Wakeup Light with touch user interface, anti-aliased Font, SD card access and RTC usage on STM32F746NG-DISCO board
Dependencies: BSP_DISCO_F746NG_patch_fixed LCD_DISCO_F746NG TS_DISCO_F746NG FATFileSystem TinyJpgDec_interwork mbed-src
UI.cpp
00001 #include "WakeupLight.h" 00002 00003 #include "Images/ic_navigate_before_white_24dp_1x.h" 00004 #include "Images/ic_notifications_none_white_48dp_1x.h" 00005 #include "Images/ic_query_builder_white_48dp_1x.h" 00006 #include "Images/ic_slideshow_white_48dp_1x.h" 00007 #include "Images/ic_visibility_off_white_48dp_1x.h" 00008 #include "Images/ic_visibility_white_48dp_1x.h" 00009 #include "Images/ic_equalizer_white_48dp_1x.h" 00010 #include "Images/ic_settings_white_48dp_1x.h" 00011 00012 #define DEFAULT_FONT &calibri_36ptFontInfo 00013 00014 #define CLIENT_COLOR_BG ((uint32_t)0xFF000000) 00015 #define CLIENT_COLOR_FG ((uint32_t)0xFFD0D0D0) 00016 #define CLIENT_FONT DEFAULT_FONT 00017 00018 #define HEADER_HEIGHT 26 00019 #define HEADER_COLOR_BG ((uint32_t)0xFF000000) 00020 #define HEADER_LINE ((uint32_t)0xFF008ED8) 00021 #define HEADER_COLOR_FG ((uint32_t)0xFFD3D3D3) 00022 #define HEADER_FONT DEFAULT_FONT 00023 00024 #define CLOCK_COLOR_BG ((uint32_t)0xFF000000) 00025 #define CLOCK_COLOR_FG ((uint32_t)0xFF7070A0) 00026 #define CLOCK_FONT &trebuchetMS_270ptFontInfo 00027 00028 #define CLOCK_IN_WORDS_CELL_WIDTH 22 00029 #define CLOCK_IN_WORDS_CELL_HEIGHT 22 00030 #define CLOCK_IN_WORDS_COLOR_FG_ACTIVE HEADER_COLOR_FG 00031 #define CLOCK_IN_WORDS_COLOR_FG_INACTIVE ((uint32_t)0xFF202020) 00032 00033 #define CLOCK_IN_WORDS_TYPE_ES 0x000001 00034 #define CLOCK_IN_WORDS_TYPE_IST 0x000002 00035 #define CLOCK_IN_WORDS_TYPE_FUENF_1 0x000004 00036 #define CLOCK_IN_WORDS_TYPE_ZEHN_1 0x000008 00037 #define CLOCK_IN_WORDS_TYPE_ZWANZIG 0x000010 00038 #define CLOCK_IN_WORDS_TYPE_DREI_1 0x000020 00039 #define CLOCK_IN_WORDS_TYPE_VIERTEL 0x000040 00040 #define CLOCK_IN_WORDS_TYPE_VOR 0x000080 00041 #define CLOCK_IN_WORDS_TYPE_NACH 0x000100 00042 #define CLOCK_IN_WORDS_TYPE_HALB 0x000200 00043 #define CLOCK_IN_WORDS_TYPE_ELF 0x000400 00044 #define CLOCK_IN_WORDS_TYPE_FUENF_2 0x000800 00045 #define CLOCK_IN_WORDS_TYPE_EINS 0x001000 00046 #define CLOCK_IN_WORDS_TYPE_ZWEI 0x002000 00047 #define CLOCK_IN_WORDS_TYPE_DREI_2 0x004000 00048 #define CLOCK_IN_WORDS_TYPE_VIER 0x008000 00049 #define CLOCK_IN_WORDS_TYPE_SECHS 0x010000 00050 #define CLOCK_IN_WORDS_TYPE_ACHT 0x020000 00051 #define CLOCK_IN_WORDS_TYPE_SIEBEN 0x040000 00052 #define CLOCK_IN_WORDS_TYPE_ZWOELF 0x080000 00053 #define CLOCK_IN_WORDS_TYPE_ZEHN_2 0x100000 00054 #define CLOCK_IN_WORDS_TYPE_NEUN 0x200000 00055 #define CLOCK_IN_WORDS_TYPE_UHR 0x400000 00056 00057 #define SLIDESHOW_COLOR_BG ((uint32_t)0xFF000000) 00058 #define SLIDESHOW_COLOR_FG ((uint32_t)0xFFAFAFAF) 00059 #define SLIDESHOW_TRANSPARENCY 128 00060 #define SLIDESHOW_FADE_STEP 5 00061 #define SLIDESHOW_TIMEOUT 15 00062 #define SLIDESHOW_FONT &trebuchetMS_135ptFontInfo 00063 00064 #define BUTTON_WIDTH 100 00065 #define BUTTON_HEIGHT 60 00066 #define BUTTON_SMALL_WIDTH 50 00067 #define BUTTON_SMALL_HEIGHT 40 00068 #define BUTTON_COLOR_BG ((uint32_t)0x00000000) 00069 #define BUTTON_COLOR_FG CLIENT_COLOR_FG 00070 #define BUTTON_COLOR_BG_START 0x008ED8 // 0x0C2696 00071 #define BUTTON_COLOR_BG_END 0x004366 // 0x07185E 00072 #define BUTTON_COLOR_BG_START_INACTIVE 0x515151 00073 #define BUTTON_COLOR_BG_END_INACTIVE 0x333333 00074 #define BUTTON_FONT DEFAULT_FONT 00075 00076 #define COLOR_BG ((uint32_t)0xFF000000) 00077 00078 #define MAX_BOXES_PER_LINE 3 00079 #define BOX_SPACING 10 00080 #define BOX_TEXT_SPACING 10 00081 #define BOX_COLOR_BG BUTTON_COLOR_BG 00082 #define BOX_COLOR_FG CLIENT_COLOR_FG 00083 #define BOX_COLOR_BG_START BUTTON_COLOR_BG_START 00084 #define BOX_COLOR_BG_END BUTTON_COLOR_BG_END 00085 #define BOX_FONT DEFAULT_FONT 00086 00087 LCD_DISCO_F746NG uiLcd; 00088 TS_DISCO_F746NG uiTs; 00089 uint16_t uiLastTouchX; 00090 uint16_t uiLastTouchY; 00091 int lastSlideshowTick=0; 00092 struct tm lastClockUpdateTime; 00093 UI_STRUCT *uiCurrent=NULL; 00094 UI_STRUCT uiClock; 00095 UI_STRUCT uiClockInWords; 00096 UI_STRUCT uiColorTest; 00097 UI_STRUCT uiWakeup; 00098 UI_STRUCT uiSlideshow; 00099 UI_STRUCT uiMain; 00100 static UI_BOX_LIST_ITEM_STRUCT uiMainItems[]= 00101 { 00102 { "Clock", ic_query_builder_white_48dp_1x }, { "Clock\nIn Words", ic_query_builder_white_48dp_1x }, { "Slideshow", ic_slideshow_white_48dp_1x }, { "Adjust\nTimers", ic_notifications_none_white_48dp_1x }, { "Settings", ic_settings_white_48dp_1x }, { "Lights\nOn", ic_visibility_white_48dp_1x }, { "Lights\nOff", ic_visibility_off_white_48dp_1x }, { "My Color", ic_equalizer_white_48dp_1x } 00103 }; 00104 00105 // 00106 // helper function 00107 // 00108 void UI_ShowClearClientRect(void) 00109 { 00110 uiLcd.SetTextColor(CLIENT_COLOR_BG); 00111 uiLcd.FillRect(0,HEADER_HEIGHT,uiLcd.GetXSize(),uiLcd.GetYSize()-HEADER_HEIGHT); 00112 } 00113 00114 void UI_ShowDisplayText(int16_t x,int16_t y,char *text,const FONT_INFO *pFont,uint32_t colorText,uint32_t colorBack) 00115 { 00116 int16_t xStart; 00117 00118 xStart=x; 00119 00120 while ((*text)!='\0') 00121 { 00122 if ((*text)=='\n') 00123 { 00124 y+=FontX_GetHeight(pFont); 00125 x=xStart; 00126 } 00127 else 00128 { 00129 x+=FontX_DisplayChar(x,y,*text,pFont,colorText,colorBack); 00130 } 00131 00132 text++; 00133 } 00134 } 00135 00136 void UI_ShowDisplayTextCenter(int16_t x,int16_t y,int16_t width,int16_t height,char *text,const FONT_INFO *pFont,uint32_t colorText,uint32_t colorBack) 00137 { 00138 if (height!=-1) 00139 y+=(height-FontX_GetHeight(pFont))/2; 00140 00141 FontX_DisplayStringAt(x,y,width,text,ALIGN_CENTER,pFont,colorText,colorBack); 00142 } 00143 00144 void UI_ShowDrawGradientButton(uint16_t x,uint16_t y,uint16_t width,uint16_t height,uint32_t colorStart,uint32_t colorEnd) 00145 { 00146 float deltaRed; 00147 float deltaGreen; 00148 float deltaBlue; 00149 float red; 00150 float green; 00151 float blue; 00152 uint16_t offset; 00153 uint32_t color; 00154 00155 deltaRed=(float)((int32_t)(colorEnd & 0xFF) - (int32_t)(colorStart & 0xFF))/height; 00156 deltaGreen=(float)((int32_t)((colorEnd >> 8) & 0xFF) - (int32_t)((colorStart >> 8)& 0xFF))/height; 00157 deltaBlue=(float)((int32_t)((colorEnd >> 16) & 0xFF) - (int32_t)((colorStart >> 16)& 0xFF))/height; 00158 00159 red=colorStart & 0xFF; 00160 green=((colorStart >> 8)& 0xFF); 00161 blue=((colorStart >> 16)& 0xFF); 00162 00163 for (offset=0;offset<height;offset++) 00164 { 00165 color=(uint8_t)red | (((uint8_t)green)<<8) | (((uint8_t)blue)<<16) | (((uint32_t)0xFF)<<24); 00166 uiLcd.SetTextColor(color); 00167 00168 if ((offset==0) || (offset==(height-1))) 00169 uiLcd.DrawHLine(x+1,y+offset,width-2); 00170 else 00171 uiLcd.DrawHLine(x,y+offset,width); 00172 00173 red+=deltaRed; 00174 green+=deltaGreen; 00175 blue+=deltaBlue; 00176 } 00177 } 00178 00179 void UI_ShowDrawButtonEx(uint16_t x,uint16_t y,uint16_t width,uint16_t height,char *text,bool active) 00180 { 00181 // paint button background 00182 if (active==true) 00183 UI_ShowDrawGradientButton(x,y,width,height,BUTTON_COLOR_BG_START,BUTTON_COLOR_BG_END); 00184 else 00185 UI_ShowDrawGradientButton(x,y,width,height,BUTTON_COLOR_BG_START_INACTIVE,BUTTON_COLOR_BG_END_INACTIVE); 00186 00187 // paint button text 00188 UI_ShowDisplayTextCenter(x,y,width,height,text,BUTTON_FONT,BUTTON_COLOR_FG,BUTTON_COLOR_BG); 00189 } 00190 00191 void UI_ShowDrawButton(uint16_t x,uint16_t y,uint16_t width,uint16_t height,char *text) 00192 { 00193 UI_ShowDrawButtonEx(x,y,width,height,text,true); 00194 } 00195 00196 void UI_DrawBitmapWithAlpha(uint32_t Xpos,uint32_t Ypos,uint8_t *pbmp) 00197 { 00198 uint32_t index = 0, width = 0, height = 0, bit_pixel = 0; 00199 uint32_t x; 00200 uint32_t part; 00201 uint32_t color[3]; 00202 uint32_t value; 00203 00204 /* Get bitmap data address offset */ 00205 index = *(__IO uint16_t *) (pbmp + 10); 00206 index |= (*(__IO uint16_t *) (pbmp + 12)) << 16; 00207 00208 /* Read bitmap width */ 00209 width = *(uint16_t *) (pbmp + 18); 00210 width |= (*(uint16_t *) (pbmp + 20)) << 16; 00211 00212 /* Read bitmap height */ 00213 height = *(uint16_t *) (pbmp + 22); 00214 height |= (*(uint16_t *) (pbmp + 24)) << 16; 00215 00216 /* Read bit/pixel */ 00217 bit_pixel = *(uint16_t *) (pbmp + 28); 00218 00219 /* Get the layer pixel format */ 00220 if ((bit_pixel/8) != 4) 00221 { 00222 DPrintf("UI_DrawBitmapWithAlpha: This is no alpha picture.\r\n"); 00223 return; 00224 } 00225 00226 /* Bypass the bitmap header */ 00227 pbmp += (index + (width * (height - 1) * (bit_pixel/8))); 00228 00229 for(index=0; index < height; index++) 00230 { 00231 for (x=0;x<width;x++) 00232 { 00233 value=uiLcd.ReadPixel(Xpos+x,Ypos+index); 00234 00235 color[0]=value & 0xFF; 00236 color[1]=(value >> 8) & 0xFF; 00237 color[2]=(value >> 16) & 0xFF; 00238 00239 if (pbmp[3]>0) 00240 { 00241 // add red 00242 part=(color[0]+(pbmp[0]*pbmp[3]/255)); 00243 if (part>255) 00244 part=255; 00245 color[0]=part; 00246 00247 // add green 00248 part=(color[1]+(pbmp[1]*pbmp[3]/255)); 00249 if (part>255) 00250 part=255; 00251 color[1]=part; 00252 00253 // add blue 00254 part=(color[2]+(pbmp[2]*pbmp[3]/255)); 00255 if (part>255) 00256 part=255; 00257 color[2]=part; 00258 00259 // draw pixel 00260 uiLcd.DrawPixel(Xpos+x,Ypos+index,color[0] | (color[1] << 8) | (color[2] << 16) | ((uint32_t)0xFF << 24)); 00261 } 00262 00263 pbmp+=4; 00264 } 00265 00266 /* Increment the source and destination buffers */ 00267 pbmp -= 2*(width*(bit_pixel/8)); 00268 } 00269 } 00270 00271 // 00272 // box list 00273 // 00274 void UI_ShowBoxList(bool initial) 00275 { 00276 if (initial==true) 00277 { 00278 int8_t lines; 00279 int8_t columns; 00280 int8_t box; 00281 int16_t width; 00282 int16_t height; 00283 int16_t startX; 00284 int16_t startY; 00285 00286 // fill background 00287 UI_ShowClearClientRect(); 00288 00289 // paint boxes 00290 if (uiCurrent->data.boxList.count<=MAX_BOXES_PER_LINE) 00291 lines=1; 00292 else 00293 lines=2; 00294 00295 columns=((uiCurrent->data.boxList.count + (lines-1)) / lines); 00296 00297 width=(uiLcd.GetXSize() - BOX_SPACING) / columns; 00298 height=(uiLcd.GetYSize() - HEADER_HEIGHT - BOX_SPACING) / lines; 00299 00300 for (box=0;box<uiCurrent->data.boxList.count;box++) 00301 { 00302 startX=BOX_SPACING+(width*(box % columns)); 00303 startY=HEADER_HEIGHT+BOX_SPACING+(height*(box/columns)); 00304 00305 // paint box background 00306 UI_ShowDrawGradientButton(startX,startY,width-BOX_SPACING,height-BOX_SPACING,BOX_COLOR_BG_START,BOX_COLOR_BG_END); 00307 00308 // paint box text 00309 UI_ShowDisplayText(startX+BOX_TEXT_SPACING,startY+BOX_TEXT_SPACING,uiCurrent->data.boxList.items[box].name,BOX_FONT,BOX_COLOR_FG,BOX_COLOR_BG); 00310 00311 // draw icon 00312 if (uiCurrent->data.boxList.items[box].image!=NULL) 00313 UI_DrawBitmapWithAlpha(startX+width-BOX_SPACING-48,startY+height-BOX_SPACING-48,uiCurrent->data.boxList.items[box].image); 00314 } 00315 } 00316 } 00317 00318 void UI_ClickBoxList(uint16_t x,uint16_t y) 00319 { 00320 int8_t lines; 00321 int8_t columns; 00322 int8_t box; 00323 int16_t width; 00324 int16_t height; 00325 int16_t startX; 00326 int16_t startY; 00327 00328 // detect at which box was clicked 00329 if (uiCurrent->data.boxList.count<=MAX_BOXES_PER_LINE) 00330 lines=1; 00331 else 00332 lines=2; 00333 00334 columns=((uiCurrent->data.boxList.count + (lines-1)) / lines); 00335 00336 width=(uiLcd.GetXSize() - BOX_SPACING) / columns; 00337 height=(uiLcd.GetYSize() - HEADER_HEIGHT - BOX_SPACING) / lines; 00338 00339 for (box=0;box<uiCurrent->data.boxList.count;box++) 00340 { 00341 startX=BOX_SPACING+(width*(box % columns)); 00342 startY=HEADER_HEIGHT+BOX_SPACING+(height*(box/columns)); 00343 00344 if ( (x>=startX) && (x<(startX+width-BOX_SPACING)) 00345 && 00346 (y>=startY) && (y<(startY+height-BOX_SPACING)) 00347 ) 00348 { 00349 uiCurrent->handler(UR_CLICK,box,uiCurrent); 00350 break; 00351 } 00352 } 00353 } 00354 00355 // 00356 // clock 00357 // 00358 void UI_ShowClock(bool initial) 00359 { 00360 char buffer[100]; 00361 struct tm *tmStruct; 00362 00363 RTC_Get(&tmStruct); 00364 00365 if ((initial==true) || (tmStruct->tm_hour!=lastClockUpdateTime.tm_hour) || (tmStruct->tm_min!=lastClockUpdateTime.tm_min)) 00366 { 00367 // fill background 00368 uiLcd.SetTextColor(CLOCK_COLOR_BG); 00369 uiLcd.FillRect(0,0,uiLcd.GetXSize(),uiLcd.GetYSize()); 00370 00371 // show clock 00372 if (tmStruct->tm_hour>=10) 00373 snprintf(buffer,sizeof(buffer),"%u %u : %u %u",tmStruct->tm_hour / 10,tmStruct->tm_hour % 10,tmStruct->tm_min / 10,tmStruct->tm_min % 10); 00374 else 00375 snprintf(buffer,sizeof(buffer),"%u : %u %u",tmStruct->tm_hour,tmStruct->tm_min / 10,tmStruct->tm_min % 10); 00376 00377 UI_ShowDisplayTextCenter(0,0,uiLcd.GetXSize(),uiLcd.GetYSize(),buffer,CLOCK_FONT,CLOCK_COLOR_FG,CLOCK_COLOR_BG); 00378 00379 lastClockUpdateTime=*tmStruct; 00380 } 00381 } 00382 00383 void UI_ClickClock(uint16_t x,uint16_t y) 00384 { 00385 // exit view 00386 UI_Show(&uiMain); 00387 } 00388 00389 // 00390 // clock in words 00391 // 00392 void UI_ShowClockInWordsText(int16_t x,int16_t y,char *text,uint32_t type,uint32_t flags) 00393 { 00394 char buffer[2]; 00395 uint32_t colorText; 00396 00397 if ((flags & type)!=0) 00398 colorText=CLOCK_IN_WORDS_COLOR_FG_ACTIVE; 00399 else 00400 colorText=CLOCK_IN_WORDS_COLOR_FG_INACTIVE; 00401 00402 while ((*text)!='\0') 00403 { 00404 buffer[0]=*text; 00405 buffer[1]='\0'; 00406 00407 UI_ShowDisplayTextCenter(x+119,y+43,CLOCK_IN_WORDS_CELL_WIDTH,CLOCK_IN_WORDS_CELL_HEIGHT,buffer,CLIENT_FONT,colorText,CLOCK_COLOR_BG); 00408 00409 text++; 00410 x+=CLOCK_IN_WORDS_CELL_WIDTH; 00411 } 00412 } 00413 00414 void UI_ShowClockInWords(bool initial) 00415 { 00416 struct tm *tmStruct; 00417 uint32_t flags; 00418 int8_t minutes; 00419 int8_t hour; 00420 00421 RTC_Get(&tmStruct); 00422 00423 if ((initial==true) || (tmStruct->tm_hour!=lastClockUpdateTime.tm_hour) || (tmStruct->tm_min!=lastClockUpdateTime.tm_min)) 00424 { 00425 // fill background 00426 UI_ShowClearClientRect(); 00427 00428 // show clock 00429 00430 /* 00431 ES K IST A FÜNF 00432 ZEHN ZWANZIG 00433 DREI VIERTEL 00434 VOR FUNK NACH 00435 HALB A ELFÜNF 00436 EINS XAM ZWEI 00437 DREI PMJ VIER 00438 SECHS NL ACHT 00439 SIEBEN ZWÖLF 00440 ZEHNEUN K UHR 00441 */ 00442 00443 flags=0; 00444 00445 minutes=tmStruct->tm_min; 00446 hour=tmStruct->tm_hour; 00447 00448 minutes=((minutes+2)/5)*5; 00449 if (minutes==5) 00450 flags|=CLOCK_IN_WORDS_TYPE_FUENF_1 | CLOCK_IN_WORDS_TYPE_NACH; 00451 else if (minutes==10) 00452 flags|=CLOCK_IN_WORDS_TYPE_ZEHN_1 | CLOCK_IN_WORDS_TYPE_NACH; 00453 else if (minutes==15) 00454 flags|=CLOCK_IN_WORDS_TYPE_VIERTEL | CLOCK_IN_WORDS_TYPE_NACH; 00455 else if (minutes==20) 00456 flags|=CLOCK_IN_WORDS_TYPE_ZWANZIG | CLOCK_IN_WORDS_TYPE_NACH; 00457 else if (minutes==25) 00458 flags|=CLOCK_IN_WORDS_TYPE_ZWANZIG | CLOCK_IN_WORDS_TYPE_NACH; 00459 else if (minutes==30) 00460 flags|=CLOCK_IN_WORDS_TYPE_HALB; 00461 else if (minutes==35) 00462 flags|=CLOCK_IN_WORDS_TYPE_HALB; 00463 else if (minutes==40) 00464 { 00465 flags|=CLOCK_IN_WORDS_TYPE_ZWANZIG | CLOCK_IN_WORDS_TYPE_VOR; 00466 hour++; 00467 } 00468 else if (minutes==45) 00469 { 00470 flags|=CLOCK_IN_WORDS_TYPE_VIERTEL | CLOCK_IN_WORDS_TYPE_VOR; 00471 hour++; 00472 } 00473 else if (minutes==50) 00474 { 00475 flags|=CLOCK_IN_WORDS_TYPE_ZEHN_1 | CLOCK_IN_WORDS_TYPE_VOR; 00476 hour++; 00477 } 00478 else if (minutes==55) 00479 { 00480 flags|=CLOCK_IN_WORDS_TYPE_FUENF_1 | CLOCK_IN_WORDS_TYPE_VOR; 00481 hour++; 00482 } 00483 00484 hour=hour%12; 00485 if (hour==0) 00486 flags|=CLOCK_IN_WORDS_TYPE_ZWOELF; 00487 else if (hour==1) 00488 flags|=CLOCK_IN_WORDS_TYPE_EINS; 00489 else if (hour==2) 00490 flags|=CLOCK_IN_WORDS_TYPE_ZWEI; 00491 else if (hour==3) 00492 flags|=CLOCK_IN_WORDS_TYPE_DREI_2; 00493 else if (hour==4) 00494 flags|=CLOCK_IN_WORDS_TYPE_VIER; 00495 else if (hour==5) 00496 flags|=CLOCK_IN_WORDS_TYPE_FUENF_2; 00497 else if (hour==6) 00498 flags|=CLOCK_IN_WORDS_TYPE_SECHS; 00499 else if (hour==7) 00500 flags|=CLOCK_IN_WORDS_TYPE_SIEBEN; 00501 else if (hour==8) 00502 flags|=CLOCK_IN_WORDS_TYPE_ACHT; 00503 else if (hour==9) 00504 flags|=CLOCK_IN_WORDS_TYPE_NEUN; 00505 else if (hour==10) 00506 flags|=CLOCK_IN_WORDS_TYPE_ZEHN_2; 00507 else if (hour==11) 00508 flags|=CLOCK_IN_WORDS_TYPE_ELF; 00509 00510 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*0,CLOCK_IN_WORDS_CELL_HEIGHT*0,"ES",1,1); 00511 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*2,CLOCK_IN_WORDS_CELL_HEIGHT*0,"K",0,1); 00512 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*3,CLOCK_IN_WORDS_CELL_HEIGHT*0,"IST",1,1); 00513 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*6,CLOCK_IN_WORDS_CELL_HEIGHT*0,"A",0,1); 00514 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*7,CLOCK_IN_WORDS_CELL_HEIGHT*0,"F\xDCNF",CLOCK_IN_WORDS_TYPE_FUENF_1,flags); 00515 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*0,CLOCK_IN_WORDS_CELL_HEIGHT*1,"ZEHN",CLOCK_IN_WORDS_TYPE_ZEHN_1,flags); 00516 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*4,CLOCK_IN_WORDS_CELL_HEIGHT*1,"ZWANZIG",CLOCK_IN_WORDS_TYPE_ZWANZIG,flags); 00517 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*0,CLOCK_IN_WORDS_CELL_HEIGHT*2,"DREI",CLOCK_IN_WORDS_TYPE_DREI_1,flags); 00518 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*4,CLOCK_IN_WORDS_CELL_HEIGHT*2,"VIERTEL",CLOCK_IN_WORDS_TYPE_VIERTEL,flags); 00519 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*0,CLOCK_IN_WORDS_CELL_HEIGHT*3,"VOR",CLOCK_IN_WORDS_TYPE_VOR,flags); 00520 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*3,CLOCK_IN_WORDS_CELL_HEIGHT*3,"FUNK",0,1); 00521 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*7,CLOCK_IN_WORDS_CELL_HEIGHT*3,"NACH",CLOCK_IN_WORDS_TYPE_NACH,flags); 00522 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*0,CLOCK_IN_WORDS_CELL_HEIGHT*4,"HALB",CLOCK_IN_WORDS_TYPE_HALB,flags); 00523 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*4,CLOCK_IN_WORDS_CELL_HEIGHT*4,"A",0,1); 00524 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*5,CLOCK_IN_WORDS_CELL_HEIGHT*4,"EL",CLOCK_IN_WORDS_TYPE_ELF,flags); 00525 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*7,CLOCK_IN_WORDS_CELL_HEIGHT*4,"F",CLOCK_IN_WORDS_TYPE_ELF | CLOCK_IN_WORDS_TYPE_FUENF_2,flags); 00526 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*8,CLOCK_IN_WORDS_CELL_HEIGHT*4,"\xDCNF",CLOCK_IN_WORDS_TYPE_FUENF_2,flags); 00527 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*0,CLOCK_IN_WORDS_CELL_HEIGHT*5,"EINS",CLOCK_IN_WORDS_TYPE_EINS,flags); 00528 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*4,CLOCK_IN_WORDS_CELL_HEIGHT*5,"XAM",0,1); 00529 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*7,CLOCK_IN_WORDS_CELL_HEIGHT*5,"ZWEI",CLOCK_IN_WORDS_TYPE_ZWEI,flags); 00530 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*0,CLOCK_IN_WORDS_CELL_HEIGHT*6,"DREI",CLOCK_IN_WORDS_TYPE_DREI_2,flags); 00531 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*4,CLOCK_IN_WORDS_CELL_HEIGHT*6,"PMJ",0,1); 00532 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*7,CLOCK_IN_WORDS_CELL_HEIGHT*6,"VIER",CLOCK_IN_WORDS_TYPE_VIER,flags); 00533 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*0,CLOCK_IN_WORDS_CELL_HEIGHT*7,"SECHS",CLOCK_IN_WORDS_TYPE_SECHS,flags); 00534 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*5,CLOCK_IN_WORDS_CELL_HEIGHT*7,"NL",0,1); 00535 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*7,CLOCK_IN_WORDS_CELL_HEIGHT*7,"ACHT",CLOCK_IN_WORDS_TYPE_ACHT,flags); 00536 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*0,CLOCK_IN_WORDS_CELL_HEIGHT*8,"SIEBEN",CLOCK_IN_WORDS_TYPE_SIEBEN,flags); 00537 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*6,CLOCK_IN_WORDS_CELL_HEIGHT*8,"ZW\xD6LF",CLOCK_IN_WORDS_TYPE_ZWOELF,flags); 00538 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*0,CLOCK_IN_WORDS_CELL_HEIGHT*9,"ZEH",CLOCK_IN_WORDS_TYPE_ZEHN_2,flags); 00539 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*3,CLOCK_IN_WORDS_CELL_HEIGHT*9,"N",CLOCK_IN_WORDS_TYPE_ZEHN_2 | CLOCK_IN_WORDS_TYPE_NEUN,flags); 00540 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*4,CLOCK_IN_WORDS_CELL_HEIGHT*9,"EUN",CLOCK_IN_WORDS_TYPE_NEUN,flags); 00541 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*7,CLOCK_IN_WORDS_CELL_HEIGHT*9,"K",0,1); 00542 UI_ShowClockInWordsText(CLOCK_IN_WORDS_CELL_WIDTH*8,CLOCK_IN_WORDS_CELL_HEIGHT*9,"UHR",1,1); 00543 00544 lastClockUpdateTime=*tmStruct; 00545 } 00546 00547 /* 00548 // draw charset 00549 int x; 00550 for (x=0x80;x<=0xff;x++) 00551 { 00552 FontX_DisplayChar( 1+(((x-0x80) % 16)*14), 00553 30+(((x-0x80)/16)*20), 00554 x, 00555 CLIENT_FONT, 00556 CLIENT_COLOR_FG, 00557 CLIENT_COLOR_BG); 00558 } 00559 */ 00560 } 00561 00562 void UI_ClickClockInWords(uint16_t x,uint16_t y) 00563 { 00564 // exit view 00565 UI_Show(&uiMain); 00566 } 00567 00568 // 00569 // slideshow 00570 // 00571 void UI_ShowSlideshowFade(uint8_t from,uint8_t to) 00572 { 00573 if (from<to) 00574 { 00575 while (from<to) 00576 { 00577 uiLcd.SetTransparency(0,from); 00578 00579 wait_ms(30); 00580 00581 if ((to-from)<SLIDESHOW_FADE_STEP) 00582 break; 00583 00584 from+=SLIDESHOW_FADE_STEP; 00585 } 00586 } 00587 else 00588 { 00589 while (from>to) 00590 { 00591 uiLcd.SetTransparency(0,from); 00592 00593 wait_ms(30); 00594 00595 if ((from-to)<SLIDESHOW_FADE_STEP) 00596 break; 00597 00598 from-=SLIDESHOW_FADE_STEP; 00599 } 00600 } 00601 00602 uiLcd.SetTransparency(0,to); 00603 } 00604 00605 void UI_ShowSlideshow(bool initial) 00606 { 00607 char buffer[100]; 00608 struct tm *tmStruct; 00609 00610 RTC_Get(&tmStruct); 00611 00612 if (((tmStruct->tm_sec / SLIDESHOW_TIMEOUT)!=lastSlideshowTick) || (initial==true)) 00613 { 00614 lastSlideshowTick=(tmStruct->tm_sec / SLIDESHOW_TIMEOUT); 00615 00616 // fill background with transparent color 00617 uiLcd.SetTextColor(0x00000000); 00618 uiLcd.FillRect(0,0,uiLcd.GetXSize(),uiLcd.GetYSize()); 00619 00620 // show clock 00621 if (tmStruct->tm_hour>=10) 00622 snprintf(buffer,sizeof(buffer),"%u %u : %u %u",tmStruct->tm_hour / 10,tmStruct->tm_hour % 10,tmStruct->tm_min / 10,tmStruct->tm_min % 10); 00623 else 00624 snprintf(buffer,sizeof(buffer),"%u : %u %u",tmStruct->tm_hour,tmStruct->tm_min / 10,tmStruct->tm_min % 10); 00625 FontX_DisplayStringAt(30,180,uiLcd.GetXSize(),buffer,ALIGN_RIGHT,SLIDESHOW_FONT,SLIDESHOW_COLOR_FG,0x00000001); 00626 00627 // hide picture layer 00628 if (initial==true) 00629 uiLcd.SetTransparency(0,0); 00630 else 00631 UI_ShowSlideshowFade(SLIDESHOW_TRANSPARENCY,0); 00632 uiLcd.SelectLayer(0); 00633 // clear old picture 00634 uiLcd.Clear(LCD_COLOR_BLACK); 00635 00636 // load picture 00637 SD_ShowRandomPicture(); 00638 00639 // show new picture 00640 if (initial==true) 00641 uiLcd.SetLayerVisible(0,ENABLE); 00642 UI_ShowSlideshowFade(0,SLIDESHOW_TRANSPARENCY); 00643 uiLcd.SelectLayer(1); 00644 } 00645 } 00646 00647 void UI_ClickSlideshow(uint16_t x,uint16_t y) 00648 { 00649 // exit view 00650 uiLcd.SetLayerVisible(0,DISABLE); 00651 UI_Show(&uiMain); 00652 } 00653 00654 // 00655 // value adjust 00656 // 00657 void UI_ShowValueAdjust(bool initial) 00658 { 00659 char buffer[20]; 00660 00661 if (initial==true) 00662 { 00663 // fill background 00664 UI_ShowClearClientRect(); 00665 00666 if (uiCurrent->data.valueAdjust.count==10) 00667 { 00668 UI_ShowDrawButton(60+(0*(BUTTON_WIDTH+15)),40,BUTTON_WIDTH,BUTTON_HEIGHT,"+"); 00669 UI_ShowDrawButton(205+(0*(BUTTON_WIDTH+15)),40,BUTTON_WIDTH,BUTTON_HEIGHT,"+"); 00670 UI_ShowDrawButton(205+(1*(BUTTON_WIDTH+15)),40,BUTTON_WIDTH,BUTTON_HEIGHT,"+"); 00671 00672 if (uiCurrent->data.valueAdjust.isTime==true) 00673 UI_ShowDisplayTextCenter(132,92,BUTTON_WIDTH,BUTTON_HEIGHT,":",CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00674 00675 UI_ShowDrawButton(60+(0*(BUTTON_WIDTH+15)),140,BUTTON_WIDTH,BUTTON_HEIGHT,"-"); 00676 UI_ShowDrawButton(205+(0*(BUTTON_WIDTH+15)),140,BUTTON_WIDTH,BUTTON_HEIGHT,"-"); 00677 UI_ShowDrawButton(205+(1*(BUTTON_WIDTH+15)),140,BUTTON_WIDTH,BUTTON_HEIGHT,"-"); 00678 } 00679 else if (uiCurrent->data.valueAdjust.count==4) 00680 { 00681 UI_ShowDrawButton(17+(0*(BUTTON_WIDTH+15)),40,BUTTON_WIDTH,BUTTON_HEIGHT,"+"); 00682 UI_ShowDrawButton(17+(1*(BUTTON_WIDTH+15)),40,BUTTON_WIDTH,BUTTON_HEIGHT,"+"); 00683 UI_ShowDrawButton(17+(2*(BUTTON_WIDTH+15)),40,BUTTON_WIDTH,BUTTON_HEIGHT,"+"); 00684 UI_ShowDrawButton(17+(3*(BUTTON_WIDTH+15)),40,BUTTON_WIDTH,BUTTON_HEIGHT,"+"); 00685 00686 UI_ShowDrawButton(17+(0*(BUTTON_WIDTH+15)),205,BUTTON_WIDTH,BUTTON_HEIGHT,"-"); 00687 UI_ShowDrawButton(17+(1*(BUTTON_WIDTH+15)),205,BUTTON_WIDTH,BUTTON_HEIGHT,"-"); 00688 UI_ShowDrawButton(17+(2*(BUTTON_WIDTH+15)),205,BUTTON_WIDTH,BUTTON_HEIGHT,"-"); 00689 UI_ShowDrawButton(17+(3*(BUTTON_WIDTH+15)),205,BUTTON_WIDTH,BUTTON_HEIGHT,"-"); 00690 } 00691 else if (uiCurrent->data.valueAdjust.count==3) 00692 { 00693 if (uiCurrent->data.valueAdjust.isTime==true) 00694 { 00695 UI_ShowDrawButton(60+(0*(BUTTON_WIDTH+15)),40,BUTTON_WIDTH,BUTTON_HEIGHT,"+"); 00696 UI_ShowDrawButton(205+(0*(BUTTON_WIDTH+15)),40,BUTTON_WIDTH,BUTTON_HEIGHT,"+"); 00697 UI_ShowDrawButton(205+(1*(BUTTON_WIDTH+15)),40,BUTTON_WIDTH,BUTTON_HEIGHT,"+"); 00698 00699 UI_ShowDisplayTextCenter(132,125,BUTTON_WIDTH,BUTTON_HEIGHT,":",CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00700 00701 UI_ShowDrawButton(60+(0*(BUTTON_WIDTH+15)),205,BUTTON_WIDTH,BUTTON_HEIGHT,"-"); 00702 UI_ShowDrawButton(205+(0*(BUTTON_WIDTH+15)),205,BUTTON_WIDTH,BUTTON_HEIGHT,"-"); 00703 UI_ShowDrawButton(205+(1*(BUTTON_WIDTH+15)),205,BUTTON_WIDTH,BUTTON_HEIGHT,"-"); 00704 } 00705 else 00706 { 00707 UI_ShowDrawButton(74+(0*(BUTTON_WIDTH+15)),40,BUTTON_WIDTH,BUTTON_HEIGHT,"+"); 00708 UI_ShowDrawButton(74+(1*(BUTTON_WIDTH+15)),40,BUTTON_WIDTH,BUTTON_HEIGHT,"+"); 00709 UI_ShowDrawButton(74+(2*(BUTTON_WIDTH+15)),40,BUTTON_WIDTH,BUTTON_HEIGHT,"+"); 00710 00711 UI_ShowDisplayTextCenter(130,125,BUTTON_WIDTH,BUTTON_HEIGHT,".",CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00712 UI_ShowDisplayTextCenter(246,125,BUTTON_WIDTH,BUTTON_HEIGHT,".",CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00713 00714 UI_ShowDrawButton(74+(0*(BUTTON_WIDTH+15)),205,BUTTON_WIDTH,BUTTON_HEIGHT,"-"); 00715 UI_ShowDrawButton(74+(1*(BUTTON_WIDTH+15)),205,BUTTON_WIDTH,BUTTON_HEIGHT,"-"); 00716 UI_ShowDrawButton(74+(2*(BUTTON_WIDTH+15)),205,BUTTON_WIDTH,BUTTON_HEIGHT,"-"); 00717 } 00718 } 00719 } 00720 00721 if (uiCurrent->data.valueAdjust.count==10) 00722 { 00723 snprintf(buffer,sizeof(buffer)," % 2u ",uiCurrent->data.valueAdjust.values[0]); 00724 UI_ShowDisplayTextCenter(60+(0*(BUTTON_WIDTH+15)),92,BUTTON_WIDTH,BUTTON_HEIGHT,buffer,CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00725 00726 snprintf(buffer,sizeof(buffer)," %u ",uiCurrent->data.valueAdjust.values[1]); 00727 UI_ShowDisplayTextCenter(205+(0*(BUTTON_WIDTH+15)),92,BUTTON_WIDTH,BUTTON_HEIGHT,buffer,CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00728 00729 snprintf(buffer,sizeof(buffer)," %u ",uiCurrent->data.valueAdjust.values[2]); 00730 UI_ShowDisplayTextCenter(205+(1*(BUTTON_WIDTH+15)),92,BUTTON_WIDTH,BUTTON_HEIGHT,buffer,CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00731 00732 if (uiCurrent->data.valueAdjust.values[3]==1) 00733 UI_ShowDrawButton(17+(0*(BUTTON_SMALL_WIDTH+15)),215,BUTTON_SMALL_WIDTH,BUTTON_SMALL_HEIGHT,"Mo"); 00734 else 00735 UI_ShowDrawButtonEx(17+(0*(BUTTON_SMALL_WIDTH+15)),215,BUTTON_SMALL_WIDTH,BUTTON_SMALL_HEIGHT,"Mo",false); 00736 00737 if (uiCurrent->data.valueAdjust.values[4]==1) 00738 UI_ShowDrawButton(17+(1*(BUTTON_SMALL_WIDTH+15)),215,BUTTON_SMALL_WIDTH,BUTTON_SMALL_HEIGHT,"Di"); 00739 else 00740 UI_ShowDrawButtonEx(17+(1*(BUTTON_SMALL_WIDTH+15)),215,BUTTON_SMALL_WIDTH,BUTTON_SMALL_HEIGHT,"Di",false); 00741 00742 if (uiCurrent->data.valueAdjust.values[5]==1) 00743 UI_ShowDrawButton(17+(2*(BUTTON_SMALL_WIDTH+15)),215,BUTTON_SMALL_WIDTH,BUTTON_SMALL_HEIGHT,"Mi"); 00744 else 00745 UI_ShowDrawButtonEx(17+(2*(BUTTON_SMALL_WIDTH+15)),215,BUTTON_SMALL_WIDTH,BUTTON_SMALL_HEIGHT,"Mi",false); 00746 00747 if (uiCurrent->data.valueAdjust.values[6]==1) 00748 UI_ShowDrawButton(17+(3*(BUTTON_SMALL_WIDTH+15)),215,BUTTON_SMALL_WIDTH,BUTTON_SMALL_HEIGHT,"Do"); 00749 else 00750 UI_ShowDrawButtonEx(17+(3*(BUTTON_SMALL_WIDTH+15)),215,BUTTON_SMALL_WIDTH,BUTTON_SMALL_HEIGHT,"Do",false); 00751 00752 if (uiCurrent->data.valueAdjust.values[7]==1) 00753 UI_ShowDrawButton(17+(4*(BUTTON_SMALL_WIDTH+15)),215,BUTTON_SMALL_WIDTH,BUTTON_SMALL_HEIGHT,"Fr"); 00754 else 00755 UI_ShowDrawButtonEx(17+(4*(BUTTON_SMALL_WIDTH+15)),215,BUTTON_SMALL_WIDTH,BUTTON_SMALL_HEIGHT,"Fr",false); 00756 00757 if (uiCurrent->data.valueAdjust.values[8]==1) 00758 UI_ShowDrawButton(17+(5*(BUTTON_SMALL_WIDTH+15)),215,BUTTON_SMALL_WIDTH,BUTTON_SMALL_HEIGHT,"Sa"); 00759 else 00760 UI_ShowDrawButtonEx(17+(5*(BUTTON_SMALL_WIDTH+15)),215,BUTTON_SMALL_WIDTH,BUTTON_SMALL_HEIGHT,"Sa",false); 00761 00762 if (uiCurrent->data.valueAdjust.values[9]==1) 00763 UI_ShowDrawButton(17+(6*(BUTTON_SMALL_WIDTH+15)),215,BUTTON_SMALL_WIDTH,BUTTON_SMALL_HEIGHT,"So"); 00764 else 00765 UI_ShowDrawButtonEx(17+(6*(BUTTON_SMALL_WIDTH+15)),215,BUTTON_SMALL_WIDTH,BUTTON_SMALL_HEIGHT,"So",false); 00766 } 00767 else if (uiCurrent->data.valueAdjust.count==4) 00768 { 00769 snprintf(buffer,sizeof(buffer)," 0x%02X ",uiCurrent->data.valueAdjust.values[0]); 00770 UI_ShowDisplayTextCenter(17+(0*(BUTTON_WIDTH+15)),125,BUTTON_WIDTH,BUTTON_HEIGHT,buffer,CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00771 00772 snprintf(buffer,sizeof(buffer)," 0x%02X ",uiCurrent->data.valueAdjust.values[1]); 00773 UI_ShowDisplayTextCenter(17+(1*(BUTTON_WIDTH+15)),125,BUTTON_WIDTH,BUTTON_HEIGHT,buffer,CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00774 00775 snprintf(buffer,sizeof(buffer)," 0x%02X ",uiCurrent->data.valueAdjust.values[2]); 00776 UI_ShowDisplayTextCenter(17+(2*(BUTTON_WIDTH+15)),125,BUTTON_WIDTH,BUTTON_HEIGHT,buffer,CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00777 00778 snprintf(buffer,sizeof(buffer)," 0x%02X ",uiCurrent->data.valueAdjust.values[3]); 00779 UI_ShowDisplayTextCenter(17+(3*(BUTTON_WIDTH+15)),125,BUTTON_WIDTH,BUTTON_HEIGHT,buffer,CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00780 } 00781 else if (uiCurrent->data.valueAdjust.count==3) 00782 { 00783 if (uiCurrent->data.valueAdjust.isTime==true) 00784 { 00785 snprintf(buffer,sizeof(buffer)," % 2u ",uiCurrent->data.valueAdjust.values[0]); 00786 UI_ShowDisplayTextCenter(60+(0*(BUTTON_WIDTH+15)),125,BUTTON_WIDTH,BUTTON_HEIGHT,buffer,CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00787 00788 snprintf(buffer,sizeof(buffer)," % 2u ",uiCurrent->data.valueAdjust.values[1]); 00789 UI_ShowDisplayTextCenter(205+(0*(BUTTON_WIDTH+15)),125,BUTTON_WIDTH,BUTTON_HEIGHT,buffer,CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00790 00791 snprintf(buffer,sizeof(buffer)," % 2u ",uiCurrent->data.valueAdjust.values[2]); 00792 UI_ShowDisplayTextCenter(205+(1*(BUTTON_WIDTH+15)),125,BUTTON_WIDTH,BUTTON_HEIGHT,buffer,CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00793 } 00794 else 00795 { 00796 snprintf(buffer,sizeof(buffer)," % 2u ",uiCurrent->data.valueAdjust.values[0]); 00797 UI_ShowDisplayTextCenter(74+(0*(BUTTON_WIDTH+15)),125,BUTTON_WIDTH,BUTTON_HEIGHT,buffer,CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00798 00799 snprintf(buffer,sizeof(buffer)," % 2u ",uiCurrent->data.valueAdjust.values[1]); 00800 UI_ShowDisplayTextCenter(74+(1*(BUTTON_WIDTH+15)),125,BUTTON_WIDTH,BUTTON_HEIGHT,buffer,CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00801 00802 snprintf(buffer,sizeof(buffer)," %02u ",uiCurrent->data.valueAdjust.values[2]); 00803 UI_ShowDisplayTextCenter(74+(2*(BUTTON_WIDTH+15)),125,BUTTON_WIDTH,BUTTON_HEIGHT,buffer,CLIENT_FONT,CLIENT_COLOR_FG,CLIENT_COLOR_BG); 00804 } 00805 } 00806 } 00807 00808 void UI_ClickValueAdjust(uint16_t x,uint16_t y) 00809 { 00810 int32_t index; 00811 00812 // detect at which button was clicked 00813 index=-1; 00814 00815 if (uiCurrent->data.valueAdjust.count==10) 00816 { 00817 if ((y>=40) && (y<(40+BUTTON_HEIGHT))) 00818 { 00819 if ((x>=(60+(0*(BUTTON_WIDTH+15)))) && (x<(60+(0*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00820 index=0; 00821 else if ((x>=(205+(0*(BUTTON_WIDTH+15)))) && (x<(205+(0*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00822 index=1; 00823 else if ((x>=(205+(1*(BUTTON_WIDTH+15)))) && (x<(205+(1*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00824 index=2; 00825 } 00826 else if ((y>=140) && (y<(140+BUTTON_HEIGHT))) 00827 { 00828 if ((x>=(60+(0*(BUTTON_WIDTH+15)))) && (x<(60+(0*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00829 index=3; 00830 else if ((x>=(205+(0*(BUTTON_WIDTH+15)))) && (x<(205+(0*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00831 index=4; 00832 else if ((x>=(205+(1*(BUTTON_WIDTH+15)))) && (x<(205+(1*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00833 index=5; 00834 } 00835 else if ((y>=215) && (y<(215+BUTTON_SMALL_HEIGHT))) 00836 { 00837 if ((x>=(17+(0*(BUTTON_SMALL_WIDTH+15)))) && (x<(17+(0*(BUTTON_SMALL_WIDTH+15))+BUTTON_SMALL_WIDTH))) 00838 index=6; 00839 else if ((x>=(17+(1*(BUTTON_SMALL_WIDTH+15)))) && (x<(17+(1*(BUTTON_SMALL_WIDTH+15))+BUTTON_SMALL_WIDTH))) 00840 index=7; 00841 else if ((x>=(17+(2*(BUTTON_SMALL_WIDTH+15)))) && (x<(17+(2*(BUTTON_SMALL_WIDTH+15))+BUTTON_SMALL_WIDTH))) 00842 index=8; 00843 else if ((x>=(17+(3*(BUTTON_SMALL_WIDTH+15)))) && (x<(17+(3*(BUTTON_SMALL_WIDTH+15))+BUTTON_SMALL_WIDTH))) 00844 index=9; 00845 else if ((x>=(17+(4*(BUTTON_SMALL_WIDTH+15)))) && (x<(17+(4*(BUTTON_SMALL_WIDTH+15))+BUTTON_SMALL_WIDTH))) 00846 index=10; 00847 else if ((x>=(17+(5*(BUTTON_SMALL_WIDTH+15)))) && (x<(17+(5*(BUTTON_SMALL_WIDTH+15))+BUTTON_SMALL_WIDTH))) 00848 index=11; 00849 else if ((x>=(17+(6*(BUTTON_SMALL_WIDTH+15)))) && (x<(17+(6*(BUTTON_SMALL_WIDTH+15))+BUTTON_SMALL_WIDTH))) 00850 index=12; 00851 } 00852 } 00853 else if (uiCurrent->data.valueAdjust.count==4) 00854 { 00855 if ((y>=40) && (y<(40+BUTTON_HEIGHT))) 00856 { 00857 if ((x>=(17+(0*(BUTTON_WIDTH+15)))) && (x<(17+(0*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00858 index=0; 00859 else if ((x>=(17+(1*(BUTTON_WIDTH+15)))) && (x<(17+(1*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00860 index=1; 00861 else if ((x>=(17+(2*(BUTTON_WIDTH+15)))) && (x<(17+(2*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00862 index=2; 00863 else if ((x>=(17+(3*(BUTTON_WIDTH+15)))) && (x<(17+(3*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00864 index=3; 00865 } 00866 else if ((y>=205) && (y<(205+BUTTON_HEIGHT))) 00867 { 00868 if ((x>=(17+(0*(BUTTON_WIDTH+15)))) && (x<(17+(0*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00869 index=4; 00870 else if ((x>=(17+(1*(BUTTON_WIDTH+15)))) && (x<(17+(1*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00871 index=5; 00872 else if ((x>=(17+(2*(BUTTON_WIDTH+15)))) && (x<(17+(2*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00873 index=6; 00874 else if ((x>=(17+(3*(BUTTON_WIDTH+15)))) && (x<(17+(3*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00875 index=7; 00876 } 00877 } 00878 else if (uiCurrent->data.valueAdjust.count==3) 00879 { 00880 if (uiCurrent->data.valueAdjust.isTime==true) 00881 { 00882 if ((y>=40) && (y<(40+BUTTON_HEIGHT))) 00883 { 00884 if ((x>=(60+(0*(BUTTON_WIDTH+15)))) && (x<(60+(0*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00885 index=0; 00886 else if ((x>=(205+(0*(BUTTON_WIDTH+15)))) && (x<(205+(0*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00887 index=1; 00888 else if ((x>=(205+(1*(BUTTON_WIDTH+15)))) && (x<(205+(1*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00889 index=2; 00890 } 00891 else if ((y>=205) && (y<(205+BUTTON_HEIGHT))) 00892 { 00893 if ((x>=(60+(0*(BUTTON_WIDTH+15)))) && (x<(60+(0*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00894 index=3; 00895 else if ((x>=(205+(0*(BUTTON_WIDTH+15)))) && (x<(205+(0*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00896 index=4; 00897 else if ((x>=(205+(1*(BUTTON_WIDTH+15)))) && (x<(205+(1*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00898 index=5; 00899 } 00900 } 00901 else 00902 { 00903 if ((y>=40) && (y<(40+BUTTON_HEIGHT))) 00904 { 00905 if ((x>=(74+(0*(BUTTON_WIDTH+15)))) && (x<(74+(0*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00906 index=0; 00907 else if ((x>=(74+(1*(BUTTON_WIDTH+15)))) && (x<(74+(1*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00908 index=1; 00909 else if ((x>=(74+(2*(BUTTON_WIDTH+15)))) && (x<(74+(2*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00910 index=2; 00911 } 00912 else if ((y>=205) && (y<(205+BUTTON_HEIGHT))) 00913 { 00914 if ((x>=(74+(0*(BUTTON_WIDTH+15)))) && (x<(74+(0*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00915 index=3; 00916 else if ((x>=(74+(1*(BUTTON_WIDTH+15)))) && (x<(74+(1*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00917 index=4; 00918 else if ((x>=(74+(2*(BUTTON_WIDTH+15)))) && (x<(74+(2*(BUTTON_WIDTH+15))+BUTTON_WIDTH))) 00919 index=5; 00920 } 00921 } 00922 } 00923 00924 if (index!=-1) 00925 uiCurrent->handler(UR_CLICK,index,uiCurrent); 00926 } 00927 00928 // 00929 // common 00930 // 00931 void UI_Init(void) 00932 { 00933 uiCurrent=NULL; 00934 00935 uiLastTouchX=0; 00936 uiLastTouchY=0; 00937 00938 uiLcd.Init(); 00939 // layer 0: slideshow pictures 00940 // layer 1: all other ui 00941 uiLcd.SelectLayer(1); 00942 uiLcd.Clear(COLOR_BG); 00943 00944 if (uiTs.Init(uiLcd.GetXSize(),uiLcd.GetYSize())==TS_OK) 00945 DPrintf("UI_Init: Size: %ux%u.\r\n",uiLcd.GetXSize(),uiLcd.GetYSize()); 00946 else 00947 DPrintf("UI_Init: Can't init touch screen.\r\n"); 00948 00949 // setup ui structs 00950 uiClock.flags=UI_FLAG_TYPE_CLOCK; 00951 uiClock.handler=NULL; 00952 00953 uiClockInWords.flags=UI_FLAG_TYPE_CLOCK_IN_WORDS; 00954 uiClockInWords.handler=NULL; 00955 00956 uiColorTest.flags=UI_FLAG_TYPE_VALUE_ADJUST; 00957 uiColorTest.handler=UI_ColorTestHandler; 00958 uiColorTest.data.valueAdjust.count=4; 00959 uiColorTest.data.valueAdjust.values[0]=0x80; 00960 uiColorTest.data.valueAdjust.values[1]=0x80; 00961 uiColorTest.data.valueAdjust.values[2]=0x80; 00962 uiColorTest.data.valueAdjust.values[3]=0x80; 00963 00964 uiMain.flags=UI_FLAG_TYPE_BOX_LIST; 00965 uiMain.handler=UI_MainHandler; 00966 uiMain.data.boxList.items=uiMainItems; 00967 uiMain.data.boxList.count=COUNT_OF(uiMainItems); 00968 00969 uiSlideshow.flags=UI_FLAG_TYPE_SLIDESHOW; 00970 00971 UI_Show(&uiMain); 00972 } 00973 00974 void UI_ShowChrome(bool initial) 00975 { 00976 char buffer[100]; 00977 struct tm *tmStruct; 00978 00979 if (initial==true) 00980 { 00981 // fill background 00982 uiLcd.SetTextColor(HEADER_COLOR_BG); 00983 uiLcd.FillRect(0,0,uiLcd.GetXSize(),HEADER_HEIGHT); 00984 00985 // draw line 00986 uiLcd.SetTextColor(HEADER_LINE); 00987 uiLcd.DrawHLine(10,HEADER_HEIGHT-1,uiLcd.GetXSize()-20); 00988 00989 if ((uiCurrent->flags & UI_FLAG_HAS_BACK_BUTTON)!=0) 00990 UI_DrawBitmapWithAlpha(5,1,ic_navigate_before_white_24dp_1x); 00991 } 00992 00993 // show clock 00994 RTC_Get(&tmStruct); 00995 snprintf(buffer,sizeof(buffer)," %u:%02u ",tmStruct->tm_hour,tmStruct->tm_min); 00996 UI_ShowDisplayTextCenter(0,2,uiLcd.GetXSize(),-1,buffer,HEADER_FONT,HEADER_COLOR_FG,HEADER_COLOR_BG); 00997 00998 // show next alarm 00999 //XXX 01000 } 01001 01002 void UI_Update(bool initial) 01003 { 01004 uint8_t typeFlag; 01005 01006 if ((uiCurrent->flags & UI_FLAG_NEEDS_CHROME)!=0) 01007 UI_ShowChrome(initial); 01008 01009 typeFlag=uiCurrent->flags & ~(UI_FLAG_NEEDS_CHROME | UI_FLAG_HAS_BACK_BUTTON); 01010 if ((typeFlag & UI_FLAG_TYPE_BOX_LIST)!=0) 01011 UI_ShowBoxList(initial); 01012 else if ((typeFlag & UI_FLAG_TYPE_CLOCK)!=0) 01013 UI_ShowClock(initial); 01014 else if ((typeFlag & UI_FLAG_TYPE_CLOCK_IN_WORDS)!=0) 01015 UI_ShowClockInWords(initial); 01016 else if ((typeFlag & UI_FLAG_TYPE_SLIDESHOW)!=0) 01017 UI_ShowSlideshow(initial); 01018 else if ((typeFlag & UI_FLAG_TYPE_VALUE_ADJUST)!=0) 01019 UI_ShowValueAdjust(initial); 01020 } 01021 01022 void UI_Show(UI_STRUCT *ui) 01023 { 01024 DPrintf_("UI_Show: 0x%X.\r\n",ui->flags); 01025 01026 uiCurrent=ui; 01027 01028 if (uiCurrent->handler!=NULL) 01029 uiCurrent->handler(UR_SHOW,0,uiCurrent); 01030 01031 UI_Update(true); 01032 } 01033 01034 void UI_Click(uint16_t x,uint16_t y) 01035 { 01036 uint8_t typeFlag; 01037 01038 DPrintf_("UI_Click: %u x %u.\r\n",x,y); 01039 01040 if ((uiCurrent->flags & UI_FLAG_HAS_BACK_BUTTON)!=0) 01041 { 01042 if ((y<40) && (x<40)) 01043 { 01044 if (uiCurrent->handler!=NULL) 01045 { 01046 uiCurrent->handler(UR_CLICK,-1,uiCurrent); 01047 return; 01048 } 01049 } 01050 } 01051 01052 typeFlag=uiCurrent->flags & ~(UI_FLAG_NEEDS_CHROME | UI_FLAG_HAS_BACK_BUTTON); 01053 if ((typeFlag & UI_FLAG_TYPE_BOX_LIST)!=0) 01054 UI_ClickBoxList(x,y); 01055 else if ((typeFlag & UI_FLAG_TYPE_CLOCK)!=0) 01056 UI_ClickClock(x,y); 01057 else if ((typeFlag & UI_FLAG_TYPE_CLOCK_IN_WORDS)!=0) 01058 UI_ClickClockInWords(x,y); 01059 else if ((typeFlag & UI_FLAG_TYPE_SLIDESHOW)!=0) 01060 UI_ClickSlideshow(x,y); 01061 else if ((typeFlag & UI_FLAG_TYPE_VALUE_ADJUST)!=0) 01062 UI_ClickValueAdjust(x,y); 01063 } 01064 01065 void UI_Poll(void) 01066 { 01067 TS_StateTypeDef tsState; 01068 01069 uiTs.GetState(&tsState); 01070 if (tsState.touchDetected>0) 01071 { 01072 if ( (ABS(uiLastTouchX-tsState.touchX[0])>4) 01073 || 01074 (ABS(uiLastTouchY-tsState.touchY[0])>4) 01075 ) 01076 { 01077 DPrintf_("UI_Poll: #%u - %ux%u.\r\n",tsState.touchDetected,tsState.touchX[0],tsState.touchY[0]); 01078 01079 uiLastTouchX=tsState.touchX[0]; 01080 uiLastTouchY=tsState.touchY[0]; 01081 01082 if (uiCurrent!=NULL) 01083 UI_Click(tsState.touchX[0],tsState.touchY[0]); 01084 } 01085 } 01086 else 01087 { 01088 uiLastTouchX=0; 01089 uiLastTouchY=0; 01090 } 01091 01092 if (uiCurrent!=NULL) 01093 { 01094 if (uiCurrent->handler!=NULL) 01095 uiCurrent->handler(UR_TIMER,0,uiCurrent); 01096 01097 UI_Update(false); 01098 } 01099 }
Generated on Tue Jul 12 2022 21:40:04 by
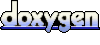