
BlackOneサンプルプログラム ”フォトフレーム” 詳細はエレキジャックのページを参照ください。 BlackOne Sample Prrogram \"PhotoFrame\"
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /************************************************************************* 00002 * 00003 * mbed BlackOne 00004 * Photo Frame Sample Program for Eleki Jack 00005 * by Techand 00006 ************************************************************************** 00007 */ 00008 00009 #include "mbed.h" 00010 Serial pc(USBTX, USBRX); // tx, rx 00011 Serial lcd(p9, p10); // lcd serial port 00012 00013 00014 // Function prototypes ************************** 00015 void set_RTC (); 00016 void calendar(); 00017 00018 00019 /*********************************** 00020 * 00021 * Photo Frame Main 00022 * 00023 ************************************ 00024 */ 00025 int main() { 00026 wait(1); 00027 lcd.printf("\x1b@14Z"); // 57600bps 00028 lcd.baud(57600); 00029 wait(1); 00030 lcd.printf("\x1b@0Z"); // Clear all data (character & graphics) 00031 lcd.printf("\x1b[>5h"); // Curser OFF 00032 lcd.printf("\x1b@30Z"); // Set buffer0 for display 00033 00034 set_RTC(); // Set and Start RTC 00035 00036 calendar(); // format and display calender 00037 00038 // Display "Photo" 00039 for (;;) 00040 { 00041 for (int i=1 ;i<=8;i++) 00042 { 00043 lcd.printf("\x1b@%d;0I",i); 00044 wait_ms(2000); 00045 } 00046 } 00047 } 00048 00049 00050 /***************************** 00051 * 00052 * Set and Init. RTC 00053 * 00054 ****************************** 00055 */ 00056 void set_RTC () { 00057 time_t seconds = time(NULL); 00058 00059 if ( (signed)seconds == -1 ) 00060 { 00061 // setup time 00062 struct tm t; 00063 00064 t.tm_sec = 00; // seconds 0-59 00065 t.tm_min = 00; // minuits 0-59 00066 t.tm_hour = 10; // hour 0-23 00067 t.tm_mday = 3; // day 1-31 00068 t.tm_mon = 2; // month 0-11 00069 t.tm_year = 112; // year from 1900 00070 00071 seconds = mktime(&t); 00072 set_time(seconds); 00073 } 00074 } 00075 00076 00077 00078 /***************************** 00079 * 00080 * format and Display Calender 00081 * 00082 ****************************** 00083 */ 00084 void calendar() 00085 { 00086 int w_today; 00087 int today; 00088 int w; 00089 00090 char buff[16]; 00091 00092 int md[12] = {31,29,31,30,31,30,31,31,30,31,30,31}; // size of month. Leap Year This Year! 00093 char sun[] = {0x93,0xFA,0x00}; // sunday 00094 char sat[] = {0x20,0x93,0x79,0x00}; // Saturday 00095 char week[] = {0x20,0x8C,0x8E,0x20,0x89,0xCE,0x20,0x90,0x85,0x20,0x96,0xD8,0x20,0x8B,0xE0,0x00}; 00096 // Week Header "sun mon tue wed thu fri sat" 00097 00098 time_t seconds = time(NULL); 00099 struct tm *t = localtime(&seconds); 00100 00101 lcd.printf("\x1b[8;0H"); 00102 lcd.printf("\x1b[1;32;m"); 00103 strftime(buff,15, "%Y %B", localtime(&seconds)); 00104 lcd.printf("%s",buff); 00105 00106 lcd.printf("\x1b[9;0H"); 00107 lcd.printf("\x1b[1;31;m"); 00108 lcd.printf("%s",sun); 00109 lcd.printf("\x1b[1;32;m"); 00110 lcd.printf("%s",week); 00111 lcd.printf("\x1b[1;34;m"); 00112 lcd.printf("%s",sat); 00113 00114 today = t->tm_mday; 00115 w_today = t->tm_wday; 00116 00117 w=0; 00118 w_today = (w_today - ((today % 7)-1)); 00119 00120 for ( int i = 1; i <= md[t->tm_mon]; i++) 00121 { 00122 lcd.printf("\x1b[%d;%dH",((w_today+w)/7)+10,(3*((w_today+w)%7))+0); 00123 00124 lcd.printf("\x1b[1;32;m"); 00125 00126 if ( (w_today+w)%7 == 0) 00127 { 00128 lcd.printf("\x1b[1;31;m"); 00129 } 00130 if ( (w_today+w)%7 == 6) 00131 { 00132 lcd.printf("\x1b[1;34;m"); 00133 } 00134 00135 if ( i == today) 00136 { 00137 lcd.printf("\x1b[7m"); 00138 } else 00139 { 00140 lcd.printf("\x1b[27m"); 00141 } 00142 00143 lcd.printf("%2d",i); 00144 w++; 00145 } 00146 }
Generated on Mon Jul 18 2022 12:38:23 by
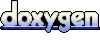