
Pachube feed API/v2 with json, temp/humid with DHT22
Dependencies: NetServices mbed DHT22
main.cpp
00001 // includes 00002 00003 #include "mbed.h" 00004 #include "EthernetNetIf.h" 00005 #include "HTTPClient.h" 00006 #include "DHT22.h" 00007 00008 EthernetNetIf eth; 00009 HTTPClient http; 00010 00011 // feed with 2 streams 00012 char contentTemplate[] = "{ \ 00013 \"title\":\"TITLE HERE\", \"version\":\"1.0.0\",\ 00014 \"datastreams\":[\ 00015 { \"id\":\"STREAM ID\", \"current_value\":\"%d\"},\ 00016 { \"id\":\"STREAM ID\", \"current_value\":\"%d\"}\ 00017 ]\ 00018 }" ; 00019 00020 00021 char content[200] ; 00022 00023 DHT22 dht22(p15) ; 00024 00025 int main() { 00026 00027 HTTPText txt; 00028 int r ; 00029 00030 printf("Start\n"); 00031 printf("\r\nSetting up...\r\n"); 00032 EthernetErr ethErr = eth.setup(); 00033 if (ethErr) { 00034 printf("Error %d in setup.\n", ethErr); 00035 return -1; 00036 } 00037 printf("\r\nSetup OK\r\n"); 00038 00039 // copy API key from settings 00040 string apiKey = "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"; 00041 00042 // use feed ID 00043 string environmentID = "xxxxx"; 00044 00045 // for authentication, API key is set in client header 00046 HTTPClient client; 00047 client.setRequestHeader("X-PachubeApiKey", apiKey); 00048 00049 // text object holds data to be posted 00050 HTTPText jsonContent("text/json"); 00051 00052 while (1) { 00053 dht22.sample() ; 00054 sprintf(content, contentTemplate, dht22.getHumidity()/10.0, dht22.getTemperature()/10.0) ; 00055 printf("Json: %s\n", content) ; 00056 jsonContent.set(content) ; 00057 00058 // uri for post includes feed ID 00059 string uri = "http://api.pachube.com/v2/feeds/" + environmentID + ".json?_method=put"; 00060 00061 // result should be 0 and response should be 200 for successful post 00062 HTTPResult result = client.post(uri.c_str(), jsonContent, NULL); 00063 00064 if (result==HTTP_OK) { 00065 printf("Result :\"%s\"\n", txt.gets()); 00066 } else { 00067 printf("Error %d\n", result); 00068 } 00069 00070 00071 r = client.getHTTPResponseCode(); 00072 if (result==HTTP_OK) { 00073 printf("Result :\"%d\"\n", r); 00074 } else { 00075 printf("Error %d\n", r); 00076 } 00077 00078 wait(60) ; 00079 } 00080 }
Generated on Wed Jul 13 2022 16:20:15 by
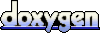