
json test
Embed:
(wiki syntax)
Show/hide line numbers
json_valueiterator.inl
00001 // Copyright 2007-2010 Baptiste Lepilleur and The JsonCpp Authors 00002 // Distributed under MIT license, or public domain if desired and 00003 // recognized in your jurisdiction. 00004 // See file LICENSE for detail or copy at http://jsoncpp.sourceforge.net/LICENSE 00005 00006 // included by json_value.cpp 00007 00008 namespace Json { 00009 00010 // ////////////////////////////////////////////////////////////////// 00011 // ////////////////////////////////////////////////////////////////// 00012 // ////////////////////////////////////////////////////////////////// 00013 // class ValueIteratorBase 00014 // ////////////////////////////////////////////////////////////////// 00015 // ////////////////////////////////////////////////////////////////// 00016 // ////////////////////////////////////////////////////////////////// 00017 00018 ValueIteratorBase::ValueIteratorBase() 00019 : current_(), isNull_(true) { 00020 } 00021 00022 ValueIteratorBase::ValueIteratorBase( 00023 const Value::ObjectValues::iterator& current) 00024 : current_(current), isNull_(false) {} 00025 00026 Value& ValueIteratorBase::deref() const { 00027 return current_->second; 00028 } 00029 00030 void ValueIteratorBase::increment() { 00031 ++current_; 00032 } 00033 00034 void ValueIteratorBase::decrement() { 00035 --current_; 00036 } 00037 00038 ValueIteratorBase::difference_type 00039 ValueIteratorBase::computeDistance(const SelfType& other) const { 00040 #ifdef JSON_USE_CPPTL_SMALLMAP 00041 return other.current_ - current_; 00042 #else 00043 // Iterator for null value are initialized using the default 00044 // constructor, which initialize current_ to the default 00045 // std::map::iterator. As begin() and end() are two instance 00046 // of the default std::map::iterator, they can not be compared. 00047 // To allow this, we handle this comparison specifically. 00048 if (isNull_ && other.isNull_) { 00049 return 0; 00050 } 00051 00052 // Usage of std::distance is not portable (does not compile with Sun Studio 12 00053 // RogueWave STL, 00054 // which is the one used by default). 00055 // Using a portable hand-made version for non random iterator instead: 00056 // return difference_type( std::distance( current_, other.current_ ) ); 00057 difference_type myDistance = 0; 00058 for (Value::ObjectValues::iterator it = current_; it != other.current_; 00059 ++it) { 00060 ++myDistance; 00061 } 00062 return myDistance; 00063 #endif 00064 } 00065 00066 bool ValueIteratorBase::isEqual(const SelfType& other) const { 00067 if (isNull_) { 00068 return other.isNull_; 00069 } 00070 return current_ == other.current_; 00071 } 00072 00073 void ValueIteratorBase::copy(const SelfType& other) { 00074 current_ = other.current_; 00075 isNull_ = other.isNull_; 00076 } 00077 00078 Value ValueIteratorBase::key() const { 00079 const Value::CZString czstring = (*current_).first; 00080 if (czstring.data()) { 00081 if (czstring.isStaticString()) 00082 return Value(StaticString(czstring.data())); 00083 return Value(czstring.data(), czstring.data() + czstring.length()); 00084 } 00085 return Value(czstring.index()); 00086 } 00087 00088 UInt ValueIteratorBase::index() const { 00089 const Value::CZString czstring = (*current_).first; 00090 if (!czstring.data()) 00091 return czstring.index(); 00092 return Value::UInt(-1); 00093 } 00094 00095 JSONCPP_STRING ValueIteratorBase::name() const { 00096 char const* keey; 00097 char const* end; 00098 keey = memberName(&end); 00099 if (!keey) return JSONCPP_STRING(); 00100 return JSONCPP_STRING(keey, end); 00101 } 00102 00103 char const* ValueIteratorBase::memberName() const { 00104 const char* cname = (*current_).first.data(); 00105 return cname ? cname : ""; 00106 } 00107 00108 char const* ValueIteratorBase::memberName(char const** end) const { 00109 const char* cname = (*current_).first.data(); 00110 if (!cname) { 00111 *end = NULL; 00112 return NULL; 00113 } 00114 *end = cname + (*current_).first.length(); 00115 return cname; 00116 } 00117 00118 // ////////////////////////////////////////////////////////////////// 00119 // ////////////////////////////////////////////////////////////////// 00120 // ////////////////////////////////////////////////////////////////// 00121 // class ValueConstIterator 00122 // ////////////////////////////////////////////////////////////////// 00123 // ////////////////////////////////////////////////////////////////// 00124 // ////////////////////////////////////////////////////////////////// 00125 00126 ValueConstIterator::ValueConstIterator() {} 00127 00128 ValueConstIterator::ValueConstIterator( 00129 const Value::ObjectValues::iterator& current) 00130 : ValueIteratorBase(current) {} 00131 00132 ValueConstIterator::ValueConstIterator(ValueIterator const& other) 00133 : ValueIteratorBase(other) {} 00134 00135 ValueConstIterator& ValueConstIterator:: 00136 operator=(const ValueIteratorBase& other) { 00137 copy(other); 00138 return *this; 00139 } 00140 00141 // ////////////////////////////////////////////////////////////////// 00142 // ////////////////////////////////////////////////////////////////// 00143 // ////////////////////////////////////////////////////////////////// 00144 // class ValueIterator 00145 // ////////////////////////////////////////////////////////////////// 00146 // ////////////////////////////////////////////////////////////////// 00147 // ////////////////////////////////////////////////////////////////// 00148 00149 ValueIterator::ValueIterator() {} 00150 00151 ValueIterator::ValueIterator(const Value::ObjectValues::iterator& current) 00152 : ValueIteratorBase(current) {} 00153 00154 ValueIterator::ValueIterator(const ValueConstIterator& other) 00155 : ValueIteratorBase(other) { 00156 throwRuntimeError("ConstIterator to Iterator should never be allowed."); 00157 } 00158 00159 ValueIterator::ValueIterator(const ValueIterator& other) 00160 : ValueIteratorBase(other) {} 00161 00162 ValueIterator& ValueIterator::operator=(const SelfType& other) { 00163 copy(other); 00164 return *this; 00165 } 00166 00167 } // namespace Json
Generated on Tue Jul 12 2022 21:24:54 by
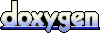