Wakeup Light with touch user interface, anti-aliased Font, SD card access and RTC usage on STM32F746NG-DISCO board
Dependencies: BSP_DISCO_F746NG_patch_fixed LCD_DISCO_F746NG TS_DISCO_F746NG FATFileSystem TinyJpgDec_interwork mbed-src
LED.cpp
00001 #include "WakeupLight.h" 00002 00003 #define LED_PERIOD 10 00004 00005 PwmOut ledBlue(PB_15); 00006 PwmOut ledRed(PH_6); 00007 PwmOut ledGreen(PB_4); 00008 PwmOut ledWhite(PA_15); 00009 Ticker ledTicker; 00010 int32_t ledAnimationStep; 00011 LED_ANIMATION_ENUM ledAnimation; 00012 00013 void LED_SetColor(int32_t color) 00014 { 00015 DPrintfCore("LED_SetColor: 0x%08X.\r\n",color); 00016 00017 ledRed.write(1.0 * COLOR_RED(color) / 255.0); 00018 ledGreen.write(1.0 * COLOR_GREEN(color) / 255.0); 00019 ledBlue.write(1.0 * COLOR_BLUE(color) / 255.0); 00020 ledWhite.write(1.0 * COLOR_WHITE(color) / 255.0); 00021 } 00022 00023 void LED_Callback() 00024 { 00025 int32_t color; 00026 00027 DPrintfCore("LED_Callback: Animation: %u, Step: %u.\r\n",ledAnimation,ledAnimationStep); 00028 00029 if (ledAnimation==LAE_WAKEUP) 00030 { 00031 if (ledAnimationStep<255) 00032 { 00033 ledAnimationStep++; 00034 color=COLOR_CREATE((ledAnimationStep/2),ledAnimationStep,0,0); 00035 LED_SetColor(color); 00036 } 00037 else if (ledAnimationStep<(255+180)) 00038 { 00039 ledAnimationStep++; 00040 00041 color=COLOR_CREATE(128,255,0,(ledAnimationStep-255)); 00042 LED_SetColor(color); 00043 } 00044 } 00045 else if (ledAnimation==LAE_OFF) 00046 { 00047 double red; 00048 double green; 00049 double blue; 00050 double white; 00051 uint32_t redInt; 00052 uint32_t greenInt; 00053 uint32_t blueInt; 00054 uint32_t whiteInt; 00055 00056 red=ledRed.read(); 00057 if (red>0.0) 00058 red-=0.001; 00059 red=MAX(red,0.0); 00060 redInt=(int32_t)(red*255.0); 00061 00062 green=ledGreen.read(); 00063 if (green>0.0) 00064 green-=0.001; 00065 green=MAX(green,0.0); 00066 greenInt=(int32_t)(green*255.0); 00067 00068 blue=ledBlue.read(); 00069 if (blue>0) 00070 blue-=0.001; 00071 blue=MAX(blue,0.0); 00072 blueInt=(int32_t)(blue*255.0); 00073 00074 white=ledWhite.read(); 00075 if (white>0) 00076 white-=0.001; 00077 white=MAX(white,0.0); 00078 whiteInt=(int32_t)(white*255.0); 00079 00080 color=COLOR_CREATE(redInt,greenInt,blueInt,whiteInt); 00081 LED_SetColor(color); 00082 } 00083 } 00084 00085 void LED_StartAnimation(LED_ANIMATION_ENUM animation) 00086 { 00087 ledAnimationStep=0; 00088 ledAnimation=animation; 00089 } 00090 00091 void LED_Init(void) 00092 { 00093 ledRed.period_ms(LED_PERIOD); 00094 ledGreen.period_ms(LED_PERIOD); 00095 ledBlue.period_ms(LED_PERIOD); 00096 ledWhite.period_ms(LED_PERIOD); 00097 00098 LED_SetColor(COLOR_CREATE(0,0,0,0)); 00099 00100 LED_StartAnimation(LAE_NONE); 00101 00102 ledTicker.attach(&LED_Callback,0.05); 00103 }
Generated on Tue Jul 12 2022 21:40:04 by
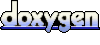