Simple neopixel (WS2812) library, tuned for stm32 (L432) at 80 MHz Should be compatible with any stm32, different clock speed may require timing adjustments in neopixel.c
Dependents: NEOPIXEL_SAMPLE ppd
neopixel.h
00001 #ifndef NEOPIXEL_H 00002 #define NEOPIXEL_H 00003 #include "mbed.h" 00004 00005 /* 00006 // Example 00007 00008 NeoPixelOut npx(D12); 00009 00010 int main() { 00011 wait(0.2); // wait for HSE to stabilize 00012 00013 npx.global_scale = 1.0f; // Adjust brightness 00014 npx.normalize = true; // Equalize brightness to make r + g + b = 255 00015 00016 Pixel strip[6]; 00017 strip[0].hex = 0xFF0000; 00018 strip[1].hex = 0xFFFF00; 00019 strip[2].hex = 0x00FF00; 00020 strip[3].hex = 0x00FFFF; 00021 strip[4].hex = 0x0000FF; 00022 strip[5].hex = 0xFF00FF; 00023 00024 npx.send(strip, 6); 00025 00026 while(1); 00027 } 00028 */ 00029 00030 00031 00032 /** 00033 * @brief Struct for easy manipulation of RGB colors. 00034 * 00035 * Set components in the xrgb.r (etc.) and you will get 00036 * the hex in xrgb.num. 00037 */ 00038 union Pixel { 00039 /** Struct for access to individual color components */ 00040 struct __attribute__((packed)) { 00041 uint8_t b; 00042 uint8_t g; 00043 uint8_t r; 00044 uint8_t a; // unused 00045 }; 00046 00047 /** RGB color as a single uint32_t */ 00048 uint32_t hex; 00049 }; 00050 00051 00052 class NeoPixelOut : DigitalOut { 00053 private: 00054 void byte(uint32_t b); 00055 00056 public: 00057 bool normalize; 00058 float global_scale; 00059 00060 NeoPixelOut(PinName pin); 00061 00062 void send(Pixel *colors, uint32_t count, bool flipwait=true); 00063 00064 /** Wait long enough to make the colors show up */ 00065 void flip(void); 00066 }; 00067 00068 00069 #endif /* NEOPIXEL_H */
Generated on Thu Jul 14 2022 05:25:24 by
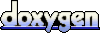