
Dead by Daylight
Embed:
(wiki syntax)
Show/hide line numbers
Motor.h
00001 #ifndef MOTOR_H_ 00002 #define MOTOR_H_ 00003 00004 #include <stdint.h> 00005 00006 //#define USE_MOTOR0_SENSOR 00007 namespace MOTOR 00008 { 00009 #define FREE 0 00010 #define BACK 1 00011 #define FOR 2 00012 #define BRAKE 3 00013 00014 #define MOUNTING_MOTOR_NUM 5 00015 00016 #define MOTOR0_D1 directions[0] 00017 #define MOTOR0_D2 directions[1] 00018 #define MOTOR1_D1 directions[2] 00019 #define MOTOR1_D2 directions[3] 00020 #define MOTOR2_D1 directions[4] 00021 #define MOTOR2_D2 directions[5] 00022 #define MOTOR3_D1 directions[6] 00023 #define MOTOR3_D2 directions[7] 00024 #define MOTOR4_D1 directions[8] 00025 #define MOTOR4_D2 directions[9] 00026 00027 #define MOTOR0_D1_PIN D3 00028 #define MOTOR0_D2_PIN D4 00029 #define MOTOR1_D1_PIN D5 00030 #define MOTOR1_D2_PIN D6 00031 #define MOTOR2_D1_PIN D8 00032 #define MOTOR2_D2_PIN D9 00033 #define MOTOR3_D1_PIN D12 00034 #define MOTOR3_D2_PIN A3 00035 #define MOTOR4_D1_PIN A1 00036 #define MOTOR4_D2_PIN A0 00037 00038 #define MOTOR0_PWM_PIN D2 00039 #define MOTOR1_PWM_PIN D7 00040 #define MOTOR2_PWM_PIN D10 00041 #define MOTOR3_PWM_PIN D11 00042 #define MOTOR4_PWM_PIN A2 00043 00044 #ifdef USE_MOTOR0_SENSOR 00045 #define MOTOR_START_NUM 1 00046 #else 00047 #define MOTOR_START_NUM 0 00048 #endif 00049 00050 typedef struct 00051 { 00052 union 00053 { 00054 struct 00055 { 00056 unsigned int d2 : 1; 00057 unsigned int d1 : 1; 00058 unsigned int : 6; 00059 }; 00060 uint8_t dir; 00061 }; 00062 float pwm; 00063 }MotorStatus; 00064 00065 class Motor 00066 { 00067 public: 00068 static void Initialize(void); 00069 static void Update(MotorStatus *status); 00070 static void SetDefault(void); 00071 static int SetStatus(float pwm); 00072 }; 00073 } 00074 00075 #endif
Generated on Thu Jul 28 2022 23:31:47 by
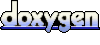