
Use Accelerometer and Joystick to mimic a mouse. This is for a class project. It is done for educational purpose. It is not practical to real world use.
Dependencies: C12832_lcd MMA7660 USBDevice mbed
accelestick.h
00001 // Name: T.H. Lu 00002 // Date: 03/27/2014 00003 // Description: 00004 // Header file for accelestick. Contains all defines and function declarations. 00005 // 00006 00007 #include <stdlib.h> 00008 #include "mbed.h" 00009 00010 #include "MMA7660.h" // Accelerometer API 00011 #include "C12832_lcd.h" // LCD API 00012 #include "USBMouseKeyboard.h"// USB Mouse API 00013 00014 #ifndef ACCELESTICK_H 00015 #define ACCELESTICK_H 00016 00017 #define SCALE_X 50 // float to int conversion factor 00018 #define SCALE_Y SCALE_X 00019 #define SCALE_Z SCALE_X 00020 #define NOISE_FLOOR 0.10*SCALE_X // zero out any integer g value less than NOISE_FLOOR 00021 00022 #define ACCEL_MIN -1.5 00023 #define ACCEL_MAX 1.5 00024 00025 #define CALIB_SMPLS 16 // number of samples used for calibrating accelerometer 00026 00027 // Joystick button values based on joyb declaration 00028 enum {JS_NONE=0, JS_DOWN=1, JS_LEFT=2, JS_CENTER=4, JS_UP=8, JS_RIGHT=16}; 00029 enum {LED_LOCK=0, LED_DEBUG, LED_CALIB, LED_ALIVE}; 00030 00031 // structure to store max and min g values for debugging 00032 typedef struct s_max_min { 00033 float max_x, max_y, max_z; 00034 float min_x, min_y, min_z; 00035 } Max_min_t; 00036 00037 // structure for accelerometer int g values 00038 typedef struct s_int_g { 00039 int16_t x, y, z; 00040 } G_int_t; 00041 00042 // structure for accelerometer float g values 00043 typedef struct s_float_pos { 00044 float x, y, z; 00045 } G_float_t; 00046 00047 // structure to store all mouse related data 00048 typedef struct 00049 { 00050 int16_t x, y, z; // x, y, z position 00051 uint8_t button; // left button, 1 = left, 2 = center, 4 = middle 00052 bool dc; // double click 00053 int8_t scroll; // <0 to go up, >0 to go down 00054 } Mouse_state_t; 00055 00056 00057 //--- Functions Declaration ---// 00058 00059 // scan joystick for mouse buttons and scroll 00060 void get_joystick_input(); 00061 00062 // sample accelerometer values for mouse movement 00063 void sample_mma(); 00064 00065 // calibrate accelerometer 00066 void calib_mma(G_int_t current); 00067 00068 // update mouse position and buttons 00069 void update_mouse(); 00070 00071 // print debug messages to USB serial 00072 void print_debug_msg(); 00073 00074 // filter out accelestick noise 00075 int16_t filter_noise(int16_t const); 00076 00077 // flash specified LED. Needed 2 copies because Ticker cannot attach functions with parameters 00078 void flash_led2(); 00079 void flash_led3(); 00080 00081 // convert floating MMA g value into integer 00082 G_int_t conv_g2int(G_float_t mma_g); 00083 00084 int8_t init_accelestick(); 00085 void run_accelestick(); 00086 00087 #endif
Generated on Mon Jul 25 2022 03:58:30 by
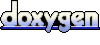