
Picotcp memory usage benchmark (still beta testing)
Dependencies: PicoTCP lpc1768-picotcp-eth mbed-rtos mbed
main.cpp
00001 /* 00002 * To enable stack measurement go to pico_mbed.h and add 00003 * #define PICO_MEASURE_STACK 00004 * to read the current number of free stack words (integers) call stack_get_free_words 00005 * 00006 * To enable memory measurement go to pico_mbed.h and add 00007 * #define MEMORY_MEASURE 00008 */ 00009 00010 #include <mbed.h> 00011 #include <stdarg.h> 00012 #include "EthernetInterface.h" 00013 00014 extern "C" 00015 { 00016 #include "pico_mbed.h" 00017 #ifdef MEMORY_MEASURE 00018 uint32_t max_mem; 00019 uint32_t cur_mem; 00020 #endif 00021 } 00022 00023 #define ECHO_SERVER_PORT 7 00024 #define BUFFER_QUANTITY (1024*1024) 00025 00026 //#define printf(...) do{}while(0) 00027 00028 void printMemoryStats(void) 00029 { 00030 #ifdef PICO_MEASURE_STACK 00031 printf("******** Stack Statistics\n"); 00032 printf("Current free stack : %d bytes\n",stack_get_free_words()*sizeof(int)); 00033 printf("Total stack size : %d bytes\n",STACK_TOTAL_WORDS*sizeof(int)); 00034 printf("Used : %.2f %%\n",100.0*(float)(STACK_TOTAL_WORDS-stack_get_free_words())/STACK_TOTAL_WORDS); 00035 #endif 00036 00037 #ifdef MEMORY_MEASURE 00038 printf("******** Memory Statistics\n"); 00039 printf("Current used memory : %d bytes\n",cur_mem); 00040 printf("Maximum used memory : %d bytes\n",max_mem); 00041 #endif 00042 } 00043 00044 int main() { 00045 00046 printf("Ethernet Interface memory test....\n"); 00047 00048 EthernetInterface eth; 00049 int connections = 0; 00050 eth.init(); 00051 printf("Waiting for DHCP to give IP\n"); 00052 while(eth.connect() != 0); 00053 printf("IP Address %s\n", eth.getIPAddress()); 00054 00055 TCPSocketServer server; 00056 server.bind(ECHO_SERVER_PORT); 00057 server.listen(); 00058 00059 while (true) { 00060 printf("\nWait for new connection...\n"); 00061 printf("Client number %d\n",++connections); 00062 TCPSocketConnection client; 00063 server.accept(client); 00064 client.set_blocking(false, 1500); // Timeout after (1.5)s 00065 printf("Connection from: %s\n", client.get_address()); 00066 char buffer[1024]; 00067 while (true) { 00068 00069 int dataReceived = 0; 00070 int dataSent = 0; 00071 00072 while(dataReceived < BUFFER_QUANTITY) 00073 { 00074 int n = client.receive(buffer, sizeof(buffer)); 00075 if (n <= 0) { 00076 printf("Receive error\n"); 00077 break; 00078 } 00079 dataReceived += n; 00080 } 00081 00082 if(dataReceived < BUFFER_QUANTITY) 00083 { 00084 printf("Receiving part of the test has failed. Exiting connection.\n"); 00085 printf("Received : %d bytes\nExpected : %d bytes\n",dataReceived,BUFFER_QUANTITY); 00086 break; 00087 } 00088 00089 while(dataSent < BUFFER_QUANTITY) 00090 { 00091 int n = client.send_all(buffer, sizeof(buffer)); 00092 if (n <= 0) { 00093 printf("Send error\n"); 00094 break; 00095 } 00096 dataSent += n; 00097 } 00098 00099 if(dataSent < BUFFER_QUANTITY) 00100 { 00101 printf("Sending part of the test has failed. Exiting connection.\n"); 00102 printf("Sent : %d bytes\nExpected : %d bytes\n",dataSent,BUFFER_QUANTITY); 00103 00104 break; 00105 } 00106 00107 dataReceived = dataSent = 0; 00108 while((dataReceived+dataSent) < 2*BUFFER_QUANTITY) 00109 { 00110 int n = client.receive(buffer, sizeof(buffer)); 00111 if (n <= 0) { 00112 printf("Receive error\n"); 00113 break; 00114 } 00115 dataReceived += n; 00116 00117 n = client.send_all(buffer, n); 00118 if (n <= 0) { 00119 printf("Send error\n"); 00120 break; 00121 } 00122 dataSent += n; 00123 } 00124 00125 if((dataReceived+dataSent) < 2*BUFFER_QUANTITY) 00126 { 00127 printf("Echo size : %d bytes\nExpected : %d bytes\n",(dataReceived+dataSent),2*BUFFER_QUANTITY); 00128 printf("Echo test has failed.Exiting connection...\n"); 00129 } 00130 00131 break; 00132 } 00133 00134 client.close(); 00135 printf("Test was finished...\n"); 00136 printMemoryStats(); 00137 } 00138 00139 return 0; 00140 }
Generated on Mon Jul 18 2022 16:22:21 by
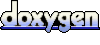