Wakeup Light with touch user interface, anti-aliased Font, SD card access and RTC usage on STM32F746NG-DISCO board
Dependencies: BSP_DISCO_F746NG_patch_fixed LCD_DISCO_F746NG TS_DISCO_F746NG FATFileSystem TinyJpgDec_interwork mbed-src
FontX.h
00001 #ifndef __FontX_h 00002 #define __FontX_h 00003 00004 #include "mbed.h" 00005 00006 #define uint_8 unsigned char 00007 #define int8 char 00008 #define int16 short 00009 #define int32 long 00010 00011 typedef struct 00012 { 00013 unsigned int16 Charwidth; 00014 unsigned int16 Charheight; 00015 unsigned int32 Offset; 00016 00017 } FONT_CHAR_INFO; 00018 00019 typedef struct 00020 { 00021 char StartCharacter; 00022 char EndCharacter; 00023 const FONT_CHAR_INFO *Descriptors; 00024 const unsigned int8 *Bitmaps; 00025 00026 } FONT_INFO; 00027 00028 #include "Calibri36pt.h" 00029 #include "TrebuchetMS270pt.h" 00030 #include "TrebuchetMS135pt.h" 00031 00032 typedef enum 00033 { 00034 ALIGN_CENTER, 00035 ALIGN_RIGHT, 00036 ALIGN_LEFT 00037 00038 } FONTX_ALIGNMENT_ENUM; 00039 00040 uint32_t FontX_GetLength(char *Text,const FONT_INFO *pFont); 00041 uint32_t FontX_GetHeight(const FONT_INFO *pFont); 00042 void FontX_DisplayStringAt(uint16_t Xpos,uint16_t Ypos,uint16_t width,char *Text,FONTX_ALIGNMENT_ENUM Alignment,const FONT_INFO *pFont,uint32_t colorText,uint32_t colorBack); 00043 uint32_t FontX_DisplayChar(uint16_t Xpos,uint16_t Ypos,char Ascii,const FONT_INFO *pFont,uint32_t colorText,uint32_t colorBack); 00044 00045 #endif 00046
Generated on Tue Jul 12 2022 21:40:03 by
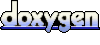