Music Engine plays Music Macro Language compositions in the background
Dependents: RETRO_BallsAndPaddle RETRO_BallAndHoles MusicBoxForFathersDay USBSec_mbed-os_dev
MusicEngine.h
00001 /////////////////////////////////////////////////////////////////////////////// 00002 // Retro Music Engine 00003 // Author: Chris Taylor (taylorza) 00004 00005 #include "mbed.h" 00006 00007 #ifndef __MUSICENGINE_H__ 00008 #define __MUSICENGINE_H__ 00009 00010 /** MusicEngine provides a means to play Music Macro Language sequences asynchronously. 00011 * Learn more about Music Macro Language (MML) on wikipedia 00012 * http://en.wikipedia.org/wiki/Music_Macro_Language 00013 */ 00014 class MusicEngine 00015 { 00016 public: 00017 /** Creates an instance of the MusicEngine 00018 * @param pin pin used to generate the note frequencies 00019 */ 00020 MusicEngine(PinName pin); 00021 00022 /** Starts playing a new MML sequence. If one is already playing it is stopped and the new sequences started. 00023 * @param mml string of MML commands to be played 00024 */ 00025 void play(char *mml); 00026 00027 /** Stop a currently playing sequence */ 00028 void stop(); 00029 00030 /** Query the engine to determine if a MML sequence is currently being played. */ 00031 bool getIsPlaying() { return _isPlaying; } 00032 00033 /** Setup a callback function that will be executed when the music sequence ends. */ 00034 void setCompletionCallback(void (*function)(void)) 00035 { 00036 _completionCallback.attach(function); 00037 } 00038 00039 /** Setup a callback function that will be executed when the music sequence ends. 00040 * @note This override is used if the callback is a class member 00041 */ 00042 template<typename T> 00043 void setCompletionCallback(T *object, void (T::*member)(void)) 00044 { 00045 _completionCallback.attach(object, member); 00046 } 00047 00048 private: 00049 void executeCommand(); 00050 void skipWhiteSpace(); 00051 char getChar(); 00052 char peekChar(); 00053 void rewind(); 00054 int getNumber(int min, int max); 00055 00056 private: 00057 PwmOut _pwm; 00058 bool _isPlaying; 00059 char *_mml; 00060 int _mmlIndex; 00061 int _octave; 00062 float _duration; 00063 float _durationRatio; 00064 float _pause; 00065 int _tempo; 00066 Timeout _scheduler; 00067 00068 FunctionPointer _completionCallback; 00069 00070 static const float PERIOD_TABLE[]; 00071 00072 static const float WHOLE_NOTE_DURATION; 00073 static const float QUARTER_NOTE_DURATION; 00074 static const float QUARTER_NOTES_PER_MINUTE; 00075 00076 static const float DEFAULT_TIMING; 00077 static const float LEGATO_TIMING; 00078 static const float STACCATO_TIMING; 00079 00080 static const int NOTE_REST; 00081 static const int NOTE_C; 00082 static const int NOTE_CS; 00083 static const int NOTE_D; 00084 static const int NOTE_DS; 00085 static const int NOTE_E; 00086 static const int NOTE_F; 00087 static const int NOTE_FS; 00088 static const int NOTE_G; 00089 static const int NOTE_GS; 00090 static const int NOTE_A; 00091 static const int NOTE_AS; 00092 static const int NOTE_B; 00093 }; 00094 #endif //__MUSICENGINE_H__
Generated on Wed Jul 13 2022 21:20:31 by
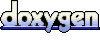