
json test
Embed:
(wiki syntax)
Show/hide line numbers
allocator.h
00001 // Copyright 2007-2010 Baptiste Lepilleur and The JsonCpp Authors 00002 // Distributed under MIT license, or public domain if desired and 00003 // recognized in your jurisdiction. 00004 // See file LICENSE for detail or copy at http://jsoncpp.sourceforge.net/LICENSE 00005 00006 #ifndef CPPTL_JSON_ALLOCATOR_H_INCLUDED 00007 #define CPPTL_JSON_ALLOCATOR_H_INCLUDED 00008 00009 #include <cstring> 00010 #include <memory> 00011 00012 #pragma pack(push, 8) 00013 00014 namespace Json { 00015 template<typename T> 00016 class SecureAllocator { 00017 public: 00018 // Type definitions 00019 using value_type = T; 00020 using pointer = T*; 00021 using const_pointer = const T*; 00022 using reference = T&; 00023 using const_reference = const T&; 00024 using size_type = std::size_t; 00025 using difference_type = std::ptrdiff_t; 00026 00027 /** 00028 * Allocate memory for N items using the standard allocator. 00029 */ 00030 pointer allocate(size_type n) { 00031 // allocate using "global operator new" 00032 return static_cast<pointer>(::operator new(n * sizeof(T))); 00033 } 00034 00035 /** 00036 * Release memory which was allocated for N items at pointer P. 00037 * 00038 * The memory block is filled with zeroes before being released. 00039 * The pointer argument is tagged as "volatile" to prevent the 00040 * compiler optimizing out this critical step. 00041 */ 00042 void deallocate(volatile pointer p, size_type n) { 00043 std::memset(p, 0, n * sizeof(T)); 00044 // free using "global operator delete" 00045 ::operator delete(p); 00046 } 00047 00048 /** 00049 * Construct an item in-place at pointer P. 00050 */ 00051 template<typename... Args> 00052 void construct(pointer p, Args&&... args) { 00053 // construct using "placement new" and "perfect forwarding" 00054 ::new (static_cast<void*>(p)) T(std::forward<Args>(args)...); 00055 } 00056 00057 size_type max_size() const { 00058 return size_t(-1) / sizeof(T); 00059 } 00060 00061 pointer address( reference x ) const { 00062 return std::addressof(x); 00063 } 00064 00065 const_pointer address( const_reference x ) const { 00066 return std::addressof(x); 00067 } 00068 00069 /** 00070 * Destroy an item in-place at pointer P. 00071 */ 00072 void destroy(pointer p) { 00073 // destroy using "explicit destructor" 00074 p->~T(); 00075 } 00076 00077 // Boilerplate 00078 SecureAllocator() {} 00079 template<typename U> SecureAllocator(const SecureAllocator<U>&) {} 00080 template<typename U> struct rebind { using other = SecureAllocator<U>; }; 00081 }; 00082 00083 00084 template<typename T, typename U> 00085 bool operator==(const SecureAllocator<T>&, const SecureAllocator<U>&) { 00086 return true; 00087 } 00088 00089 template<typename T, typename U> 00090 bool operator!=(const SecureAllocator<T>&, const SecureAllocator<U>&) { 00091 return false; 00092 } 00093 00094 } //namespace Json 00095 00096 #pragma pack(pop) 00097 00098 #endif // CPPTL_JSON_ALLOCATOR_H_INCLUDED
Generated on Tue Jul 12 2022 21:24:54 by
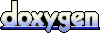