
Internet of Greens. Mini automated greenhouse, with an internet of sensors.
Dependencies: HC_SR04_Ultrasonic_Library PixelArrayBuffer Servo TSL2561 mbed-rtos mbed
Fork of Final_project by
main.cpp
00001 #include "mbed.h" 00002 #include "th02.h" 00003 #include "TSL2561.h" 00004 #include "ultrasonic.h" 00005 #include "Servo.h" 00006 #include "neopixel.h" 00007 #include "stdlib.h" 00008 #include "rtos.h" 00009 #include "support.h" 00010 00011 using namespace std; 00012 00013 DigitalOut temp_alert(p21); 00014 float tankHeight = 9.5f; //INITIALIZE!!! 00015 00016 //Thread t1; 00017 //Thread t2; 00018 00019 void dist(int distance){ //save plant height in inches! 00020 //prev_height = plant_height; 00021 plant_height =(distance*0.0393701); 00022 } 00023 ultrasonic mu(p6, p7, .1, 1, &dist); 00024 00025 00026 void Irrigation(void const *args) { 00027 while(1){ 00028 moisture_level = moisture; 00029 if (!watering && moisture_level > 0.3f) 00030 water_plant(); 00031 Thread::wait(86400000); //SET CORRECT INTERVAL 00032 } 00033 } 00034 00035 void Status(void const *args) { 00036 while(1){ 00037 read_temp(); 00038 read_humidity(); 00039 mu.checkDistance(); 00040 lux = sensor.lux(); 00041 moisture_level = moisture; 00042 00043 if (temperature < 15.0f || temperature > 30.0f) 00044 temp_alert = 1; 00045 else 00046 temp_alert = 0; 00047 00048 if (moisture_level > 0.8f) 00049 water_plant(); 00050 00051 if (lux < 5.0f){ 00052 lights_on = true; 00053 switch_lights(); 00054 } else { 00055 lights_on = false; 00056 switch_lights(); 00057 } 00058 00059 Thread::wait(2000); //SET CORRECT INTERVAL 00060 } 00061 } 00062 00063 00064 int main() { 00065 char c=0; 00066 pi.baud(9600); 00067 mu.startUpdates(); 00068 moisture_level = moisture; 00069 00070 //t1.start(Irrigation); 00071 //t2.start(Status); 00072 Thread t1(Irrigation); 00073 Thread t2(Status); 00074 00075 while(1) { 00076 c = pi.getc(); 00077 if (c != 0) { 00078 switch(c){ 00079 case '1': 00080 { 00081 lights_on = true; 00082 switch_lights(); 00083 break; 00084 } 00085 case '0': 00086 { 00087 lights_on = false; 00088 switch_lights(); 00089 break; 00090 } 00091 case '2': 00092 { 00093 water_plant(); 00094 break; 00095 } 00096 case '3': 00097 { 00098 //std::string s = patch::to_string((float)moisture_level); 00099 std::string s = patch::to_string((float)sensor.lux()); 00100 pi.printf("%s",s); 00101 break; 00102 } 00103 case '4': 00104 { 00105 read_temp(); 00106 std::string s = patch::to_string((float)temperature); 00107 pi.printf("%s",s); 00108 break; 00109 } 00110 case '5': 00111 { 00112 read_humidity(); 00113 std::string s = patch::to_string((float)humidity); 00114 pi.printf("%s",s); 00115 break; 00116 } 00117 case '6': 00118 { 00119 mu.checkDistance(); 00120 std::string s = patch::to_string((float)plant_height); 00121 pi.printf("%s",s); 00122 break; 00123 } 00124 default: 00125 break; 00126 00127 } 00128 pi.putc(0); 00129 } 00130 wait(1); 00131 } 00132 00133 return 0; 00134 } 00135 00136 00137 00138 00139 00140 00141 00142 00143 00144 00145 00146 00147 00148
Generated on Mon Jul 18 2022 05:50:58 by
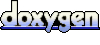