
kintone REST API sample
Dependencies: C12832 HTTPClient LM75B SNICInterface mbed-rtos mbed
main.cpp
00001 #define POST_URL "https://{subdomain}.cybozu.com/k/v1/record.json" 00002 #define APP_ID 999 00003 #define API_TOKEN "xxx" 00004 #define BASIC64 "xxx" 00005 #define DEMO_AP_SSID "xxx" 00006 #define DEMO_AP_SECUTIRY_KEY "xxx" 00007 #define INTERVAL 60 00008 00009 #include "mbed.h" 00010 #include "LM75B.h" 00011 #include "C12832.h" 00012 #include "HTTPClient.h" 00013 #include "SNIC_WifiInterface.h" 00014 00015 C12832 lcd(p5, p7, p6, p8, p11); 00016 LM75B sensor(p28, p27); 00017 00018 #if defined(TARGET_LPC1768) 00019 C_SNIC_WifiInterface wifi( p9, p10, NC, NC, p30 ); 00020 #elif defined(TARGET_NUCLEO_F401RE) || defined(TARGET_NUCLEO_L152RE) 00021 C_SNIC_WifiInterface wifi( D8, D2, NC, NC, D3); 00022 #elif defined(TARGET_K64F) 00023 C_SNIC_WifiInterface wifi( D1, D0, NC, NC, D2); 00024 #endif 00025 #define DEMO_AP_SECURITY_TYPE e_SEC_WPA2_AES 00026 00027 00028 00029 void display(char *message) 00030 { 00031 lcd.cls(); 00032 lcd.locate(0,3); 00033 lcd.printf("%s", message); 00034 wait(0.1); 00035 } 00036 00037 void post(void) 00038 { 00039 HTTPClient http; 00040 HTTPResult ret; 00041 char str[512]; 00042 char message[128]; 00043 00044 HTTPText inText(str, 1024); 00045 00046 char post_template[] = "{\"app\": %i, \"record\": {\"temp\": {\"value\": \"%.1f\"}}}\n"; 00047 char header_template[] = "X-Cybozu-API-Token: %s\nContent-Type: application/json\n"; 00048 char header_template_basic[] = "Authorization:Basic %s\nX-Cybozu-API-Token: %s\nContent-Type: application/json\n"; 00049 char post_data[256]; 00050 char header_data[256]; 00051 00052 // Basic Authentications 00053 if (BASIC64 != "") { 00054 sprintf(header_data, header_template_basic, BASIC64, API_TOKEN); 00055 } else { 00056 sprintf(header_data, header_template, API_TOKEN); 00057 } 00058 sprintf(post_data, post_template, APP_ID, (float)sensor); 00059 00060 HTTPText outText(post_data, strlen(post_data)); 00061 00062 http.setHeader(header_data) ; 00063 http.setSSLversion(3) ; /* TLSv1.2 */ 00064 ret = http.post(POST_URL, outText, &inText); 00065 if (ret == HTTP_OK) { 00066 sprintf(message, "OK: %s", str); 00067 display(message); 00068 } else { 00069 sprintf(message, "ERROR: %d", http.getHTTPResponseCode()); 00070 display(message); 00071 } 00072 } 00073 00074 int main() 00075 { 00076 int check = 0; 00077 char message[128]; 00078 00079 // Initialize Wi-Fi interface 00080 check = wifi.init(); 00081 if (check != 0) { 00082 display("Wifi could not be initialized"); 00083 return -1; 00084 } else { 00085 display("wifi initialized successfully"); 00086 } 00087 wait(0.5); 00088 00089 // good form to make sure you are disconnected from all AP's 00090 check = wifi.disconnect(); 00091 if (check != 0) { 00092 display("disconnect failed"); 00093 return -1; 00094 } else { 00095 display("disconnection successful"); 00096 } 00097 wait(0.3); 00098 00099 // Connect AP 00100 wifi.connect( DEMO_AP_SSID 00101 , strlen(DEMO_AP_SSID) 00102 , DEMO_AP_SECURITY_TYPE 00103 , DEMO_AP_SECUTIRY_KEY 00104 , strlen(DEMO_AP_SECUTIRY_KEY) ); 00105 wait(0.5); 00106 00107 // Get DHCP IP 00108 check = wifi.setIPConfig(true); 00109 if (check != 0) { 00110 display("SetIPConfig failed"); 00111 return -1; 00112 } else { 00113 display("SetIPConfig successful"); 00114 } 00115 sprintf(message, "IP: %s", wifi.getIPAddress()); 00116 display(message); 00117 00118 while (true) { 00119 post(); 00120 wait(INTERVAL); 00121 } 00122 00123 wifi.disconnect(); 00124 }
Generated on Sun Jul 17 2022 14:51:21 by
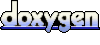