
TCP Official Tx Benchmark
Dependencies: PicoTCP lpc1768-picotcp-eth mbed-rtos mbed
main.cpp
00001 #include <mbed.h> 00002 #include <stdarg.h> 00003 #include "EthernetInterface.h" 00004 00005 #define ECHO_SERVER_PORT 7 00006 #define BUFFER_QUANTITY (1024*1024) 00007 #define BUFFER_SIZE (1024) 00008 00009 00010 EthernetInterface eth; 00011 00012 int main() 00013 { 00014 TCPSocketServer server; 00015 00016 printf("Started PicoTCP Tx Benchmark....\n"); 00017 00018 eth.init(); 00019 printf("Waiting for DHCP server to give IP...\n"); 00020 00021 while(eth.connect() != 0); 00022 printf("IP address assigned : %s\n",eth.getIPAddress()); 00023 00024 server.bind(ECHO_SERVER_PORT); 00025 server.listen(); 00026 printf("Local server listening on port : %d\n",ECHO_SERVER_PORT); 00027 00028 00029 while (true) { 00030 printf("\n>>> You can start PicoTCP_Official_TCP_Tx_Benchmark.py\n"); 00031 00032 TCPSocketConnection client; 00033 server.accept(client); 00034 client.set_blocking(false, 1500); // Timeout after (1.5)s 00035 00036 char buffer[BUFFER_SIZE]; 00037 int dataSent = 0; 00038 while(dataSent < BUFFER_QUANTITY) 00039 { 00040 int n = client.send_all(buffer, sizeof(buffer)); 00041 if (n <= 0) { 00042 break; 00043 } 00044 dataSent += n; 00045 } 00046 00047 if(dataSent == BUFFER_QUANTITY) 00048 { 00049 printf("You can read the Tx Throughput now \n"); 00050 } 00051 else 00052 { 00053 printf("Benchmark failed !\n"); 00054 } 00055 } 00056 }
Generated on Sun Jul 17 2022 01:06:36 by
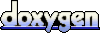