Simple HTTP Client Example with HTTPClient class
Dependencies: HTTPClient mbed-rtos mbed-src
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "HTTPClient.h" 00004 00005 #define HOST "192.168.1.12" 00006 00007 static HTTPClient http; 00008 static char str[1024*20]; 00009 00010 void main_body(void const *av) { 00011 00012 char url[100] ; 00013 int ret ; 00014 //GET data 00015 00016 printf("\nTrying to fetch page...\n"); 00017 sprintf(url, "http://%s/", HOST) ; 00018 printf("url=%s\n",url) ; 00019 ret = http.get(url, str, sizeof(str)-1,16); 00020 if (!ret) { 00021 printf("Page fetched successfully - read %d characters\n", strlen(str)); 00022 printf("Result: %s\n", str); 00023 } else { 00024 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00025 } 00026 00027 } 00028 00029 static EthernetInterface eth; 00030 00031 int main() 00032 { 00033 int ret ; 00034 void *av ; 00035 printf("HTTP Client Starting,...\n") ; 00036 ret = eth.init(); //Use DHCP 00037 while(1) { 00038 ret = eth.connect(); 00039 if(ret == 0)break ; 00040 } 00041 printf("IP = %s\n", eth.getIPAddress()); 00042 00043 main_body(av) ; 00044 00045 eth.disconnect(); 00046 while(1) { 00047 } 00048 00049 }
Generated on Thu Jul 28 2022 15:41:23 by
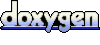