
Embed:
(wiki syntax)
Show/hide line numbers
max6675.cpp
00001 00002 #include <mbed.h> 00003 #include "max6675.h" 00004 00005 max6675::max6675(SPI& _spi, PinName _ncs) : spi(_spi), ncs(_ncs) { 00006 00007 } 00008 00009 float max6675::read_temp() { 00010 short value = 0; 00011 float temp = 0; 00012 00013 uint8_t highByte=0; 00014 uint8_t lowByte=0; 00015 00016 select(); 00017 wait(.25); //This delay is needed else it does'nt seem to update the temp 00018 00019 highByte = spi.write(0); 00020 lowByte = spi.write(0); 00021 deselect(); 00022 00023 00024 if (lowByte & (1<<2)) { 00025 error("No Probe"); 00026 } else { 00027 value = (highByte << 5 | lowByte>>3); 00028 } 00029 00030 temp = (value*0.25); // Multiply the value by 0.25 to get temp in ˚C or 00031 // * (9.0/5.0)) + 32.0; // Convert value to ˚F (ensure proper floats!) 00032 00033 return temp; 00034 } 00035 00036 void max6675::select() { 00037 ncs = 0; 00038 } 00039 00040 void max6675::deselect() { 00041 ncs = 1; 00042 }
Generated on Thu Jul 14 2022 01:35:19 by
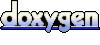