(int i = 0; i < count; i++) { 00063 if (j >= i) { 00064 colors[i] = rgbColor; 00065 } else { 00066 colors[i] = (rgb_color) {0, 0, 0}; 00067 } 00068 } 00069 00070 _ledStrip.write(colors, count); 00071 wait(0.07); 00072 } 00073 } 00074 00075 void TI_NEOPIXEL::circleRainbow(int count) { 00076 00077 for(int j = 0; j < count; j++) { 00078 rgb_color colors[count]; 00079 00080 for(int i = 0; i < count; i++) { 00081 if (j >= i) { 00082 uint8_t phase = 256/count*i; 00083 colors[i] = convertHsvToRgb(phase / 256.0, 1.0, 1.0); 00084 } else { 00085 colors[i] = (rgb_color) {0, 0, 0}; 00086 } 00087 } 00088 00089 _ledStrip.write(colors, count); 00090 wait(0.07); 00091 } 00092 } 00093 00094 rgb_color TI_NEOPIXEL::convertHsvToRgb(float h, float s, float v) 00095 { 00096 int i = floor(h * 6); 00097 float f = h * 6 - i; 00098 float p = v * (1 - s); 00099 float q = v * (1 - f * s); 00100 float t = v * (1 - (1 - f) * s); 00101 float r = 0, g = 0, b = 0; 00102 00103 switch(i % 6){ 00104 case 0: r = v; g = t; b = p; break; 00105 case 1: r = q; g = v; b = p; break; 00106 case 2: r = p; g = v; b = t; break; 00107 case 3: r = p; g = q; b = v; break; 00108 case 4: r = t; g = p; b = v; break; 00109 case 5: r = v; g = p; b = q; break; 00110 } 00111 00112 return (rgb_color){r * 255, g * 255, b * 255}; 00113 }
Generated on Wed Jul 13 2022 16:00:03 by
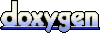