
embedded smtf7 based scope
Dependencies: BSP_DISCO_F746NG_patch_fixed mbed
Interface.h
00001 #ifndef __Interface_h 00002 #define __Interface_h 00003 00004 #pragma pack(1) 00005 00006 #define BUFFER_SIZE 1000 // number of samples per HT_DATA report 00007 00008 #define CHANNEL_COUNT 2 00009 #define HEADER_MAGIC 0x53636F70 // 'Scop' 00010 00011 typedef enum 00012 { 00013 HT_NONE, 00014 HT_DATA, 00015 HT_INFO_REQUEST, 00016 HT_INFO_RESPONSE, 00017 HT_CAPTURE_START, 00018 00019 } HEADER_TYPE_ENUM; 00020 00021 typedef struct 00022 { 00023 float values[CHANNEL_COUNT]; 00024 00025 } VALUES_STRUCT; 00026 00027 typedef struct 00028 { 00029 uint32_t magic; 00030 uint8_t type; 00031 uint32_t length; // length in bytes of following data 00032 00033 } HEADER_STRUCT; 00034 00035 typedef struct 00036 { 00037 uint8_t versionMajor; 00038 uint8_t versionMinor; 00039 char name[20]; 00040 00041 } INTERFACE_INFO_RESPONSE_STRUCT; 00042 00043 typedef enum 00044 { 00045 TM_NONE =0x00, 00046 TM_RAISING =0x01, 00047 TM_FALLING =0x02, 00048 TM_AUTO =0x04, 00049 00050 } TRIGGER_MODE_ENUM; 00051 00052 typedef struct 00053 { 00054 float triggerLevel; 00055 uint8_t triggerMode; 00056 uint16_t delay; // in usec 00057 00058 } INTERFACE_CAPTURE_START_STRUCT; 00059 00060 #pragma pack() 00061 00062 #endif
Generated on Mon Jul 18 2022 01:21:00 by
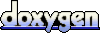