Development mbed library for MAX32630FTHR
Dependents: blinky_max32630fthr
api.py
00001 """Tests for the toolchain sub-system""" 00002 import sys 00003 import os 00004 from string import printable 00005 from copy import deepcopy 00006 from mock import MagicMock, patch 00007 from hypothesis import given 00008 from hypothesis.strategies import text, lists, fixed_dictionaries 00009 00010 ROOT = os.path.abspath(os.path.join(os.path.dirname(__file__), "..", "..", 00011 "..")) 00012 sys.path.insert(0, ROOT) 00013 00014 from tools.toolchains import TOOLCHAIN_CLASSES, LEGACY_TOOLCHAIN_NAMES,\ 00015 Resources 00016 from tools.targets import TARGET_MAP 00017 00018 def test_instantiation (): 00019 """Test that all exported toolchain may be instantiated""" 00020 for name, tc_class in TOOLCHAIN_CLASSES.items(): 00021 cls = tc_class(TARGET_MAP["K64F"]) 00022 assert name == cls.name or\ 00023 name == LEGACY_TOOLCHAIN_NAMES[cls.name] 00024 00025 ALPHABET = [char for char in printable if char not in [u'.', u'/']] 00026 00027 @given(fixed_dictionaries({ 00028 'common': lists(text()), 00029 'c': lists(text()), 00030 'cxx': lists(text()), 00031 'asm': lists(text()), 00032 'ld': lists(text())}), 00033 lists(text(min_size=1, alphabet=ALPHABET), min_size=1)) 00034 def test_toolchain_profile_c (profile, source_file): 00035 """Test that the appropriate profile parameters are passed to the 00036 C compiler""" 00037 filename = deepcopy(source_file) 00038 filename[-1] += ".c" 00039 to_compile = os.path.join(*filename) 00040 with patch('os.mkdir') as _mkdir: 00041 for _, tc_class in TOOLCHAIN_CLASSES.items(): 00042 toolchain = tc_class(TARGET_MAP["K64F"], build_profile=profile) 00043 toolchain.inc_md5 = "" 00044 toolchain.build_dir = "" 00045 compile_command = toolchain.compile_command(to_compile, 00046 to_compile + ".o", []) 00047 for parameter in profile['c'] + profile['common']: 00048 assert any(parameter in cmd for cmd in compile_command), \ 00049 "Toolchain %s did not propigate arg %s" % (toolchain.name, 00050 parameter) 00051 00052 @given(fixed_dictionaries({ 00053 'common': lists(text()), 00054 'c': lists(text()), 00055 'cxx': lists(text()), 00056 'asm': lists(text()), 00057 'ld': lists(text())}), 00058 lists(text(min_size=1, alphabet=ALPHABET), min_size=1)) 00059 def test_toolchain_profile_cpp (profile, source_file): 00060 """Test that the appropriate profile parameters are passed to the 00061 C++ compiler""" 00062 filename = deepcopy(source_file) 00063 filename[-1] += ".cpp" 00064 to_compile = os.path.join(*filename) 00065 with patch('os.mkdir') as _mkdir: 00066 for _, tc_class in TOOLCHAIN_CLASSES.items(): 00067 toolchain = tc_class(TARGET_MAP["K64F"], build_profile=profile) 00068 toolchain.inc_md5 = "" 00069 toolchain.build_dir = "" 00070 compile_command = toolchain.compile_command(to_compile, 00071 to_compile + ".o", []) 00072 for parameter in profile['cxx'] + profile['common']: 00073 assert any(parameter in cmd for cmd in compile_command), \ 00074 "Toolchain %s did not propigate arg %s" % (toolchain.name, 00075 parameter) 00076 00077 @given(fixed_dictionaries({ 00078 'common': lists(text()), 00079 'c': lists(text()), 00080 'cxx': lists(text()), 00081 'asm': lists(text()), 00082 'ld': lists(text())}), 00083 lists(text(min_size=1, alphabet=ALPHABET), min_size=1)) 00084 def test_toolchain_profile_asm (profile, source_file): 00085 """Test that the appropriate profile parameters are passed to the 00086 Assembler""" 00087 filename = deepcopy(source_file) 00088 filename[-1] += ".s" 00089 to_compile = os.path.join(*filename) 00090 with patch('os.mkdir') as _mkdir: 00091 for _, tc_class in TOOLCHAIN_CLASSES.items(): 00092 toolchain = tc_class(TARGET_MAP["K64F"], build_profile=profile) 00093 toolchain.inc_md5 = "" 00094 toolchain.build_dir = "" 00095 compile_command = toolchain.compile_command(to_compile, 00096 to_compile + ".o", []) 00097 if not compile_command: 00098 assert compile_command, to_compile 00099 for parameter in profile['asm']: 00100 assert any(parameter in cmd for cmd in compile_command), \ 00101 "Toolchain %s did not propigate arg %s" % (toolchain.name, 00102 parameter) 00103 00104 for name, Class in TOOLCHAIN_CLASSES.items(): 00105 CLS = Class(TARGET_MAP["K64F"]) 00106 assert name == CLS.name or name == LEGACY_TOOLCHAIN_NAMES[CLS.name] 00107 00108 00109 @given(lists(text(alphabet=ALPHABET, min_size=1), min_size=1)) 00110 def test_detect_duplicates(filenames): 00111 c_sources = [os.path.join(name, "dupe.c") for name in filenames] 00112 s_sources = [os.path.join(name, "dupe.s") for name in filenames] 00113 cpp_sources = [os.path.join(name, "dupe.cpp") for name in filenames] 00114 with MagicMock() as notify: 00115 toolchain = TOOLCHAIN_CLASSES["ARM"](TARGET_MAP["K64F"], notify=notify) 00116 res = Resources() 00117 res.c_sources = c_sources 00118 res.s_sources = s_sources 00119 res.cpp_sources = cpp_sources 00120 assert res.detect_duplicates(toolchain) == 1,\ 00121 "Not Enough duplicates found" 00122 00123 _, (notification, _), _ = notify.mock_calls[1] 00124 assert "dupe.o" in notification["message"] 00125 assert "dupe.s" in notification["message"] 00126 assert "dupe.c" in notification["message"] 00127 assert "dupe.cpp" in notification["message"]
Generated on Tue Jul 12 2022 14:20:55 by
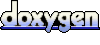