Lightning sensor library It is inherited from https://developer.mbed.org/users/casper/code/AS3935/
Dependents: BLE_Lightning_sensor
AS3935_ext.cpp
00001 /* 00002 AS3935_ext.cpp - AS3935 Franklin Lightning Sensor™ IC by AMS library 00003 Copyright (c) 2015 T.Naka. All rights reserved. 00004 00005 This library is free software; you can redistribute it and/or 00006 modify it under the terms of the GNU Lesser General Public 00007 License as published by the Free Software Foundation; either 00008 version 3 of the License, or (at your option) any later version. 00009 00010 This library is distributed in the hope that it will be useful, 00011 but WITHOUT ANY WARRANTY; without even the implied warranty of 00012 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00013 Lesser General Public License for more details. 00014 00015 You should have received a copy of the GNU Lesser General Public 00016 License along with this library; if not, write to the Free Software 00017 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00018 */ 00019 00020 #include "AS3935_ext.h" 00021 00022 AS3935_ext::AS3935_ext(PinName sda, PinName scl, int adresse, PinName irqin): AS3935(sda, scl ,adresse) 00023 { 00024 irq = irqin; 00025 wait_ms(11); 00026 } 00027 00028 void AS3935_ext::reset() 00029 { 00030 00031 AS3935::reset(); 00032 00033 // Callibrate automatically the internal RCO Oscillators 00034 registerWrite(0x3D,0xff,0x96); 00035 wait_ms(2); 00036 00037 // Minimum number of lightning 00038 registerWrite(AS3935_MIN_NUM_LIGH ,1); 00039 00040 } 00041 00042 int AS3935_ext::MeasureLCOFreq() 00043 { 00044 DigitalIn display(irq); 00045 int t0; 00046 int t1; 00047 float hz; 00048 unsigned int ticks_per_second; 00049 // int d; 00050 #define LFCLK_FREQUENCY 0x8000 00051 #define RTC_COUNTER_SIZE 0x1000000 00052 ticks_per_second = LFCLK_FREQUENCY / (NRF_RTC1->PRESCALER + 1); 00053 00054 // Set LCO_FDIV as 32 (0x3) (multiply 128) 00055 wait_ms(20); 00056 registerWrite(AS3935_LCO_FDIV,0x3); 00057 00058 // Enable display LCO on IRQ 00059 wait_ms(20); 00060 registerWrite(AS3935_DISP_LCO,1); 00061 00062 wait_ms(100); 00063 00064 // Wait until IRQ becomes low; 00065 while(display.read() == 1) 00066 00067 // Wait until IRQ becomes high 00068 wait_ms(20); 00069 while(display.read() == 0); 00070 t0 = NRF_RTC1->COUNTER; 00071 00072 for(int i=0;i<1000;i++) { 00073 // Wait until IRQ becomes low 00074 while(display.read() == 1); 00075 00076 // Wait until IRQ becomes high; 00077 while(display.read() == 0); 00078 } 00079 t1 = NRF_RTC1->COUNTER; 00080 // printf("%d\r\n",t1-t0); 00081 00082 // 1000*128/500k = 0.256sec 00083 hz = 1000* 128 * ticks_per_second / (unsigned int)((t1 - t0 + RTC_COUNTER_SIZE) % RTC_COUNTER_SIZE); 00084 00085 // Disable display LCO on IRQ pin; 00086 registerWrite(AS3935_DISP_LCO,0); 00087 00088 return(hz); 00089 }
Generated on Tue Jul 12 2022 15:22:13 by
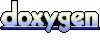