Semaphore based wait and sync for the mp3 play
Dependents: RTOS-VS1053b-mp3_semaphore
VS1053t.cpp
00001 /* mbed VLSI VS1053t library 00002 * 00003 * Thread based VS1053 class inherited by VS1053b 00004 * 00005 */ 00006 00007 00008 #include "mbed.h" 00009 #include "rtos.h" 00010 00011 #include "defines.h" 00012 #include "VS1053t.h" 00013 00014 VS1053t :: VS1053t( 00015 PinName mosi, PinName miso, PinName sck, 00016 PinName cs, PinName rst, PinName dreq, PinName dcs, 00017 char* buffer, int buffer_size, bool thread 00018 ): 00019 00020 VS1053( mosi, miso, sck, cs, rst, dreq, dcs, 00021 buffer, buffer_size) 00022 { } 00023 00024 Semaphore lock_buff(1) ; 00025 00026 unsigned int VS1053t::waitBuffer(unsigned int size, uint32_t time) { 00027 int n ; 00028 WaitingSize = size ; 00029 while ((n = VS1053t::bufferFree()) < size) { 00030 if(thread) 00031 lock_buff.wait(time) ; 00032 else 00033 wait_ms(100) ; 00034 } 00035 // if(n < size) { time out 00036 return(n) ; 00037 } 00038 00039 unsigned char VS1053t::bufferGetByte(void) { 00040 unsigned char retVal = 0x00; 00041 if (bufferCount() > 0x00) { 00042 retVal = *_bufferReadPointer++; 00043 if (_bufferReadPointer >= _buffer + BUFFER_SIZE) { 00044 _bufferReadPointer = _buffer; 00045 } 00046 } 00047 if (thread && (VS1053t::bufferFree() >= WaitingSize)) 00048 lock_buff.release() ; 00049 return retVal; 00050 }
Generated on Fri Jul 29 2022 13:01:45 by
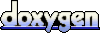